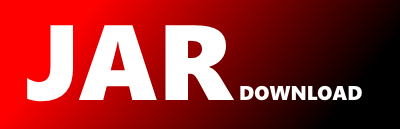
rt.pipeline.pipe.PipeResource Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rts-pipeline Show documentation
Show all versions of rts-pipeline Show documentation
Framework to build reactive services for reactive application front-ends
The newest version!
package rt.pipeline.pipe;
import com.google.common.base.Objects;
import java.util.Collection;
import java.util.HashMap;
import java.util.function.Consumer;
import org.eclipse.xtend.lib.annotations.Accessors;
import org.eclipse.xtext.xbase.lib.Procedures.Procedure0;
import org.eclipse.xtext.xbase.lib.Procedures.Procedure1;
import org.eclipse.xtext.xbase.lib.Pure;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import rt.async.pubsub.IMessageBus;
import rt.async.pubsub.IResource;
import rt.async.pubsub.ISubscription;
import rt.async.pubsub.Message;
import rt.pipeline.pipe.PipeContext;
import rt.pipeline.pipe.Pipeline;
import rt.pipeline.pipe.channel.IPipeChannel;
@SuppressWarnings("all")
public class PipeResource implements IResource {
private final static Logger logger = LoggerFactory.getLogger("RESOURCE");
@Accessors
private final String client;
@Accessors
private Procedure1 super Message> sendCallback;
@Accessors
private Procedure1 super PipeContext> contextCallback;
@Accessors
private Procedure0 closeCallback;
private final Pipeline pipeline;
private final HashMap subscriptions = new HashMap();
private final HashMap channels = new HashMap();
private final HashMap, Object> objects = new HashMap, Object>();
public IMessageBus bus() {
return this.pipeline.getMb();
}
public Object object(final Class> type, final Object instance) {
return this.objects.put(type, instance);
}
public T object(final Class type) {
Object _get = this.objects.get(type);
return ((T) _get);
}
PipeResource(final Pipeline pipeline, final String client) {
PipeResource.logger.debug("CREATE {}", client);
this.pipeline = pipeline;
this.client = client;
}
@Override
public void subscribe(final String address) {
boolean _containsKey = this.subscriptions.containsKey(address);
if (_containsKey) {
return;
}
IMessageBus _mb = this.pipeline.getMb();
final ISubscription sub = _mb.subscribe(address, this.sendCallback);
this.subscriptions.put(address, sub);
}
@Override
public void unsubscribe(final String address) {
final ISubscription listener = this.subscriptions.remove(address);
if (listener!=null) {
listener.remove();
}
}
@Override
public void disconnect() {
if (this.closeCallback!=null) {
this.closeCallback.apply();
}
}
public void process(final Message msg) {
boolean _notEquals = (!Objects.equal(this.contextCallback, null));
if (_notEquals) {
this.pipeline.process(this, msg, this.contextCallback);
} else {
this.pipeline.process(this, msg);
}
}
public void send(final Message msg) {
if (this.sendCallback!=null) {
this.sendCallback.apply(msg);
}
}
public void addChannel(final IPipeChannel channel) {
IPipeChannel.PipeChannelInfo _info = channel.getInfo();
String _uuid = _info.getUuid();
this.channels.put(_uuid, channel);
}
public IPipeChannel removeChannel(final String uuid) {
return this.channels.remove(uuid);
}
public void release() {
PipeResource.logger.debug("RELEASE {}", this.client);
Collection _values = this.subscriptions.values();
final Consumer _function = (ISubscription it) -> {
it.remove();
};
_values.forEach(_function);
this.subscriptions.clear();
Collection _values_1 = this.channels.values();
final Consumer _function_1 = (IPipeChannel it) -> {
it.close();
};
_values_1.forEach(_function_1);
this.channels.clear();
}
@Pure
public String getClient() {
return this.client;
}
@Pure
public Procedure1 super Message> getSendCallback() {
return this.sendCallback;
}
public void setSendCallback(final Procedure1 super Message> sendCallback) {
this.sendCallback = sendCallback;
}
@Pure
public Procedure1 super PipeContext> getContextCallback() {
return this.contextCallback;
}
public void setContextCallback(final Procedure1 super PipeContext> contextCallback) {
this.contextCallback = contextCallback;
}
@Pure
public Procedure0 getCloseCallback() {
return this.closeCallback;
}
public void setCloseCallback(final Procedure0 closeCallback) {
this.closeCallback = closeCallback;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy