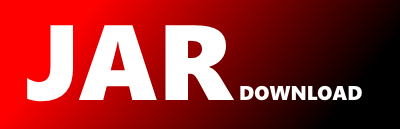
rt.pipeline.pipe.Pipeline Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rts-pipeline Show documentation
Show all versions of rts-pipeline Show documentation
Framework to build reactive services for reactive application front-ends
The newest version!
package rt.pipeline.pipe;
import com.google.common.base.Objects;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.Map;
import java.util.Set;
import org.eclipse.xtend.lib.annotations.AccessorType;
import org.eclipse.xtend.lib.annotations.Accessors;
import org.eclipse.xtext.xbase.lib.CollectionLiterals;
import org.eclipse.xtext.xbase.lib.ObjectExtensions;
import org.eclipse.xtext.xbase.lib.Pair;
import org.eclipse.xtext.xbase.lib.Procedures.Procedure1;
import org.eclipse.xtext.xbase.lib.Pure;
import rt.async.pubsub.IMessageBus;
import rt.async.pubsub.IPublisher;
import rt.async.pubsub.IResource;
import rt.async.pubsub.Message;
import rt.pipeline.DefaultMessageBus;
import rt.pipeline.IComponent;
import rt.pipeline.UserInfo;
import rt.pipeline.pipe.PipeContext;
import rt.pipeline.pipe.PipeResource;
@SuppressWarnings("all")
public class Pipeline {
@Accessors
private final IMessageBus mb;
@Accessors(AccessorType.PUBLIC_SETTER)
private Procedure1 super Throwable> failHandler = null;
private final ArrayList interceptors = new ArrayList();
private final HashMap services = new HashMap();
private final HashMap> serviceAuthorizations = new HashMap>();
private final HashSet ctxServices = new HashSet();
public Pipeline() {
this(new DefaultMessageBus());
}
public Pipeline(final IMessageBus mb) {
this.mb = mb;
}
void process(final PipeResource resource, final Message msg) {
this.process(resource, msg, null);
}
void process(final PipeResource resource, final Message msg, final Procedure1 super PipeContext> onContextCreated) {
Iterator _iterator = this.interceptors.iterator();
final PipeContext ctx = new PipeContext(this, resource, msg, _iterator);
if (onContextCreated!=null) {
onContextCreated.apply(ctx);
}
final Procedure1 _function = (PipeContext it) -> {
it.object(IPublisher.class, this.mb);
it.object(IResource.class, resource);
for (final IComponent ctxSrv : this.ctxServices) {
Class extends IComponent> _class = ctxSrv.getClass();
it.object(_class, ctxSrv);
}
it.next();
};
ObjectExtensions.operator_doubleArrow(ctx, _function);
}
public PipeResource createResource(final String client) {
return new PipeResource(this, client);
}
public void fail(final Throwable ex) {
if (this.failHandler!=null) {
this.failHandler.apply(ex);
}
}
public void addInterceptor(final IComponent interceptor) {
this.interceptors.add(interceptor);
}
public Set getComponentPaths() {
return this.services.keySet();
}
public IComponent getComponent(final String path) {
return this.services.get(path);
}
public void addComponent(final String path, final IComponent component) {
Pair _mappedTo = Pair.of("all", "all");
this.addComponent(path, component, Collections.unmodifiableMap(CollectionLiterals.newHashMap(_mappedTo)));
}
public void addComponent(final String path, final IComponent component, final Map authorizations) {
this.services.put(path, component);
this.serviceAuthorizations.put(path, authorizations);
}
public IComponent removeComponent(final String path) {
final IComponent cmp = this.services.remove(path);
this.serviceAuthorizations.remove(cmp);
return cmp;
}
public IComponent getService(final String address) {
return this.services.get(("srv:" + address));
}
public void addService(final String address, final IComponent service) {
this.addService(address, service, false);
}
public void addService(final String address, final IComponent service, final Map authorizations) {
this.addService(address, service, false, authorizations);
}
public void addService(final String address, final IComponent service, final boolean asContext) {
Pair _mappedTo = Pair.of("all", "all");
this.addService(address, service, asContext, Collections.unmodifiableMap(CollectionLiterals.newHashMap(_mappedTo)));
}
public void addService(final String address, final IComponent service, final boolean asContext, final Map authorizations) {
final String srvAddress = ("srv:" + address);
this.addComponent(srvAddress, service, authorizations);
if (asContext) {
this.ctxServices.add(service);
}
}
public void removeService(final String address) {
final String srvAddress = ("srv:" + address);
final IComponent srv = this.removeComponent(srvAddress);
this.ctxServices.remove(srv);
}
public String addAuthorization(final String path, final String cmd, final String group) {
String _xblockexpression = null;
{
Map auths = this.serviceAuthorizations.get(path);
if ((auths == null)) {
HashMap _hashMap = new HashMap();
auths = _hashMap;
this.serviceAuthorizations.put(path, auths);
}
_xblockexpression = auths.put(cmd, group);
}
return _xblockexpression;
}
public boolean isAuthorized(final Message msg, final UserInfo user) {
final Map auth = this.serviceAuthorizations.get(msg.path);
if ((auth == null)) {
return false;
}
final String allGroup = auth.get("all");
if ((allGroup != null)) {
boolean _equals = Objects.equal(allGroup, "all");
if (_equals) {
return true;
}
if ((user == null)) {
return false;
}
return user.groups.contains(allGroup);
}
final String methGroup = auth.get(msg.cmd);
if ((methGroup != null)) {
boolean _equals_1 = Objects.equal(methGroup, "all");
if (_equals_1) {
return true;
}
if ((user == null)) {
return false;
}
return user.groups.contains(methGroup);
}
return false;
}
@Pure
public IMessageBus getMb() {
return this.mb;
}
public void setFailHandler(final Procedure1 super Throwable> failHandler) {
this.failHandler = failHandler;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy