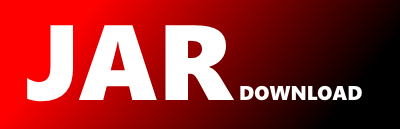
rt.pipeline.pipe.Pipeline.xtend Maven / Gradle / Ivy
package rt.pipeline.pipe
import java.util.ArrayList
import java.util.HashMap
import java.util.HashSet
import java.util.Map
import org.eclipse.xtend.lib.annotations.Accessors
import rt.async.pubsub.IMessageBus
import rt.async.pubsub.IPublisher
import rt.async.pubsub.IResource
import rt.async.pubsub.Message
import rt.pipeline.DefaultMessageBus
import rt.pipeline.IComponent
import rt.pipeline.UserInfo
class Pipeline {
@Accessors val IMessageBus mb
@Accessors(PUBLIC_SETTER) (Throwable) => void failHandler = null
val interceptors = new ArrayList
val services = new HashMap
val serviceAuthorizations = new HashMap>
val ctxServices = new HashSet
new() { this(new DefaultMessageBus) }
new(IMessageBus mb) {
this.mb = mb
}
package def void process(PipeResource resource, Message msg) {
resource.process(msg, null)
}
package def void process(PipeResource resource, Message msg, (PipeContext) => void onContextCreated) {
val ctx = new PipeContext(this, resource, msg, interceptors.iterator)
onContextCreated?.apply(ctx)
ctx => [
object(IPublisher, mb)
object(IResource, resource)
for (ctxSrv: ctxServices)
object(ctxSrv.class, ctxSrv)
next
]
}
def createResource(String client) {
return new PipeResource(this, client)
}
def fail(Throwable ex) {
failHandler?.apply(ex)
}
def void addInterceptor(IComponent interceptor) {
interceptors.add(interceptor)
}
def getComponentPaths() {
return services.keySet
}
def getComponent(String path) {
return services.get(path)
}
def void addComponent(String path, IComponent component) {
addComponent(path, component, #{ 'all' -> 'all' })
}
def void addComponent(String path, IComponent component, Map authorizations) {
services.put(path, component)
serviceAuthorizations.put(path, authorizations)
}
def removeComponent(String path) {
val cmp = services.remove(path)
serviceAuthorizations.remove(cmp)
return cmp
}
def getService(String address) {
return services.get('srv:' + address)
}
def void addService(String address, IComponent service) {
addService(address, service, false)
}
def void addService(String address, IComponent service, Map authorizations) {
addService(address, service, false, authorizations)
}
def void addService(String address, IComponent service, boolean asContext) {
addService(address, service, asContext, #{ 'all' -> 'all' })
}
def void addService(String address, IComponent service, boolean asContext, Map authorizations) {
val srvAddress = 'srv:' + address
addComponent(srvAddress, service, authorizations)
if (asContext) ctxServices.add(service)
}
def void removeService(String address) {
val srvAddress = 'srv:' + address
val srv = removeComponent(srvAddress)
ctxServices.remove(srv)
}
def addAuthorization(String path, String cmd, String group) {
var auths = serviceAuthorizations.get(path)
if (auths === null) {
auths = new HashMap
serviceAuthorizations.put(path, auths)
}
auths.put(cmd, group)
}
def boolean isAuthorized(Message msg, UserInfo user) {
val auth = serviceAuthorizations.get(msg.path)
if (auth === null) return false
val allGroup = auth.get('all')
if (allGroup !== null) {
if (allGroup == 'all') return true
if (user === null) return false
return user.groups.contains(allGroup)
}
val methGroup = auth.get(msg.cmd)
if (methGroup !== null) {
if (methGroup == 'all') return true
if (user === null) return false
return user.groups.contains(methGroup)
}
return false
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy