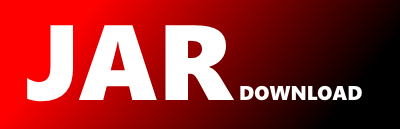
rt.pipeline.pipe.channel.ReceiveBuffer Maven / Gradle / Ivy
package rt.pipeline.pipe.channel;
import com.google.common.base.Objects;
import java.nio.ByteBuffer;
import java.nio.channels.FileChannel;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.nio.file.StandardOpenOption;
import org.eclipse.xtend2.lib.StringConcatenation;
import org.eclipse.xtext.xbase.lib.Exceptions;
import org.eclipse.xtext.xbase.lib.Procedures.Procedure0;
import org.eclipse.xtext.xbase.lib.Procedures.Procedure1;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import rt.pipeline.PathValidator;
import rt.pipeline.pipe.channel.ChannelBuffer;
import rt.pipeline.pipe.channel.ChannelPump;
@SuppressWarnings("all")
public class ReceiveBuffer extends ChannelBuffer {
private final static Logger logger = LoggerFactory.getLogger("BUFFER-RECEIVE");
@Override
public Logger getLogger() {
return ReceiveBuffer.logger;
}
private Procedure1 super String> onBegin = null;
private Procedure1 super ByteBuffer> onData = null;
public void onBegin(final Procedure1 super String> onBegin) {
this.onBegin = onBegin;
}
public void onData(final Procedure1 super ByteBuffer> onData) {
this.onData = onData;
}
public ReceiveBuffer(final ChannelPump inPump, final ChannelPump outPump) {
super(inPump, outPump);
final Procedure1 _function = (ChannelBuffer.Signal signal) -> {
ReceiveBuffer.logger.debug("SIGNAL {}", signal);
boolean _equals = Objects.equal(signal, null);
if (_equals) {
this.error("Received incorrect signal!");
return;
}
boolean _equals_1 = Objects.equal(signal.flag, ChannelBuffer.Signal.SIGNAL_BEGIN);
if (_equals_1) {
if (this.isLocked) {
this.error("Channel is already locked!");
return;
}
this.isLocked = true;
this.needConfirmation = true;
this.signalName = signal.name;
if (this.onBegin!=null) {
this.onBegin.apply(this.signalName);
}
} else {
boolean _equals_2 = Objects.equal(signal.flag, ChannelBuffer.Signal.SIGNAL_END);
if (_equals_2) {
if ((!this.isLocked)) {
this.error("Channel is not locked!");
return;
}
boolean _notEquals = (!Objects.equal(signal.name, this.signalName));
if (_notEquals) {
StringConcatenation _builder = new StringConcatenation();
_builder.append("Signal value != signalName: ");
_builder.append(signal.name, "");
_builder.append(" != ");
_builder.append(this.signalName, "");
this.error(_builder.toString());
return;
}
ChannelBuffer.Signal _endConfirm = ChannelBuffer.Signal.endConfirm(this.signalName);
this.endOk(_endConfirm);
} else {
boolean _equals_3 = Objects.equal(signal.flag, ChannelBuffer.Signal.SIGNAL_ERROR);
if (_equals_3) {
boolean _notEquals_1 = (!Objects.equal(signal.name, this.signalName));
if (_notEquals_1) {
StringConcatenation _builder_1 = new StringConcatenation();
_builder_1.append("Signal value != signalName: ");
_builder_1.append(signal.name, "");
_builder_1.append(" != ");
_builder_1.append(this.signalName, "");
this.error(_builder_1.toString());
return;
}
this.endError(signal.message);
}
}
}
};
inPump.onSignal = _function;
final Procedure1 _function_1 = (ByteBuffer it) -> {
if ((!this.isLocked)) {
this.error("Can not receive data with channel in unlocked state!");
return;
}
int _limit = it.limit();
ReceiveBuffer.logger.debug("SIGNAL-DATA {}B", Integer.valueOf(_limit));
boolean _notEquals = (!Objects.equal(this.fileChannel, null));
if (_notEquals) {
this.writeBufferToFile(it);
} else {
if (this.onData!=null) {
this.onData.apply(it);
}
}
};
inPump.onData = _function_1;
}
public void operator_doubleGreaterThan(final Procedure1 super ByteBuffer> onData) {
if ((!this.isLocked)) {
this.error("Channel is not locked!");
return;
}
this.onData = onData;
this.confirm();
}
public void writeToFile(final String filePath, final Procedure0 onFinal) {
if ((!this.isLocked)) {
this.error("Channel is not locked!");
return;
}
boolean _notEquals = (!Objects.equal(onFinal, null));
if (_notEquals) {
this.onEnd(onFinal);
}
try {
boolean _isValid = PathValidator.isValid(filePath);
boolean _not = (!_isValid);
if (_not) {
StringConcatenation _builder = new StringConcatenation();
_builder.append("Invalid path ");
_builder.append(filePath, "");
this.error(_builder.toString());
return;
}
final Path path = Paths.get(filePath);
Path _parent = path.getParent();
Files.createDirectories(_parent);
FileChannel _open = FileChannel.open(path, StandardOpenOption.CREATE, StandardOpenOption.WRITE);
this.fileChannel = _open;
this.confirm();
} catch (final Throwable _t) {
if (_t instanceof Exception) {
final Exception ex = (Exception)_t;
ex.printStackTrace();
StringConcatenation _builder_1 = new StringConcatenation();
Class extends Exception> _class = ex.getClass();
String _simpleName = _class.getSimpleName();
_builder_1.append(_simpleName, "");
_builder_1.append(": ");
String _message = ex.getMessage();
_builder_1.append(_message, "");
this.error(_builder_1.toString());
} else {
throw Exceptions.sneakyThrow(_t);
}
}
}
public void writeToFile(final String filePath) {
this.writeToFile(filePath, null);
}
private void writeBufferToFile(final ByteBuffer buffer) {
try {
this.fileChannel.write(buffer);
} catch (final Throwable _t) {
if (_t instanceof Exception) {
final Exception ex = (Exception)_t;
ex.printStackTrace();
StringConcatenation _builder = new StringConcatenation();
Class extends Exception> _class = ex.getClass();
String _simpleName = _class.getSimpleName();
_builder.append(_simpleName, "");
_builder.append(": ");
String _message = ex.getMessage();
_builder.append(_message, "");
this.error(_builder.toString());
} else {
throw Exceptions.sneakyThrow(_t);
}
}
}
private void confirm() {
if (this.needConfirmation) {
this.needConfirmation = false;
ChannelBuffer.Signal _beginConfirm = ChannelBuffer.Signal.beginConfirm(this.signalName);
this.outPump.pushSignal(_beginConfirm);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy