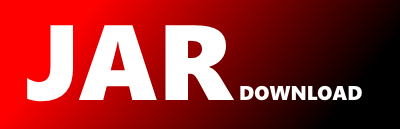
rt.pipeline.pipe.channel.SendBuffer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rts-pipeline Show documentation
Show all versions of rts-pipeline Show documentation
Framework to build reactive services for reactive application front-ends
The newest version!
package rt.pipeline.pipe.channel;
import com.google.common.base.Objects;
import java.nio.ByteBuffer;
import java.nio.channels.FileChannel;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.nio.file.StandardOpenOption;
import java.util.concurrent.atomic.AtomicInteger;
import org.eclipse.xtend2.lib.StringConcatenation;
import org.eclipse.xtext.xbase.lib.Exceptions;
import org.eclipse.xtext.xbase.lib.Functions.Function0;
import org.eclipse.xtext.xbase.lib.Procedures.Procedure0;
import org.eclipse.xtext.xbase.lib.Procedures.Procedure1;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import rt.async.AsyncUtils;
import rt.pipeline.PathValidator;
import rt.pipeline.pipe.channel.ChannelBuffer;
import rt.pipeline.pipe.channel.ChannelPump;
@SuppressWarnings("all")
public class SendBuffer extends ChannelBuffer {
private final static Logger logger = LoggerFactory.getLogger("BUFFER-SEND");
@Override
public Logger getLogger() {
return SendBuffer.logger;
}
private Procedure1 super SendBuffer> onReady = null;
public SendBuffer(final ChannelPump outPump, final ChannelPump inPump) {
super(inPump, outPump);
final Procedure1 _function = (ChannelBuffer.Signal signal) -> {
SendBuffer.logger.debug("BACK-SIGNAL {}", signal);
boolean _equals = Objects.equal(signal, null);
if (_equals) {
this.error("Received incorrect signal!");
return;
}
boolean _notEquals = (!Objects.equal(signal.name, this.signalName));
if (_notEquals) {
StringConcatenation _builder = new StringConcatenation();
_builder.append("Signal confirmation \'");
_builder.append(signal.name, "");
_builder.append("\' != \'");
_builder.append(this.signalName, "");
_builder.append("\' ");
this.endError(_builder.toString());
return;
}
boolean _equals_1 = Objects.equal(signal.flag, ChannelBuffer.Signal.SIGNAL_BEGIN_CONFIRM);
if (_equals_1) {
if (this.needConfirmation) {
this.needConfirmation = false;
if (this.onReady!=null) {
this.onReady.apply(this);
}
}
} else {
boolean _equals_2 = Objects.equal(signal.flag, ChannelBuffer.Signal.SIGNAL_END_CONFIRM);
if (_equals_2) {
if (this.needConfirmation) {
this.endOk();
}
} else {
boolean _equals_3 = Objects.equal(signal.flag, ChannelBuffer.Signal.SIGNAL_ERROR);
if (_equals_3) {
this.endError(signal.message);
}
}
}
};
inPump.onSignal = _function;
}
public void begin(final String name, final Procedure1 super SendBuffer> onReady) {
final Function0 _function = () -> {
return Boolean.valueOf((!this.isLocked));
};
final Procedure0 _function_1 = () -> {
this.isLocked = true;
this.needConfirmation = true;
this.signalName = name;
this.onReady = onReady;
final Procedure0 _function_2 = () -> {
if (this.needConfirmation) {
this.needConfirmation = false;
this.endError("Begin confirmation timeout!");
}
};
AsyncUtils.timeout(_function_2);
ChannelBuffer.Signal _begin = ChannelBuffer.Signal.begin(this.signalName);
this.push(_begin);
};
AsyncUtils.waitUntil(_function, _function_1);
}
public void end() {
if ((!this.isLocked)) {
this.endError("Channel is not locked!");
return;
}
this.needConfirmation = true;
final Procedure0 _function = () -> {
if (this.needConfirmation) {
this.needConfirmation = false;
this.endError("End confirmation timeout!");
}
};
AsyncUtils.timeout(_function);
ChannelBuffer.Signal _end = ChannelBuffer.Signal.end(this.signalName);
this.push(_end);
}
public void operator_doubleLessThan(final ByteBuffer buffer) {
if ((!this.isLocked)) {
this.endError("Can not send data with channel in unlocked state!");
return;
}
final Function0 _function = () -> {
return Boolean.valueOf(this.outPump.isReady());
};
final Procedure0 _function_1 = () -> {
this.outPump.pushData(buffer);
};
AsyncUtils.waitUntil(_function, _function_1);
}
public void sendSliced(final ByteBuffer buffer, final int bufferSize, final Procedure0 onFinal) {
final int limit = buffer.limit();
int _xifexpression = (int) 0;
if ((bufferSize < limit)) {
_xifexpression = bufferSize;
} else {
_xifexpression = limit;
}
buffer.limit(_xifexpression);
final AtomicInteger index = new AtomicInteger(0);
final Function0 _function = () -> {
int _get = index.get();
return Boolean.valueOf((_get < limit));
};
final Function0 _function_1 = () -> {
boolean _isReady = this.outPump.isReady();
if (_isReady) {
this.outPump.pushData(buffer);
final int nextPosition = index.addAndGet(bufferSize);
final int nextLimit = (nextPosition + bufferSize);
int _xifexpression_1 = (int) 0;
if ((nextPosition < limit)) {
_xifexpression_1 = nextPosition;
} else {
_xifexpression_1 = limit;
}
buffer.position(_xifexpression_1);
int _xifexpression_2 = (int) 0;
if ((nextLimit < limit)) {
_xifexpression_2 = nextLimit;
} else {
_xifexpression_2 = limit;
}
buffer.limit(_xifexpression_2);
}
return true;
};
final Procedure0 _function_2 = () -> {
boolean _notEquals = (!Objects.equal(onFinal, null));
if (_notEquals) {
onFinal.apply();
}
};
final Procedure1 _function_3 = (Exception it) -> {
StringConcatenation _builder = new StringConcatenation();
Class extends Exception> _class = it.getClass();
String _simpleName = _class.getSimpleName();
_builder.append(_simpleName, "");
_builder.append(": ");
String _message = it.getMessage();
_builder.append(_message, "");
this.error(_builder.toString());
it.printStackTrace();
};
AsyncUtils.asyncWhile(_function, _function_1, _function_2, _function_3);
}
public void sendSliced(final ByteBuffer buffer, final Procedure0 onFinal) {
this.sendSliced(buffer, (1024 * 1024), onFinal);
}
public void sendFile(final String filePath, final int bufferSize, final Procedure0 onFinal) {
final Procedure1 _function = (SendBuffer it) -> {
try {
SendBuffer.logger.info(("FILE-BEGIN " + filePath));
boolean _notEquals = (!Objects.equal(onFinal, null));
if (_notEquals) {
it.onEnd(onFinal);
}
try {
boolean _isValid = PathValidator.isValid(filePath);
boolean _not = (!_isValid);
if (_not) {
StringConcatenation _builder = new StringConcatenation();
_builder.append("Invalid path ");
_builder.append(filePath, "");
it.endError(_builder.toString());
return;
}
final Path path = Paths.get(filePath);
FileChannel _open = FileChannel.open(path, StandardOpenOption.READ);
it.fileChannel = _open;
} catch (final Throwable _t) {
if (_t instanceof Exception) {
final Exception ex = (Exception)_t;
StringConcatenation _builder_1 = new StringConcatenation();
Class extends Exception> _class = ex.getClass();
String _simpleName = _class.getSimpleName();
_builder_1.append(_simpleName, "");
_builder_1.append(": ");
String _message = ex.getMessage();
_builder_1.append(_message, "");
it.endError(_builder_1.toString());
return;
} else {
throw Exceptions.sneakyThrow(_t);
}
}
final FileChannel fc = it.fileChannel;
final ByteBuffer buffer = ByteBuffer.allocate(bufferSize);
int _read = fc.read(buffer);
final AtomicInteger pos = new AtomicInteger(_read);
final Function0 _function_1 = () -> {
int _get = pos.get();
return Boolean.valueOf((_get > 0));
};
final Function0 _function_2 = () -> {
try {
if ((!it.isLocked)) {
it.endError("Unlocked before transfer conclusion!");
return false;
}
boolean _isReady = it.outPump.isReady();
if (_isReady) {
buffer.flip();
it.outPump.pushData(buffer);
buffer.clear();
int _read_1 = fc.read(buffer);
pos.set(_read_1);
}
return true;
} catch (Throwable _e) {
throw Exceptions.sneakyThrow(_e);
}
};
final Procedure0 _function_3 = () -> {
it.end();
SendBuffer.logger.info(("FILE-END " + filePath));
};
final Procedure1 _function_4 = (Exception it_1) -> {
StringConcatenation _builder_2 = new StringConcatenation();
Class extends Exception> _class_1 = it_1.getClass();
String _simpleName_1 = _class_1.getSimpleName();
_builder_2.append(_simpleName_1, "");
_builder_2.append(": ");
String _message_1 = it_1.getMessage();
_builder_2.append(_message_1, "");
this.error(_builder_2.toString());
it_1.printStackTrace();
};
AsyncUtils.asyncWhile(_function_1, _function_2, _function_3, _function_4);
} catch (Throwable _e) {
throw Exceptions.sneakyThrow(_e);
}
};
this.begin(filePath, _function);
}
public void sendFile(final String filePath) {
this.sendFile(filePath, (1024 * 1024), null);
}
public void sendFile(final String filePath, final int bufferSize) {
this.sendFile(filePath, bufferSize, null);
}
public void sendFile(final String filePath, final Procedure0 onEnd) {
this.sendFile(filePath, (1024 * 1024), onEnd);
}
private void push(final ChannelBuffer.Signal signal) {
SendBuffer.logger.debug("SIGNAL {}", signal);
this.outPump.pushSignal(signal);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy