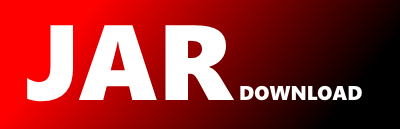
com.github.shyiko.dotenv.guice.DotEnvModule Maven / Gradle / Ivy
package com.github.shyiko.dotenv.guice;
import com.google.inject.AbstractModule;
import com.google.inject.Binder;
import com.google.inject.Key;
import com.github.shyiko.dotenv.DotEnv;
import java.util.Map;
public class DotEnvModule extends AbstractModule {
@FunctionalInterface
public interface TriConsumer {
void accept(T t, U u, V v);
}
public static final TriConsumer DEFAULT_BINDER =
(binder, annotation, value) -> {
binder.bind(Key.get(String.class, annotation)).toInstance(value);
if ("true".equalsIgnoreCase(value) || "false".equalsIgnoreCase(value)) {
binder.bind(Key.get(Boolean.class, annotation)).toInstance(Boolean.parseBoolean(value));
}
try {
int v = Integer.parseInt(value);
binder.bind(Key.get(Integer.class, annotation)).toInstance(v);
} catch (NumberFormatException e) { /* it's fine */ }
try {
long v = Long.parseLong(value);
binder.bind(Key.get(Long.class, annotation)).toInstance(v);
} catch (NumberFormatException e) { /* it's fine */ }
};
private final Map env;
private final TriConsumer binder;
public DotEnvModule() {
this(DotEnv.load(), DEFAULT_BINDER);
}
public DotEnvModule(TriConsumer binder) {
this(DotEnv.load(), binder);
}
public DotEnvModule(Map env) {
this(env, DEFAULT_BINDER);
}
public DotEnvModule(Map env, TriConsumer binder) {
this.env = env;
this.binder = binder;
}
@Override
protected void configure() {
this.env.forEach((key, value) -> binder.accept(this.binder(), new DotEnvValueImpl(key), value));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy