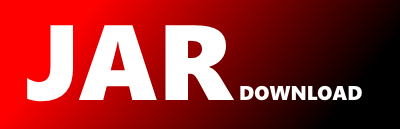
com.github.simonharmonicminor.juu.collection.immutable.ImmutableCollectionUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-useful-utils Show documentation
Show all versions of java-useful-utils Show documentation
Just some useful utils for everyday coding
package com.github.simonharmonicminor.juu.collection.immutable;
import com.github.simonharmonicminor.juu.monad.Try;
import java.util.Objects;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.stream.Collectors;
class ImmutableCollectionUtils {
private ImmutableCollectionUtils() {
}
private static String baseCollectionString(ImmutableCollection collection) {
return collection.stream().map(Objects::toString).collect(Collectors.joining(", "));
}
static String setToString(ImmutableCollection collection) {
return String.format("{%s}", baseCollectionString(collection));
}
static String listToString(ImmutableCollection collection) {
return String.format("[%s]", baseCollectionString(collection));
}
static boolean listEquals(ImmutableList> current, Object other) {
if (current == other) return true;
if (!(other instanceof ImmutableList)) return false;
ImmutableList> otherList = (ImmutableList>) other;
if (otherList.size() != current.size()) return false;
for (int i = 0; i < current.size(); i++) {
if (!Objects.equals(current.get(i), otherList.get(i))) return false;
}
return true;
}
static boolean setEquals(ImmutableSet> current, Object other) {
if (current == other) return true;
if (!(other instanceof ImmutableSet)) return false;
ImmutableSet> otherSet = (ImmutableSet>) other;
if (current.size() != otherSet.size())
return false;
BiConsumer, ImmutableSet>> checkForEquality =
(set1, set2) -> {
for (Object obj : set1) {
if (set2.notContains(obj))
throw new RuntimeException("not equal");
}
};
checkForEquality.accept(current, otherSet);
checkForEquality.accept(otherSet, current);
return true;
}
static boolean pairEquals(Pair, ?> current, Object other) {
if (current == other) return true;
if (!(other instanceof Pair)) return false;
Pair, ?> otherPair = (Pair, ?>) other;
return Objects.equals(current.getKey(), otherPair.getKey())
&& Objects.equals(current.getValue(), otherPair.getValue());
}
static boolean mapEquals(ImmutableMap, ?> current, Object other) {
if (current == other) return true;
if (!(other instanceof ImmutableMap)) return false;
ImmutableMap, ?> otherMap = (ImmutableMap, ?>) other;
if (current.size() != otherMap.size())
return false;
Consumer> checkForEquality = map ->
map.forEach((k, v) -> {
if (current.notContainsKey(k)
|| otherMap.notContainsKey(k)
|| current.get(k) != otherMap.get(k))
throw new RuntimeException("not equal");
});
return Try.of(() -> {
checkForEquality.accept(current);
checkForEquality.accept(otherMap);
return true;
}).orElse(false);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy