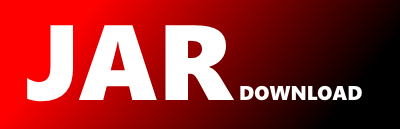
com.github.simonharmonicminor.juu.collection.immutable.ImmutableHashSet Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-useful-utils Show documentation
Show all versions of java-useful-utils Show documentation
Just some useful utils for everyday coding
package com.github.simonharmonicminor.juu.collection.immutable;
import java.io.Serializable;
import java.util.HashSet;
import java.util.Iterator;
import java.util.Objects;
import java.util.Set;
import java.util.function.Function;
import java.util.function.Predicate;
import java.util.stream.Stream;
import static com.github.simonharmonicminor.juu.collection.immutable.Immutable.listOf;
import static com.github.simonharmonicminor.juu.collection.immutable.ImmutableCollectionUtils.setEquals;
import static com.github.simonharmonicminor.juu.collection.immutable.ImmutableCollectionUtils.setToString;
/**
* An immutable implementation of java native {@link HashSet}
*
* @param the type of the content
* @see ImmutableSet
* @see Set
* @see HashSet
* @see Serializable
* @since 1.0
*/
public class ImmutableHashSet implements ImmutableSet, Serializable {
private final HashSet hashSet;
public ImmutableHashSet(Iterable iterable) {
this(iterable, true);
}
ImmutableHashSet(Iterable iterable, boolean needsCloning) {
Objects.requireNonNull(iterable);
if (iterable instanceof HashSet) {
this.hashSet = needsCloning ? new HashSet<>((HashSet) iterable) : (HashSet) iterable;
} else if (iterable instanceof ImmutableHashSet) {
ImmutableHashSet immutableHashSet = (ImmutableHashSet) iterable;
this.hashSet = immutableHashSet.hashSet;
} else {
hashSet = new HashSet<>();
for (T element : iterable) {
hashSet.add(element);
}
}
}
private static ImmutableSet newImmutableHashSetWithoutCloning(Set set) {
if (set.isEmpty()) return Immutable.emptySet();
return new ImmutableHashSet<>(set, false);
}
@Override
public ImmutableSet concatWith(Iterable iterable) {
Objects.requireNonNull(iterable);
HashSet newHashSet = new HashSet<>(this.hashSet);
for (T t : iterable) {
newHashSet.add(t);
}
return newImmutableHashSetWithoutCloning(newHashSet);
}
@Override
public ImmutableSet map(Function super T, ? extends R> mapper) {
Objects.requireNonNull(mapper);
HashSet newHashSet = new HashSet<>(size());
for (T t : this) {
newHashSet.add(mapper.apply(t));
}
return newImmutableHashSetWithoutCloning(newHashSet);
}
@Override
public ImmutableSet flatMap(Function super T, ? extends Iterable> mapper) {
Objects.requireNonNull(mapper);
HashSet newHashSet = new HashSet<>(size());
for (T t : this) {
ImmutableHashSet immutableHashSet = new ImmutableHashSet<>(mapper.apply(t));
newHashSet.addAll(immutableHashSet.hashSet);
}
return newImmutableHashSetWithoutCloning(newHashSet);
}
@Override
public ImmutableSet filter(Predicate super T> predicate) {
Objects.requireNonNull(predicate);
HashSet newHashSet = new HashSet<>(size());
for (T t : this) {
if (predicate.test(t)) newHashSet.add(t);
}
return newImmutableHashSetWithoutCloning(newHashSet);
}
@Override
public int size() {
return hashSet.size();
}
@Override
public boolean contains(Object element) {
return hashSet.contains(element);
}
@Override
public ImmutableList toList() {
return listOf(this.hashSet);
}
@Override
public ImmutableSet toSet() {
return this;
}
@Override
public Stream parallelStream() {
return this.hashSet.parallelStream();
}
@Override
public Stream stream() {
return this.hashSet.stream();
}
@Override
public Iterator iterator() {
return hashSet.iterator();
}
@Override
public boolean equals(Object o) {
return setEquals(this, o);
}
@Override
public int hashCode() {
return Objects.hash(hashSet);
}
@Override
public String toString() {
return setToString(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy