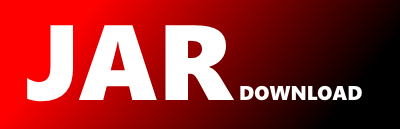
com.github.simonharmonicminor.juu.collection.immutable.ImmutableNavigableSet Maven / Gradle / Ivy
Show all versions of java-useful-utils Show documentation
package com.github.simonharmonicminor.juu.collection.immutable;
import java.util.*;
/**
* @param
* @see NavigableSet
*/
public interface ImmutableNavigableSet extends ImmutableSortedSet {
/**
* Returns the greatest element in this set strictly less than the given element, or {@code null}
* if there is no such element.
*
* @param t the value to match
* @return the greatest element less than {@code t}, or {@code null} if there is no such element
*/
Optional lower(T t);
/**
* Returns the greatest element in this set less than or equal to the given element, or {@code
* null} if there is no such element.
*
* @param t the value to match
* @return the greatest element less than or equal to {@code t}, or {@code null} if there is no
* such element
*/
Optional floor(T t);
/**
* Returns the least element in this set greater than or equal to the given element, or {@code
* null} if there is no such element.
*
* @param t the value to match
* @return the least element greater than or equal to {@code t}, or {@code null} if there is no
* such element
*/
Optional ceiling(T t);
/**
* Returns the least element in this set strictly greater than the given element, or {@code null}
* if there is no such element.
*
* @param t the value to match
* @return the least element greater than {@code t}, or {@code null} if there is no such element
*/
Optional higher(T t);
/**
* Returns a reverse order view of the elements contained in this set. The descending set is
* backed by this set, so changes to the set are reflected in the descending set, and vice-versa.
* If either set is modified while an iteration over either set is in progress (except through the
* iterator's own {@code remove} operation), the results of the iteration are undefined.
*
* The returned set has an ordering equivalent to {@link Collections#reverseOrder(Comparator)
* Collections.reverseOrder}{@code (comparator())}. The expression {@code
* s.descendingSet().descendingSet()} returns a view of {@code s} essentially equivalent to {@code
* s}.
*
* @return a reverse order view of this set
*/
ImmutableNavigableSet reversedOrderSet();
/**
* Returns an iterator over the elements in this set, in descending order. Equivalent in effect to
* {@code reversedOrderSet().iterator()}.
*
* @return an iterator over the elements in this set, in descending order
*/
Iterator reversedOrderIterator();
/**
* Returns a view of the portion of this set whose elements range from {@code fromElement} to
* {@code toElement}. If {@code fromElement} and {@code toElement} are equal, the returned set is
* empty unless {@code fromInclusive} and {@code toInclusive} are both true. The returned set is
* backed by this set, so changes in the returned set are reflected in this set, and vice-versa.
* The returned set supports all optional set operations that this set supports.
*
* The returned set will throw an {@code IllegalArgumentException} on an attempt to insert an
* element outside its range.
*
* @param fromElement low endpoint of the returned set
* @param fromInclusive {@code true} if the low endpoint is to be included in the returned view
* @param toElement high endpoint of the returned set
* @param toInclusive {@code true} if the high endpoint is to be included in the returned view
* @return a view of the portion of this set whose elements range from {@code fromElement},
* inclusive, to {@code toElement}, exclusive
*/
ImmutableNavigableSet subSet(
T fromElement, boolean fromInclusive, T toElement, boolean toInclusive);
/**
* Returns a view of the portion of this set whose elements are less than (or equal to, if {@code
* inclusive} is true) {@code toElement}. The returned set is backed by this set, so changes in
* the returned set are reflected in this set, and vice-versa. The returned set supports all
* optional set operations that this set supports.
*
* The returned set will throw an {@code IllegalArgumentException} on an attempt to insert an
* element outside its range.
*
* @param toElement high endpoint of the returned set
* @param inclusive {@code true} if the high endpoint is to be included in the returned view
* @return a view of the portion of this set whose elements are less than (or equal to, if {@code
* inclusive} is true) {@code toElement}
*/
ImmutableNavigableSet headSet(T toElement, boolean inclusive);
/**
* Returns a view of the portion of this set whose elements are greater than (or equal to, if
* {@code inclusive} is true) {@code fromElement}. The returned set is backed by this set, so
* changes in the returned set are reflected in this set, and vice-versa. The returned set
* supports all optional set operations that this set supports.
*
* The returned set will throw an {@code IllegalArgumentException} on an attempt to insert an
* element outside its range.
*
* @param fromElement low endpoint of the returned set
* @param inclusive {@code true} if the low endpoint is to be included in the returned view
* @return a view of the portion of this set whose elements are greater than or equal to {@code
* fromElement}
*/
ImmutableNavigableSet tailSet(T fromElement, boolean inclusive);
/**
* Converts this immutable navigable set to mutable one. Creates new object, so its mutations does
* not affect the immutable one.
*
* @return new mutable navigable set
*/
NavigableSet toMutableNavigableSet();
}