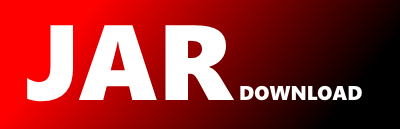
fr.simply.StubServer.scala Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of simplyscala-server_2.9.2 Show documentation
Show all versions of simplyscala-server_2.9.2 Show documentation
provides a fast & ultra-lightweight http server with an API dedicated to define server’s routes (request-response), start & stop server
The newest version!
package fr.simply
import org.simpleframework.transport.connect.SocketConnection
import java.net.{BindException, InetSocketAddress, SocketAddress}
import util.{Text_Plain, ContentType}
import org.simpleframework.http.Request
case class ServerRoute(restVerb: RestVerb,
path: String,
response: ServerResponse,
params: Map[String, String] = Map())
trait ServerResponse
case class StaticServerResponse(contentType: ContentType, body: String, code: Int) extends ServerResponse
case class DynamicServerResponse(response: Request => StaticServerResponse) extends ServerResponse
object GET {
def apply(path: String, params: Map[String, String] = Map(), response: ServerResponse): ServerRoute =
ServerRoute(Get, path, response, params)
}
object POST {
def apply(path: String, params: Map[String, String] = Map(), response: ServerResponse): ServerRoute =
ServerRoute(Post, path, response, params)
}
class StubServer(port: Int, routes: ServerRoute*) {
private var defaultResponse = StaticServerResponse(Text_Plain, "error", 404)
private var simplyServer: SocketConnection = _
private var portUsed = port
def portInUse: Int = portUsed
def start: StubServer = {
this.simplyServer = startServer
this
}
def stop = if (simplyServer != null) simplyServer.close()
def defaultResponse(contentType: ContentType, body: String, responseCode: Int): StubServer = {
this.defaultResponse = StaticServerResponse(contentType, body, responseCode)
this
}
private def startServer: SocketConnection = {
val container = new SimplyScala(defaultResponse, routes.toList)
val connection = new SocketConnection(container)
startServerWithAvailablePort(connection, port)
}
private def startServerWithAvailablePort(connection: SocketConnection, port: Int): SocketConnection = {
val address: SocketAddress = new InetSocketAddress(port)
try {
connection.connect(address)
portUsed = port
} catch {
case e: BindException => startServerWithAvailablePort(connection, port + 1)
}
connection
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy