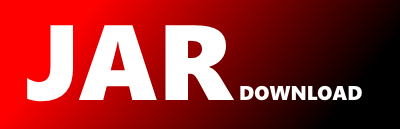
com.github.simy4.xpath.expr.PredicateExpr Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of xpath-to-xml-core Show documentation
Show all versions of xpath-to-xml-core Show documentation
Convenient utility to build XML models by evaluating XPath expressions
package com.github.simy4.xpath.expr;
import com.github.simy4.xpath.XmlBuilderException;
import com.github.simy4.xpath.navigator.Node;
import com.github.simy4.xpath.view.AbstractViewVisitor;
import com.github.simy4.xpath.view.BooleanView;
import com.github.simy4.xpath.view.NumberView;
import com.github.simy4.xpath.view.View;
import com.github.simy4.xpath.view.ViewContext;
public class PredicateExpr implements Expr {
private final Expr predicate;
public PredicateExpr(Expr predicate) {
this.predicate = predicate;
}
@Override
public View resolve(ViewContext context) throws XmlBuilderException {
return predicate.resolve(context).visit(new PredicateVisitor(context));
}
@Override
public String toString() {
return "[" + predicate + "]";
}
private static final class PredicateVisitor extends AbstractViewVisitor> {
private final ViewContext context;
private PredicateVisitor(ViewContext context) {
this.context = context;
}
@Override
public View visit(NumberView numberView) throws XmlBuilderException {
final double number = numberView.toNumber();
if (0 == Double.compare(number, context.getPosition())) {
context.getCurrent().mark();
return BooleanView.of(true);
} else if (context.isGreedy() && !context.hasNext() && number > context.getPosition()
&& context.getCurrent().isNew()) {
final T node = context.getCurrent().getNode();
long numberOfNodesToCreate = (long) number - context.getPosition();
do {
context.getNavigator().prependCopy(node);
} while (--numberOfNodesToCreate > 0);
return BooleanView.of(true);
} else {
return BooleanView.of(false);
}
}
@Override
protected View returnDefault(View view) throws XmlBuilderException {
return view;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy