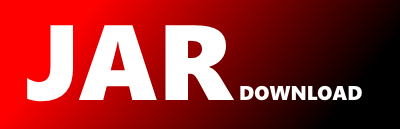
com.github.simy4.xpath.dom.navigator.DomElementsIterable Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of xpath-to-xml-dom Show documentation
Show all versions of xpath-to-xml-dom Show documentation
Convenient utility to build XML models by evaluating XPath expressions
package com.github.simy4.xpath.dom.navigator;
import org.w3c.dom.Node;
import java.util.Iterator;
import java.util.NoSuchElementException;
final class DomElementsIterable implements Iterable {
private final Node parent;
DomElementsIterable(Node parent) {
this.parent = parent;
}
@Override
public Iterator iterator() {
return new DomElementsIterator(parent);
}
private static final class DomElementsIterator implements Iterator {
private Node child;
private DomElementsIterator(Node parent) {
this.child = parent.getFirstChild();
}
@Override
public boolean hasNext() {
return null != child;
}
@Override
public DomNode next() {
if (!hasNext()) {
throw new NoSuchElementException("No more elements");
}
final Node next = child;
child = next.getNextSibling();
return new DomNode(next);
}
@Override
public void remove() {
throw new UnsupportedOperationException("remove");
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy