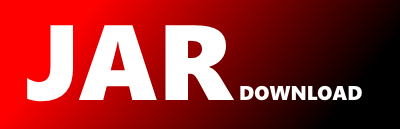
com.github.simy4.xpath.helpers.OrderedProperties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of xpath-to-xml-test Show documentation
Show all versions of xpath-to-xml-test Show documentation
Convenient utility to build XML models by evaluating XPath expressions
package com.github.simy4.xpath.helpers;
import java.util.Collections;
import java.util.Enumeration;
import java.util.LinkedHashMap;
import java.util.LinkedHashSet;
import java.util.Map;
import java.util.Properties;
import java.util.Set;
/**
* Properties that preserves the order in which they were read from the file.
*
* @author Alex Simkin
*/
public class OrderedProperties extends Properties {
private static final long serialVersionUID = 1L;
private final Set
© 2015 - 2025 Weber Informatics LLC | Privacy Policy