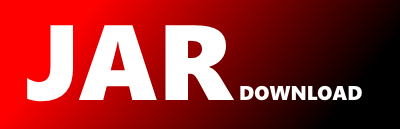
com.github.sirikid.iterators.SingletonIterator Maven / Gradle / Ivy
/*
* Copyright (c) 2015-2016, Ivan Sokolov. All rights reserved.
* This code is licensed under MIT license (see LICENSE for details)
*/
package com.github.sirikid.iterators;
import java.util.Iterator;
import java.util.NoSuchElementException;
import javax.annotation.Nullable;
/**
* Iterator, that contains only one element.
*
* @author Ivan Sokolov
* @version 1.0.0
* @param type of element
* @see SingletonSpliterator
* @since 1.0.0
*/
public class SingletonIterator implements Iterator {
@Nullable private final T value;
private boolean alreadyUsed;
/**
* Sole constructor.
*
* @param value the value to be in iterator
*/
public SingletonIterator(@Nullable T value) {
this.value = value;
alreadyUsed = false;
}
/** {@inheritDoc} */
@Override
public boolean hasNext() {
return !alreadyUsed;
}
/** {@inheritDoc} */
@Nullable
@Override
public T next() {
if (!hasNext()) throw new NoSuchElementException();
// final T result = value;
// value = null; // XXX Shall I assign null for proper GC working or retain this link?
alreadyUsed = true;
// return result;
return value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy