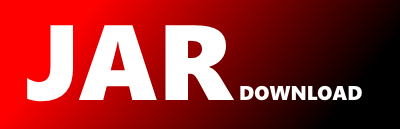
com.github.sirikid.iterators.SingletonSpliterator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of maybe Show documentation
Show all versions of maybe Show documentation
Implementation Maybe monad in Java
/*
* Copyright (c) 2015-2016, Ivan Sokolov. All rights reserved.
* This code is licensed under MIT license (see LICENSE for details)
*/
package com.github.sirikid.iterators;
import java.util.Spliterator;
import java.util.function.Consumer;
import javax.annotation.Nullable;
import static java.util.Objects.requireNonNull;
/**
* Spliterator, that contains only one element.
*
* @author Ivan Sokolov
* @version 1.0.0
* @param type of element
* @see SingletonIterator
* @since 1.0.0
*/
public class SingletonSpliterator implements Spliterator {
@Nullable private final T value;
private boolean alreadyUsed;
/**
* Sole constructor.
*
* @param value the value to be in spliterator
*/
public SingletonSpliterator(@Nullable T value) {
this.value = value;
alreadyUsed = false;
}
/** {@inheritDoc} */
@Override
public boolean tryAdvance(Consumer super T> action) {
requireNonNull(action);
if (!alreadyUsed) {
action.accept(value);
// value = null; // See line 48 in SingletonIterator.java
alreadyUsed = true;
return true;
}
return false;
}
/** {@inheritDoc} */
@Nullable
@Override
public Spliterator trySplit() {
return null;
}
/** {@inheritDoc} */
@Override
public long estimateSize() {
return alreadyUsed ? 0 : 1;
}
/** {@inheritDoc} */
@Override
public int characteristics() {
return DISTINCT | SIZED | SUBSIZED | IMMUTABLE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy