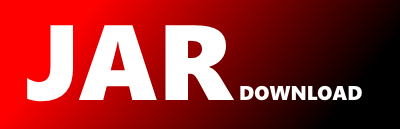
com.github.sitture.envconfig.EnvConfigProperties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of env-config Show documentation
Show all versions of env-config Show documentation
A simple utility to manage environment configs in Java-based projects by merging *.properties files with environment variables overrides.
package com.github.sitture.envconfig;
import java.io.File;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import java.util.Optional;
import java.util.stream.Collectors;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
class EnvConfigProperties {
private static final Logger LOG = LoggerFactory.getLogger(EnvConfigProperties.class);
private final List environments;
private final Path configDir;
private final Path configProfilesPath;
EnvConfigProperties() {
configDir = getConfigPath();
configProfilesPath = getConfigProfilePath();
environments = getEnvList();
}
String getBuildDir() {
return Paths.get(Optional.ofNullable(System.getProperty("project.build.directory"))
.orElse(System.getProperty("user.dir"))).toString();
}
List getEnvironments() {
return environments;
}
String getCurrentEnvironment() {
return environments.get(0);
}
String getConfigProfile() {
return getConfigProperty(EnvConfigKey.CONFIG_PROFILE, "");
}
private List getEnvList() {
final List environments = new ArrayList<>();
environments.add(EnvConfigUtils.CONFIG_ENV_DEFAULT);
environments.addAll(EnvConfigUtils.getListOfValues(getConfigProperty(EnvConfigKey.CONFIG_ENV, EnvConfigUtils.CONFIG_ENV_DEFAULT).toLowerCase(), EnvConfigUtils.CONFIG_DELIMITER_DEFAULT));
Collections.reverse(environments);
return environments.stream().distinct().collect(Collectors.toList());
}
Path getConfigPath(final String env) {
return Paths.get(this.configDir.toString(), env);
}
private Path getConfigPath() {
return getPath(Paths.get(getConfigProperty(EnvConfigKey.CONFIG_PATH, EnvConfigUtils.CONFIG_PATH_DEFAULT)).toAbsolutePath());
}
Path getConfigProfilePath(final String env, final String configProfile) {
return Paths.get(this.configProfilesPath.toString(), env, configProfile);
}
private Path getConfigProfilePath() {
return getPath(Paths.get(getConfigProperty(EnvConfigKey.CONFIG_PROFILES_PATH, this.configDir.toString())).toAbsolutePath());
}
private Path getPath(final Path configPath) {
final File configDir = configPath.toFile();
if (!configDir.exists() || !configDir.isDirectory()) {
throw new EnvConfigException(
"'" + configPath + "' does not exist or not a valid config directory!");
}
return configPath;
}
private String getConfigProperty(final EnvConfigKey key, final String defaultValue) {
return Optional.ofNullable(System.getProperty(key.getProperty()))
.orElse(Optional.ofNullable(getEnvByPropertyKey(key))
.orElse(defaultValue));
}
private String getEnvByPropertyKey(final EnvConfigKey key) {
LOG.debug("Getting {} from system.env", key);
return Optional.ofNullable(System.getenv(EnvConfigUtils.getProcessedEnvKey(key.getProperty())))
.orElse(System.getenv(key.getProperty()));
}
private String getRequiredConfigProperty(final EnvConfigKey key) {
return Optional.ofNullable(getConfigProperty(key, null))
.orElseThrow(() -> new EnvConfigException(String.format("Missing required variable '%s'", key.getProperty())));
}
boolean isConfigKeepassEnabled() {
return Boolean.parseBoolean(getConfigProperty(EnvConfigKey.CONFIG_KEEPASS_ENABLED, "false"));
}
EnvConfigKeepassProperties getKeepassProperties() {
return new EnvConfigKeepassProperties(new File(getConfigProperty(EnvConfigKey.CONFIG_KEEPASS_FILENAME, getBuildDir())).getName(),
getRequiredConfigProperty(EnvConfigKey.CONFIG_KEEPASS_MASTERKEY));
}
boolean isConfigVaultEnabled() {
return Boolean.parseBoolean(getConfigProperty(EnvConfigKey.CONFIG_VAULT_ENABLED, "false"));
}
EnvConfigVaultProperties getVaultProperties() {
return new EnvConfigVaultProperties(getRequiredConfigProperty(EnvConfigKey.CONFIG_VAULT_ADDRESS),
getRequiredConfigProperty(EnvConfigKey.CONFIG_VAULT_NAMESPACE),
getRequiredConfigProperty(EnvConfigKey.CONFIG_VAULT_TOKEN),
getRequiredConfigProperty(EnvConfigKey.CONFIG_VAULT_SECRET_PATH),
getConfigProperty(EnvConfigKey.CONFIG_VAULT_DEFAULT_PATH, null),
Integer.parseInt(getConfigProperty(EnvConfigKey.CONFIG_VAULT_VALIDATE_MAX_RETRIES, "5")));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy