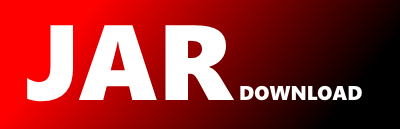
com.github.siwenyan.common.DoubleAction Maven / Gradle / Ivy
package com.github.siwenyan.common;
import org.apache.log4j.Logger;
public class DoubleAction implements IAction {
private static Logger log = Logger.getLogger(DoubleAction.class);
private final IAction action;
private final IAction recover;
private final String trace;
private final long breakMillis;
public DoubleAction(IAction action, IAction recover, String trace, long breakMillis) {
this.action = action;
if (null == recover) {
this.recover = IAction.NO_ACTION;
} else {
this.recover = recover;
}
this.trace = trace;
this.breakMillis = breakMillis;
}
public DoubleAction(IAction action, String trace, long breakMillis) {
this(action, null, trace, breakMillis);
}
@Override
public boolean execute() {
try {
log.debug(trace + "Take a break before first action.");
Sys.sleep(breakMillis);
log.debug(trace + "First Action ...");
if (action.execute()) {
log.debug(trace + "... first action success.");
return true;
} else {
log.debug(trace + "... first action fail.");
throw new RuntimeException("Throw Exception to handover to second action.");
}
} catch (Exception e) {
log.debug(trace + "... first action error: " + e.getMessage());
try {
log.debug(trace + "Try recover before second action.");
if (recover.execute()) {
log.debug(trace + "Take a break before second action.");
Sys.sleep(breakMillis);
log.debug("Second Action ...");
if (action.execute()) {
log.debug(trace + "... second action success");
return true;
} else {
log.debug(trace + "... second action fail.");
return false;
}
} else {
log.debug(trace + "Recover fail.");
return false;
}
} catch (Exception e1) {
log.debug(trace + "... recover or second action error: " + e.getMessage());
return false;
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy