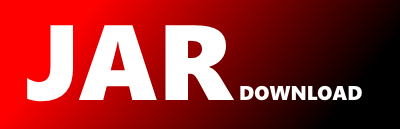
com.github.siwenyan.common.MyEnvironment Maven / Gradle / Ivy
package com.github.siwenyan.common;
import com.github.siwenyan.dish_parser.Constants;
import java.io.*;
import java.util.HashSet;
import java.util.Map;
import java.util.Set;
import java.util.TreeSet;
public class MyEnvironment {
private MapStringToObject
© 2015 - 2024 Weber Informatics LLC | Privacy Policy