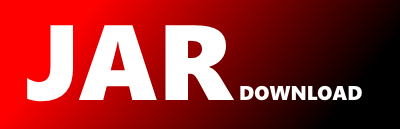
com.github.siwenyan.profile.QaManager Maven / Gradle / Ivy
package com.github.siwenyan.profile;
import com.github.siwenyan.common.EasyJson;
import com.github.siwenyan.common.StringTools;
import com.github.siwenyan.common.Sys;
import org.apache.commons.io.FileUtils;
import java.io.File;
import java.util.*;
public class QaManager {
private Map qas = new LinkedHashMap<>();
private Map qaByFeature = new LinkedHashMap<>();
private List featureKeys = new LinkedList<>();
protected QaManager(String qaRootFolder) {
File rootFolder = Sys.findFile(qaRootFolder);
if (!rootFolder.isDirectory()) {
throw new RuntimeException("No such directory: " + qaRootFolder);
}
Set criteriaKeys = new LinkedHashSet<>();
for (File file : FileUtils.listFiles(rootFolder, new String[]{"json"}, true)) {
Qa qa = (Qa) EasyJson.readValue(file, Qa.class);
if (null == qa) {
throw new RuntimeException("Unable to read: " + file);
}
if (null != qas.put(qa.getName(), qa)) {
throw new RuntimeException("Duplicated qa name: " + qa.getName());
}
qa.setSource(file.getAbsolutePath());
criteriaKeys.addAll(qa.getCriteria().keySet());
}
featureKeys.addAll(criteriaKeys);
calculateQaByFeature();
}
public Qa getQa(Map pf) {
String feature = getFeature(pf);
return qaByFeature.get(feature);
}
public String getFeature(Map pf) {
String feature = "";
for (String key : featureKeys) {
String value = pf.get(key);
if (!StringTools.isEmpty(value)) {
feature += key + "=" + value + ", ";
}
}
feature = feature.substring(0, feature.length() - 2);
return feature;
}
private void calculateQaByFeature() {
qaByFeature.clear();
for (Qa qa : qas.values()) {
String feature = getFeature(qa.getCriteria());
Qa dupQa = qaByFeature.put(feature, qa);
if (null != dupQa) {
throw new RuntimeException("Duplicated feature [" + feature + "] with " + qa.getName() + " and " + dupQa.getName());
}
}
}
public Set getNames() {
return this.qas.keySet();
}
public Set getFeatures() {
return this.qaByFeature.keySet();
}
public List getFeatureKeys() {
return new ArrayList<>(featureKeys);
}
protected void merge(QaManager anotherQaManager) {
this.qas.putAll(anotherQaManager.qas);
Set allKeys = new LinkedHashSet<>();
allKeys.addAll(this.featureKeys);
allKeys.addAll(anotherQaManager.featureKeys);
this.featureKeys.clear();
this.featureKeys.addAll(allKeys);
calculateQaByFeature();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy