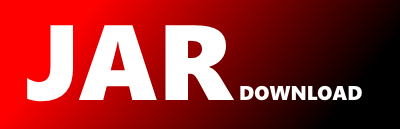
com.github.siwenyan.superglue.team.PageWorkerBase Maven / Gradle / Ivy
package com.github.siwenyan.superglue.team;
import com.github.siwenyan.common.DateTimeTools;
import com.github.siwenyan.common.StringTools;
import com.github.siwenyan.common.Sys;
import com.github.siwenyan.common.WhiteBoard;
import com.github.siwenyan.superglue.AbstractStepSet;
import com.github.siwenyan.web.WebUtils;
import cucumber.api.Scenario;
import org.openqa.selenium.WebDriver;
import java.util.LinkedHashMap;
import java.util.Map;
public abstract class PageWorkerBase extends AbstractStepSet {
//page control options
public static final String STOP_AT_PAGE_BEGIN = "stopAtPageBegin";
public static final String STOP_AT_PAGE = "stopAtPage";
public static final String STOP_AT_NEXT_PAGE_BEGIN = "stopAtNextPageBegin";
public static final String CONTINUE_FROM_PAGE = "continueFromPage";
protected final Map pf;
private final String objectNameKey;
protected Map> outcome = new LinkedHashMap<>();
public PageWorkerBase(Scenario scenario, WebDriver webDriver, Map pf, String objectNameKey) {
super(scenario, webDriver);
this.pf = pf;
this.objectNameKey = objectNameKey;
}
public Map> getOutcome() {
return this.outcome;
}
public abstract boolean accept();
public abstract void work();
public abstract void handOff();
public abstract String getPageName();
public String getShortPageName() {
return StringTools.shortName(this.getPageName());
}
public void tryStopAtPageBegin() throws StopAtPageBeginException {
WebUtils.demoBreakPoint(scenario(), null, "Stop at page begin: " + StringTools.shortName(this.getPageName()));
String objectName = pf.get(objectNameKey);
String prefix = StringTools.isEmpty(objectName) ? "" : (objectName + "_");
WhiteBoard.getInstance().putMap(getOwner(), prefix + getShortPageName(), collectStatus());
if (pf.containsKey(STOP_AT_PAGE_BEGIN) && getPageName().contains(pf.get(STOP_AT_PAGE_BEGIN))) {
throw new StopAtPageBeginException(getPageName());
}
}
public void tryStopAtPage() throws StopAtPageException {
if ("true".equalsIgnoreCase(Sys.conf.getProperty("demo"))) {
WebUtils.demoBreakPoint(scenario(), null, "Stop at page: " + StringTools.shortName(this.getPageName()));
}
if (pf.containsKey(STOP_AT_PAGE) && getPageName().contains(pf.get(STOP_AT_PAGE))) {
throw new StopAtPageException(getPageName());
}
}
public Map collectStatus() {
Map status = new LinkedHashMap<>();
status.put("timestamp", DateTimeTools.timestamp());
return status;
}
public void handOffRecover(Exception e) {
// do nothing
}
public boolean acceptRecover(Exception e) {
// return false means no need to recover for the second chance until check
return false;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy