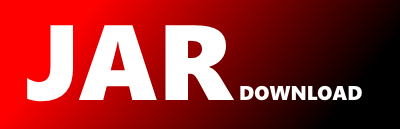
com.github.siwenyan.si.ExecutorManager Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of side Show documentation
Show all versions of side Show documentation
Java runner of Selenium IDE project
package com.github.siwenyan.si;
import java.util.HashMap;
import java.util.Map;
public class ExecutorManager {
private static ExecutorManager instance = new ExecutorManager();
public static ExecutorManager getInstance() {
return instance;
}
private Map executors = new HashMap();
private Map> customExecutors = new HashMap>();
private ExecutorManager() {
this.executors.put("store", new SiCommandExecutorStore());
this.executors.put("run", new SiCommandExecutorRun());
this.executors.put("open", new SiCommandExecutorOpen());
this.executors.put("click", new SiCommandExecutorClick());
this.executors.put("type", new SiCommandExecutorType());
this.executors.put("setWindowSize", new SiCommandExecutorSetWindowSize());
this.executors.put("sendKeys", new SiCommandExecutorSendKeys());
this.executors.put("waitForElementVisible", new SiCommandExecutorWaitForElementVisible());
this.executors.put("storeText", new SiCommandExecutorStoreText());
this.executors.put("echo", new SiCommandExecutorEcho());
this.executors.put("if", new SiCommandExecutorIf());
this.executors.put("else", new SiCommandExecutorElse());
this.executors.put("end", new SiCommandExecutorEnd());
this.executors.put("selectWindow", new SiCommandExecutorSelectWindow());
this.executors.put("select", new SiCommandExecutorSelect());
this.executors.put("waitForElementEditable", new SiCommandExecutorWaitForElementEditable());
this.executors.put("pause", new SiCommandExecutorPause());
this.executors.put("storeTitle", new SiCommandExecutorStoreTitle());
this.executors.put("runScript", new SiCommandExecutorRunScript());
this.executors.put("assert", new SiCommandExecutorAssert());
this.executors.put("verifyText", new SiCommandExecutorVerifyText());
this.executors.put("waitForElementPresent", new SiCommandExecutorWaitForElementPresent());
this.executors.put("verifyElementPresent", new SiCommandExecutorVerifyElementPresent());
this.executors.put("verifyElementNotPresent", new SiCommandExecutorVerifyElementNotPresent());
this.executors.put("verifyValue", new SiCommandExecutorVerifyValue());
this.executors.put("waitForElementNotVisible", new SiCommandExecutorWaitForElementNotVisible());
this.executors.put("verify", new SiCommandExecutorVerify());
this.executors.put("storeXpathCount", new SiCommandExecutorStoreXpathCount());
this.executors.put("//", new SiCommandExecutor() {
@Override
public void execute(SiCommand command, SiContext siContext) throws SiVerifyException, SiWebDriverException {
if (command.getSiModelCommand().getCommand().matches("//\\s*pause.*")) {
if (siContext.isIE() || command.getSiTest().getSiContext().isRetrying()) {
siContext.getExecutorManager().getBasicExecutor("pause").execute(command, siContext);
}
}
}
});
}
protected SiCommandExecutor getExecutor(SiCommand command) {
String sCommand = command.getCommand();
if (command instanceof SiCommandComment) {
return this.executors.get("//");
}
if (this.executors.containsKey(sCommand)) {
if (this.customExecutors.containsKey(sCommand)) {
String cusCommand = command.getSiModelCommand().getTarget();
for (String customCommandName : this.customExecutors.get(sCommand).keySet()) {
if (cusCommand.equals(customCommandName)) {
return this.customExecutors.get(sCommand).get(customCommandName);
}
}
}
return this.executors.get(sCommand);
} else {
throw new RuntimeException("Unsupported command: " + sCommand);
}
}
public void putCustomExecutor(String commandName, String customCommandName, SiCommandExecutor customExecutor) {
if (!this.executors.containsKey(commandName)) {
throw new RuntimeException("No such command " + commandName + " to be tunneled.");
}
if (!this.customExecutors.containsKey(commandName)) {
this.customExecutors.put(commandName, new HashMap());
}
Map customExecutorByRegex = this.customExecutors.get(commandName);
if (customExecutorByRegex.containsKey(customCommandName)) {
throw new RuntimeException("Duplicated regex [" + customCommandName + "] for tunnel command " + commandName + ".");
} else {
customExecutorByRegex.put(customCommandName, customExecutor);
}
}
protected SiCommandExecutor getBasicExecutor(String sCommand) {
return this.executors.get(sCommand);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy