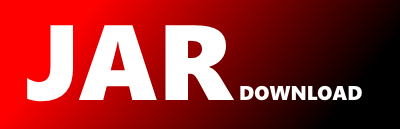
com.github.siwenyan.si.SiCommand Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of side Show documentation
Show all versions of side Show documentation
Java runner of Selenium IDE project
package com.github.siwenyan.si;
import com.github.siwenyan.si.model.SiModelCommand;
import org.jetbrains.annotations.Nullable;
import org.openqa.selenium.WebElement;
public class SiCommand {
public static SiCommand createSiCommand(SiModelCommand siModelCommand, SiTest siTest) {
String commandName = siModelCommand.getCommand();
if (commandName.startsWith("//")) {
return new SiCommandComment(siModelCommand, siTest);
}
switch (commandName) {
case "if":
return new SiCommandIf(siModelCommand, siTest);
case "else":
return new SiCommandElse(siModelCommand, siTest);
case "end":
return new SiCommandEnd(siModelCommand, siTest);
default:
return new SiCommand(siModelCommand, siTest);
}
}
private final SiModelCommand siModelCommand;
private SiTest siTest;
private int indexInTest;
private SiCommand[] pairCommand = new SiCommand[2];
protected SiCommand(SiModelCommand siModelCommand, SiTest siTest) {
this.siModelCommand = siModelCommand;
this.siTest = siTest;
}
@Override
public String toString() {
String s = "#" + (this.indexInTest + 1) + "." + this.siModelCommand.toString();
return s;
}
public SiModelCommand getSiModelCommand() {
return this.siModelCommand;
}
public SiTest getSiTest() {
return this.siTest;
}
public void setSiTest(SiTest siTest) {
this.siTest = siTest;
}
public int getIndexInTest() {
return indexInTest;
}
public void setIndexInTest(int indexInTest) {
this.indexInTest = indexInTest;
}
public SiCommand getPairInFront() {
return this.pairCommand[0];
}
public void setPairInFront(SiCommand pairCommand) {
this.pairCommand[0] = pairCommand;
}
public SiCommand getPairInBack() {
return this.pairCommand[1];
}
public void setPairInBack(SiCommand pairCommand) {
this.pairCommand[1] = pairCommand;
}
public String getCommand() {
return this.siModelCommand.getCommand();
}
@Nullable
public WebElement getTargetElement() throws SiWebDriverException {
SiContext siContext = this.getSiTest().getSiContext();
SiCommandExecutor executor = siContext.getExecutorManager().getExecutor(this);
if (!(executor instanceof ISiCommandExecutorFlagUi)) {
return null;
}
switch (this.getCommand()) {
case "open":
case "selectWindow":
case "setWindowSize":
case "storeTitle":
case "verifyElementNotPresent":
return null;
}
String target = this.getSiModelCommand().getTarget();
if (null != target && !target.isEmpty()) {
WebElement element = siContext.asWebDriver().findElement(siContext.by(target));
return element;
}
return null;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy