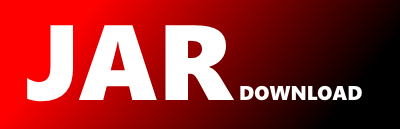
com.github.siwenyan.si.SiCommandExecutor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of side Show documentation
Show all versions of side Show documentation
Java runner of Selenium IDE project
package com.github.siwenyan.si;
import org.openqa.selenium.OutputType;
import org.openqa.selenium.TakesScreenshot;
import org.openqa.selenium.WebElement;
import java.util.HashSet;
import java.util.Set;
public abstract class SiCommandExecutor {
private Set handlersBefore;
public void beforeExecute(SiCommand command, SiContext siContext) throws SiVerifyException, SiWebDriverException {
boolean opensWindow = command.getSiModelCommand().getOpensWindow();
if (opensWindow) {
this.handlersBefore = siContext.asWebDriver().getWindowHandles();
}
if (this instanceof ISiCommandExecutorFlagUi) {
ISiCommandExecutorFlagUi executor = (ISiCommandExecutorFlagUi) this;
this.sleep(Long.parseLong(siContext.getVarValue("config.siContext.waitBeforeCommand")));
}
if ("before".equalsIgnoreCase(siContext.takingCommandScreenshots(command.getSiModelCommand()))) {
this.embedScreenshot(command, siContext, "Before command:");
}
}
public abstract void execute(SiCommand command, SiContext siContext) throws SiVerifyException, SiWebDriverException;
public void afterExecute(SiCommand command, SiContext siContext) throws SiVerifyException, SiWebDriverException {
if ("after".equalsIgnoreCase(siContext.takingCommandScreenshots(command.getSiModelCommand()))) {
this.embedScreenshot(command, siContext, "After command:");
}
if (this instanceof ISiCommandExecutorFlagUi) {
ISiCommandExecutorFlagUi executor = (ISiCommandExecutorFlagUi) this;
this.sleep(Long.parseLong(siContext.getVarValue("config.siContext.waitAfterCommand")));
}
boolean opensWindow = command.getSiModelCommand().getOpensWindow();
if (opensWindow) {
String windowHandleName = command.getSiModelCommand().getWindowHandleName();
Long windowTimeout = command.getSiModelCommand().getWindowTimeout();
long t0 = System.currentTimeMillis();
while (System.currentTimeMillis() - t0 < windowTimeout) {
Set handlersAfter = new HashSet(siContext.asWebDriver().getWindowHandles());
handlersAfter.removeAll(this.handlersBefore);
if (handlersAfter.size() == 1) {
siContext.addVar(windowHandleName, handlersAfter.iterator().next());
return;
} else if (handlersAfter.size() > 1) {
throw new SiVerifyException("Too many windows opened: " + handlersAfter.toString());
}
}
throw new SiVerifyException("Timeout waiting for new windows to open: " + command.toString());
}
}
public void embedScreenshot(SiCommand command, SiContext siContext, String description) {
if (Boolean.parseBoolean(siContext.getVarValue("config.siContext.takingCommandScreenshots"))) {
try {
WebElement element = null;
String style0 = null;
try {
element = command.getTargetElement();
style0 = element.getAttribute("style");
} catch (Exception e) {
// do nothing
}
if (null != element) {
siContext.asJavascriptExecutor().executeScript("arguments[0].setAttribute('style', arguments[1]);",
element, "color: black; background: #FDFF47; border: 2px solid red;");
}
byte[] screenshot = ((TakesScreenshot) siContext.asWebDriver()).getScreenshotAs(OutputType.BYTES);
if (null != element) {
siContext.asJavascriptExecutor().executeScript("arguments[0].setAttribute('style', arguments[1]);",
element, style0);
}
if (null != description) {
siContext.getSiReporter().write(description);
}
siContext.getSiReporter().write(siContext.getBreadcrumbTrail());
siContext.getSiReporter().write(command.toString());
siContext.getSiReporter().embed(screenshot, "image/jpeg");
} catch (Exception e) {
//do nothing
}
}
}
protected void sleep(long millis) {
try {
Thread.sleep(millis);
} catch (InterruptedException e) {
//do nothing
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy