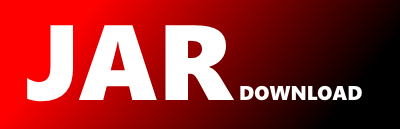
com.github.siwenyan.si.SiCommandExecutorWaitForElementVisible Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of side Show documentation
Show all versions of side Show documentation
Java runner of Selenium IDE project
package com.github.siwenyan.si;
import com.github.siwenyan.common.Sys;
import org.openqa.selenium.By;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.support.ui.ExpectedConditions;
import org.openqa.selenium.support.ui.WebDriverWait;
class SiCommandExecutorWaitForElementVisible extends SiCommandExecutor implements ISiCommandExecutorFlagUi {
@Override
public void execute(SiCommand command, SiContext siContext) throws SiVerifyException, SiWebDriverException {
By by = siContext.by(command.getSiModelCommand().getTarget());
long timeOutInMilliseconds = 10000;
try {
timeOutInMilliseconds = Integer.parseInt(command.getSiModelCommand().getValue());
} catch (Exception e) {
// do nothing
}
WebDriverWait wait = new WebDriverWait(siContext.asWebDriver(), timeOutInMilliseconds / 1000);
long lastMilliseconds = System.currentTimeMillis() + timeOutInMilliseconds;
while (System.currentTimeMillis() < lastMilliseconds) {
try {
wait.until(ExpectedConditions.visibilityOfElementLocated(by));
WebElement element = siContext.asWebDriver().findElement(by);
if (element.isDisplayed()) {
return;
}
} catch (Exception e) {
//do nothing
}
Sys.sleep(1000);
}
throw new RuntimeException("Time out looking for " + by.toString() + ".");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy