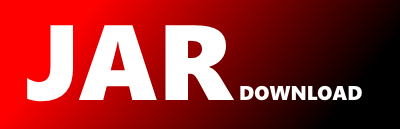
com.github.siwenyan.si.SiContextMonitor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of side Show documentation
Show all versions of side Show documentation
Java runner of Selenium IDE project
package com.github.siwenyan.si;
import com.github.siwenyan.common.Reporter;
import com.github.siwenyan.common.QuickJson;
import com.github.siwenyan.common.Sys;
import org.apache.commons.io.FileUtils;
import org.jetbrains.annotations.Nullable;
import java.io.File;
import java.util.HashMap;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
public class SiContextMonitor {
protected final Map>> specsByEventName = new HashMap>>();
public SiContextMonitor(List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy