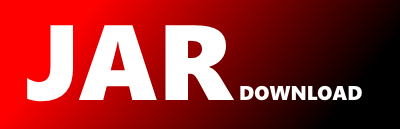
com.github.siwenyan.si.SiModelProvider Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of side Show documentation
Show all versions of side Show documentation
Java runner of Selenium IDE project
package com.github.siwenyan.si;
import com.github.siwenyan.common.QuickJson;
import com.github.siwenyan.common.Reporter;
import com.github.siwenyan.common.DateTimeUtils;
import com.github.siwenyan.common.Sys;
import com.github.siwenyan.si.model.SiModel;
import com.github.siwenyan.si.model.SiModelCommand;
import com.github.siwenyan.si.model.SiModelSuite;
import com.github.siwenyan.si.model.SiModelTest;
import com.thoughtworks.xstream.XStream;
import org.apache.commons.io.FileUtils;
import org.jetbrains.annotations.Nullable;
import java.io.*;
import java.util.*;
public class SiModelProvider {
private final Map siModelByName = new HashMap();
private static XStream xstream;
private static XStream getXStream() {
if (null == xstream) {
xstream = new XStream();
}
return xstream;
}
/***
*
* usage: split a SIDE project file into SIDE test files
* $ java SiModelProvider split target/output/saveAs.side target/output/saveAs
*
* usage: build a SIDE project by scanning all SIDE tests in a directory recursively.
* $ java SiModelProvider build * target/output/saveAs.side http://example.com/ src/main/resources/side src/main/resources/side/common/tests
*
* usage: build a SIDE project file to execute a specific SIDE test.
* All dependencies must be included into the same SIDE project by scanning all tests within a folder recursively
* $ java SiModelProvider build demo target/output/saveAs.side http://example.com/ src/main/resources/side src/main/resources/side/common/tests
*
* @param args
* args[0]=[split|build]
*
* when args[0]=split
* args[1]=side project file full path
* args[2]=target folder
*
* when args[0]=build
* args[1]=the target SIDE test or * to include all SIDE tests
* args[2]=the SIDE project file to be generated
* args[3]=the SIDE project base url
* args[4...]=the folders containing all the tests
*
* @throws IOException for file reading and writing
* @throws RuntimeException for json parsing and syntax checking
*
*/
public static void main(String[] args) throws IOException {
String opt = args[0];
switch (opt) {
case "split":
String sideFileName = args[1].trim();
String targetFolderName = args[2].trim();
while (targetFolderName.endsWith("/") || targetFolderName.endsWith("\\")) {
targetFolderName = targetFolderName.substring(0, targetFolderName.length() - 1).trim();
}
targetFolderName += "_" + DateTimeUtils.timestamp();
File targetFolder = Sys.findFile(targetFolderName);
if (null != targetFolder) {
if (targetFolder.isFile() || targetFolder.isDirectory() && targetFolder.listFiles().length > 0) {
throw new RuntimeException("Not a directory or the directory is not empty: " + targetFolderName);
}
} else {
FileUtils.forceMkdir(new File(targetFolderName));
}
SiModel siModel = buildSiModel(sideFileName);
assignSuites(siModel);
for (SiModelTest test : siModel.getTests()) {
String path = test.getSuite();
if (null == path || path.isEmpty()) {
path = File.separator;
} else {
path = File.separator + path.replace('.', File.separatorChar) + File.separator;
}
String testName = test.getName();
String jsonTest = toJsonString(test);
jsonTest = QuickJson.prettyJson(jsonTest);
File jsonTestFile = new File(targetFolderName + path + testName + ".json");
FileUtils.writeStringToFile(jsonTestFile, jsonTest);
}
break;
case "build":
String testName = args[1];
String saveAs = args[2];
String url = args[3];
String[] homes = Arrays.copyOfRange(args, 4, args.length);
SiModel siModel1 = buildSiModel(url, homes);
if (!"*".equals(testName)) {
siModel1 = siModel1.extractSiModelForTest(testName);
}
String jsonModel = toJsonString(siModel1);
jsonModel = QuickJson.prettyJson(jsonModel);
System.out.println(jsonModel);
FileUtils.writeStringToFile(new File(saveAs), jsonModel);
break;
default:
throw new RuntimeException("Unsupported option: " + opt);
}
}
private static void assignSuites(SiModel siModel) {
Map testByIds = new HashMap();
for (SiModelTest siModelTest : siModel.getTests()) {
testByIds.put(siModelTest.getId(), siModelTest);
}
for (SiModelSuite siModelSuite : siModel.getSuites()) {
for (String id : siModelSuite.getTests()) {
testByIds.get(id).setSuite(siModelSuite.getName());
}
}
}
public static SiModelTest randomSiModelTest(SiModelCommand... siModelCommands) {
return randomSiModelTest(Arrays.asList(siModelCommands));
}
public static SiModelTest randomSiModelTest(List siModelCommands) {
SiModelTest siModelTest = new SiModelTest();
siModelTest.setName(Reporter.getTimestamp());
for (SiModelCommand siModelCommand : siModelCommands) {
siModelTest.getCommands().add(siModelCommand);
}
return siModelTest;
}
//option #1
// {
// "id": "7e5b6270-fd6a-4f69-86b7-18626fa5727a",
// "comment": "",
// "command": "run",
// "target": "request summary",
// "targets": [],
// "value": ""
// }
//
//option #2
// command::target[::value]
public static SiModelCommand buildSiModelCommand(String s) {
SiModelCommand siModelCommand = null;
try {
siModelCommand = (SiModelCommand) QuickJson.readValue(s, SiModelCommand.class);
} catch (Exception e) {
String[] p = s.split("::");
siModelCommand = new SiModelCommand();
siModelCommand.setCommand(p[0]);
siModelCommand.setTarget(p[1]);
if (p.length > 2) {
siModelCommand.setValue(p[2]);
}
}
return siModelCommand;
}
public static SiModelCommand buildSiModelCommand(Map spec) {
Properties props = new Properties();
props.putAll(spec);
SiModelCommand siModelCommand = new SiModelCommand();
siModelCommand.setCommand(props.getProperty("Command"));
siModelCommand.setTarget(props.getProperty("Target", props.getProperty("Param1")));
if (props.containsKey("Value") || props.containsKey("Param2")) {
siModelCommand.setValue(props.getProperty("Value", props.getProperty("Param2")));
}
return siModelCommand;
}
@Nullable
public SiModel getSiModelByName(String name) {
return this.siModelByName.get(name);
}
public void setSiModelByName(String name, SiModel siModel) {
this.siModelByName.put(name, siModel);
}
@Nullable
public static SiModel buildSiModel(String modelSource) {
try {
String s = null;
File sideFile = Sys.findFile(modelSource);
if(null==sideFile){
s = modelSource;
}else{
try{
s = FileUtils.readFileToString(sideFile);}catch (Exception e){
s= modelSource;
}
}
SiModel siModel = SiModelProvider.buildSiModelByJsonString(s);
return siModel;
} catch (Exception e) {
throw new RuntimeException(e);
}
}
@Nullable
public static SiModel buildSiModelByJsonString(String s) {
SiModel value = null;
try {
value = (SiModel)QuickJson.readValue(s, SiModel.class);
return value;
} catch (Exception e) {
throw new RuntimeException(e);
}
}
@Nullable
public static SiModel buildSiModel(String url, String... homes) {
SiModel siModel = new SiModel();
siModel.setName("All");
siModel.setUrl(url);
Map allTests = new HashMap();
for (String home : homes) {
allTests.putAll(scanSiModelTest(home));
}
for (SiModelTest siModelTest : allTests.values()) {
siModel.getTests().add(siModelTest);
}
siModel.createSuites();
siModel.reIndex();
return siModel;
}
private static Map> allTestsByHome = new HashMap>();
public static Map scanSiModelTest(String home) {
if (!allTestsByHome.containsKey(home)) {
Map allTests = new HashMap();
File homeDir = Sys.findFile(home);
Collection files = FileUtils.listFiles(homeDir, new String[]{"json"}, true);
for (File file : files) {
try {
String suite = calculateSuiteNameByFilePath(file, home);
String s = FileUtils.readFileToString(file);
SiModelTest siModelTest = (SiModelTest)QuickJson.readValue(s, SiModelTest.class);
siModelTest.setSuite(suite);
allTests.put(siModelTest.getName(), siModelTest);
} catch (Exception e) {
e.printStackTrace();
//do nothing
}
}
allTestsByHome.put(home, allTests);
}
return allTestsByHome.get(home);
}
private static String calculateSuiteNameByFilePath(File file, String home) {
try {
String path = file.getPath();
path = path.split(home, 2)[1];
path = path.substring(home.length() + 1, path.length() - file.getName().length() - 1);
path = path.replace(File.separatorChar, '.');
return path;
} catch (Exception e) {
return "";
}
}
@Nullable
public static String toJsonString(SiModel siModel) {
try {
return QuickJson.prettyJson(siModel);
} catch (Exception e) {
throw new RuntimeException(e);
}
}
@Nullable
public static String toJsonString(SiModelTest siModelTest) {
try {
return QuickJson.prettyJson(siModelTest);
} catch (Exception e) {
throw new RuntimeException(e);
}
}
@Nullable
public static String toXmlString(SiModel siModel) {
try {
String xml = getXStream().toXML(siModel);
return xml;
} catch (Exception e) {
throw new RuntimeException(e);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy