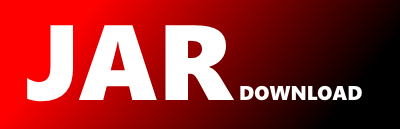
com.github.siwenyan.si.SiTest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of side Show documentation
Show all versions of side Show documentation
Java runner of Selenium IDE project
package com.github.siwenyan.si;
import com.github.siwenyan.common.Reporter;
import com.github.siwenyan.common.Sys;
import com.github.siwenyan.si.model.SiModelCommand;
import com.github.siwenyan.si.model.SiModelTest;
import org.apache.log4j.Logger;
import org.openqa.selenium.NoSuchWindowException;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import java.util.Stack;
public class SiTest {
private final static Logger LOGGER = Logger.getLogger(SiTest.class.getName());
private final SiModelTest siModelTest;
private List commandList = new ArrayList();
private SiContext siContext;
private int cursor;
private int size;
public SiTest(SiModelTest siModelTest, SiContext siContext) {
this.siModelTest = siModelTest;
this.siContext = siContext;
init();
}
private void init() {
int cmdIndex = 0; // zero based index because it's used to set the cursor
Stack tracking = new Stack();
for (SiModelCommand cmdM : this.siModelTest.getCommands()) {
SiCommand cmd = SiCommand.createSiCommand(cmdM, this);
this.commandList.add(cmd);
cmd.setIndexInTest(cmdIndex++);
switch (cmd.getCommand()) {
case "times":
tracking.push(cmd);
break;
case "while":
tracking.push(cmd);
break;
case "if":
tracking.push(cmd);
break;
case "else":
SiCommand popIf = tracking.pop();
if (!"if".equals(popIf.getSiModelCommand().getCommand())) {
throw new RuntimeException(cmd.toString() + " missing if in test: " + this.siModelTest.toString());
}
popIf.setPairInBack(cmd);
cmd.setPairInFront(popIf);
tracking.push(cmd);
break;
case "end":
SiCommand popBegin = tracking.pop();
switch (popBegin.getSiModelCommand().getCommand()) {
case "times":
case "while":
case "if":
case "else":
break;
default:
throw new RuntimeException(cmd.toString() + " missing begin (times, while, if or else) in test: " + this.siModelTest.toString());
}
popBegin.setPairInBack(cmd);
cmd.setPairInFront(popBegin);
break;
case "do":
tracking.push(cmd);
break;
case "repeatIf":
SiCommand popDo = tracking.pop();
if (!"do".equals(popDo.getSiModelCommand().getCommand())) {
throw new RuntimeException(cmd.toString() + " missing do in test: " + this.siModelTest.toString());
}
popDo.setPairInBack(cmd);
cmd.setPairInFront(popDo);
break;
default:
// do nothing
}
}
if (tracking.size() > 0) {
throw new RuntimeException(this.siModelTest.toString() + " missing ending for commands: " + tracking.toString());
}
this.size = this.commandList.size();
}
@Override
public String toString() {
return this.getSiModelTest().toString();
}
public boolean hasNextCommand() {
return this.cursor < this.size;
}
public SiCommand nextCommand() {
return this.commandList.get(this.cursor++);
}
public void run() {
this.run(0, null, null);
}
public void run(int retry) {
this.run(retry, null, null);
}
public void run(int retry, List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy