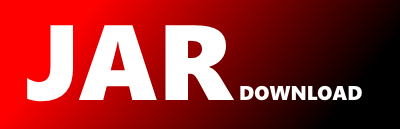
com.github.siwenyan.si.SiUiSelect Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of side Show documentation
Show all versions of side Show documentation
Java runner of Selenium IDE project
package com.github.siwenyan.si;
import org.openqa.selenium.*;
import org.openqa.selenium.support.ui.Select;
public class SiUiSelect {
private final WebElement element;
private final Select select;
private final SiContext siContext;
public SiUiSelect(WebElement element, SiContext siContext) {
this.siContext = siContext;
this.element = element;
this.select = new Select(element);
}
public void select(String optionExpression) throws SiWebDriverException {
if (!optionExpression.contains("=")) {
optionExpression = "label=" + optionExpression;
}
String[] p = optionExpression.split("=", 2);
try {
switch (p[0]) {
case "label":
this.select.selectByVisibleText(p[1]);
break;
case "value":
this.select.selectByValue(p[1]);
break;
case "index":
this.select.selectByIndex(Integer.parseInt(p[1]));
break;
default:
throw new RuntimeException("Unsupported option type: " + p[0]);
}
} catch (ElementNotInteractableException e) {
siContext.getReporter().reportMessage("Using javascript...");
String jsf = "";
jsf += "var theElement = document.getElementById('%s'); ";
jsf += "var theOptions = theElement.getElementsByTagName('option'); ";
jsf += "for(var i = 0; i < theOptions.length; i++) { ";
jsf += " if(%s) { ";
jsf += " theOptions[i].selected = 'selected'; ";
jsf += " break; ";
jsf += " } ";
jsf += "} ";
jsf += "theElement.onchange(); ";
String id = element.getAttribute("id");
String condition = "";
switch (p[0]) {
case "label":
condition = "'" + p[1] + "' == theOptions[i].text";
break;
case "value":
condition = "'" + p[1] + "' == theOptions[i].getAttribute('value')";
break;
case "index":
condition = Integer.parseInt(p[1]) + " == i";
break;
default:
throw new RuntimeException("Unsupported option type: " + p[0]);
}
String js = String.format(jsf, id, condition);
this.siContext.asJavascriptExecutor().executeScript(js);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy