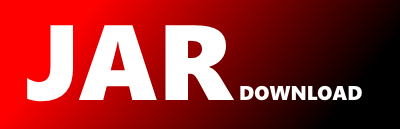
com.github.siwenyan.si.SiUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of side Show documentation
Show all versions of side Show documentation
Java runner of Selenium IDE project
package com.github.siwenyan.si;
import com.github.siwenyan.si.model.SiModel;
import com.github.siwenyan.si.model.SiModelCommand;
import com.github.siwenyan.si.model.SiModelTest;
import java.util.*;
public class SiUtils {
public static void extractVariablesByValues(SiModel siModel, Map varNamesByValues) {
Set shellTests = new HashSet();
for (SiModelTest siModelTest : siModel.getTests()) {
Set valuesAppearedInTest = new HashSet();
for (SiModelCommand siModelCommand : siModelTest.getCommands()) {
String commandValue = siModelCommand.getValue();
if (!commandValue.isEmpty()) {
for (String value : varNamesByValues.keySet()) {
if (commandValue.equals(value)) {
valuesAppearedInTest.add(value);
commandValue = commandValue.replace(value, "${" + varNamesByValues.get(value) + "}");
siModelCommand.setValue(commandValue);
}
}
}
String commandTarget = siModelCommand.getTarget();
if (!commandTarget.isEmpty()) {
for (String value : varNamesByValues.keySet()) {
if (commandTarget.equals(value)) {
valuesAppearedInTest.add(value);
commandTarget = commandTarget.replace(value, "${" + varNamesByValues.get(value) + "}");
siModelCommand.setTarget(commandTarget);
}
}
}
}
if (valuesAppearedInTest.size() > 0) {
SiModelTest shellTest = new SiModelTest();
shellTest.setName(siModelTest.getName() + "_shell");
shellTest.setSuite(siModelTest.getSuite());
for (String value : valuesAppearedInTest) {
String name = varNamesByValues.get(value);
SiModelCommand storeCommand = new SiModelCommand();
storeCommand.setCommand("store");
storeCommand.setTarget(value);
storeCommand.setValue(name);
shellTest.getCommands().add(storeCommand);
}
SiModelCommand runCommand = new SiModelCommand();
runCommand.setCommand("run");
runCommand.setTarget(siModelTest.getName());
shellTest.getCommands().add(runCommand);
shellTests.add(shellTest);
}
}
for (SiModelTest shellTest : shellTests) {
siModel.getTests().add(shellTest);
}
}
public static Map namesByValues(List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy