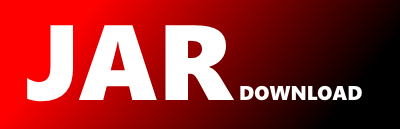
com.github.siwenyan.si.model.SiModel Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of side Show documentation
Show all versions of side Show documentation
Java runner of Selenium IDE project
package com.github.siwenyan.si.model;
import com.fasterxml.jackson.annotation.JsonIgnore;
import org.jetbrains.annotations.Nullable;
import java.util.*;
public class SiModel {
public static final String CONFIG_PREFIX = "config.";
private String id = UUID.randomUUID().toString();
private String version = "2.0";
private String name = "";
private String url = "";
private List tests = new ArrayList();
private List suites = new ArrayList();
private List urls = new ArrayList();
private List plugins = new ArrayList();
@JsonIgnore
private Map testById = new HashMap();
@JsonIgnore
private Map testByName = new HashMap();
@JsonIgnore
private Map suiteByName = new HashMap();
@JsonIgnore
private Map> locatorNamesBylocators = new HashMap>();
@JsonIgnore
private Set variableNameSet = new LinkedHashSet<>();
public SiModel() {
}
public SiModel(SiModel siModel) {
this.name = siModel.getName();
this.url = siModel.getUrl();
this.tests.addAll(siModel.getTests());
this.suites.addAll(siModel.getSuites());
this.urls.addAll(siModel.getUrls());
this.plugins.addAll(siModel.getPlugins());
}
@Override
public String toString() {
return this.name + "." + this.version;
}
public String getId() {
return this.id;
}
public void setId(String id) {
this.id = id;
}
public String getVersion() {
return this.version;
}
public void setVersion(String version) {
this.version = version;
}
public String getName() {
return this.name;
}
public void setName(String name) {
this.name = name;
}
public String getUrl() {
return this.url;
}
public void setUrl(String url) {
this.url = url;
}
public List getTests() {
return this.tests;
}
public void setTests(List tests) {
this.tests = tests;
}
public List getSuites() {
return this.suites;
}
public void setSuites(List suites) {
this.suites = suites;
}
public List getUrls() {
return this.urls;
}
public void setUrls(List urls) {
this.urls = urls;
}
public List getPlugins() {
return this.plugins;
}
public void setPlugins(List plugins) {
this.plugins = plugins;
}
public Map> getLocatorNamesBylocators() {
return locatorNamesBylocators;
}
public void setLocatorNamesBylocators(Map> locatorNamesBylocators) {
this.locatorNamesBylocators = locatorNamesBylocators;
}
public void reIndex() {
this.testById = new HashMap();
this.testByName = new HashMap();
this.suiteByName = new HashMap();
for (SiModelTest siModelTest : this.getTests()) {
this.testById.put(siModelTest.getId(), siModelTest);
this.testByName.put(siModelTest.getName(), siModelTest);
}
for (SiModelSuite siModelSuite : this.getSuites()) {
this.suiteByName.put(siModelSuite.getName(), siModelSuite);
}
}
public SiModelTest getSiModelTestById(String key) {
return this.testById.get(key);
}
public SiModelTest getSiModelTestByName(String key) {
return this.testByName.get(key);
}
public List getSiModelSuiteListBySuiteNameRegex(String regex) {
List list = new ArrayList();
for (String key : this.suiteByName.keySet()) {
if (key.matches(regex)) {
list.add(this.suiteByName.get(key));
}
}
return list;
}
public void clearTargets() {
for (SiModelTest siModelTest : this.getTests()) {
for (SiModelCommand siModelCommand : siModelTest.getCommands()) {
siModelCommand.getTargets().clear();
}
}
}
public void resetTargets(String cmdRegex, String tgtRegex, String suiteRegex) {
try {
for (SiModelTest siModelTest : this.getTests()) {
for (SiModelCommand siModelCommand : siModelTest.getCommands()) {
String command = siModelCommand.getCommand();
String target = siModelCommand.getTarget();
if (command.matches(cmdRegex) && target.matches(tgtRegex)) {
for (String name : this.suiteByName.keySet()) {
if (name.matches(suiteRegex)) {
SiModelSuite siModelSuite = this.suiteByName.get(name);
for (String testId : siModelSuite.getTests()) {
String testName = this.testById.get(testId).getName();
siModelCommand.getTargets().add(Arrays.asList(new String[]{testName, ""}));
siModelCommand.setComment(siModelCommand.getComment() + testName + ", ");
}
}
}
}
}
}
} catch (Exception e) {
// do nothing
}
}
public void createSuites() {
Map> testIdsBySuiteName = new HashMap>();
for (SiModelTest siModelTest : this.getTests()) {
String suite = siModelTest.getSuite();
if (null == suite || suite.isEmpty()) {
continue;
}
if (!testIdsBySuiteName.containsKey(suite)) {
testIdsBySuiteName.put(suite, new LinkedList());
}
testIdsBySuiteName.get(suite).add(siModelTest.getId());
}
Map existingSuitesByName = new HashMap();
for (SiModelSuite siModelSuite : this.getSuites()) {
existingSuitesByName.put(siModelSuite.getName(), siModelSuite);
}
for (String suiteName : testIdsBySuiteName.keySet()) {
if (existingSuitesByName.containsKey(suiteName)) {
for (String testId : testIdsBySuiteName.get(suiteName)) {
if (!existingSuitesByName.get(suiteName).getTests().contains(testId)) {
existingSuitesByName.get(suiteName).getTests().add(testId);
}
}
} else {
SiModelSuite siModelSuite = new SiModelSuite();
siModelSuite.setName(suiteName);
siModelSuite.setTests(testIdsBySuiteName.get(suiteName));
this.getSuites().add(siModelSuite);
}
}
}
public void syntaxCheck() {
variableNameSet.clear();
//UUID must be unique
Map modelsById = new HashMap();
modelsById.put(this.getId(), this);
for (SiModelTest siModelTest : this.getTests()) {
String siModelTestId = siModelTest.getId();
if (modelsById.containsKey(siModelTestId)) {
throw new RuntimeException(
"Suggested SiModelTest UUID [" + UUID.randomUUID().toString() + "] for ["
+ siModelTest.toString()
+ "] to resolve the conflict with ["
+ modelsById.get(siModelTestId).toString() + "]");
} else {
modelsById.put(siModelTestId, siModelTest);
}
for (SiModelCommand siModelCommand : siModelTest.getCommands()) {
String siModelCommandId = siModelCommand.getId();
if (modelsById.containsKey(siModelCommandId)) {
throw new RuntimeException(
"Suggested SiModelCommand UUID [" + UUID.randomUUID().toString() + "] for ["
+ siModelCommand.toString() + "] in test [" + siModelTest.toString()
+ "] to resolve the conflict with ["
+ modelsById.get(siModelCommandId).toString() + "]");
} else {
modelsById.put(siModelCommandId, siModelCommand);
}
}
}
//Test name must be unique
Map testByName = new HashMap();
for (SiModelTest test : this.getTests()) {
String testName = test.getName();
if (testByName.containsKey(test.getName())) {
throw new RuntimeException("Duplicated test name: [" + testName + "]");
}
testByName.put(test.getName(), test);
}
//Command "run" must have a valid test name as target
for (SiModelTest test : this.getTests()) {
for (SiModelCommand siModelCommand : test.getCommands()) {
if ("run".equals(siModelCommand.getCommand())) {
String target = siModelCommand.getTarget();
if (!testByName.containsKey(target)) {
throw new RuntimeException("No such test: [" + target + "] in test [" + test.toString() + "]");
}
}
}
}
}
@Nullable
public SiModel extractSiModelForTest(String testName) {
SiModelTest rootTest = this.getSiModelTestByName(testName);
if (null == rootTest) {
return null;
}
SiModel siModel = new SiModel();
siModel.setName("Extracted for " + rootTest.toString());
siModel.setUrl(this.getUrl());
Queue testQueue = new LinkedList<>();
testQueue.add(rootTest);
while (testQueue.size() > 0) {
SiModelTest currTest = testQueue.poll();
siModel.getTests().add(currTest);
for (SiModelCommand cmd : currTest.getCommands()) {
if ("run".equals(cmd.getCommand())) {
SiModelTest requiredSiModelTest = this.getSiModelTestByName(cmd.getTarget());
if (null == requiredSiModelTest) {
throw new RuntimeException("No such test: [" + cmd.toString() + "] which is required by test [" + currTest.toString() + "]");
} else {
testQueue.add(requiredSiModelTest);
}
}
}
}
siModel.createSuites();
siModel.reIndex();
return siModel;
}
public void appendTests(Set tests) {
this.getTests().addAll(tests);
this.createSuites();
}
public void appendTest(SiModelTest test) {
this.getTests().add(test);
this.createSuites();
}
public void appendConfig(String suiteName, String testName, Properties properties) {
//extract all variable names
this.variableNameSet.clear();
for (SiModelTest test : this.getTests()) {
for (SiModelCommand siModelCommand : test.getCommands()) {
variableNameSet.addAll(VarExpression.extractVariableNames(siModelCommand.getTarget()));
variableNameSet.addAll(VarExpression.extractVariableNames(siModelCommand.getValue()));
}
}
SiModelTest loadConfig = new SiModelTest();
loadConfig.setSuite(suiteName);
testName = null == testName || testName.trim().isEmpty() ? loadConfig.getId() : testName;
loadConfig.setName(testName);
for (String key : this.variableNameSet) {
if (key.startsWith(CONFIG_PREFIX)) {
String key1 = key.substring(CONFIG_PREFIX.length());
String value = properties.getProperty(key1);
if (null == value) {
value = "";
}
value = value.trim();
SiModelCommand siModelCommand = new SiModelCommand();
siModelCommand.setCommand("store");
siModelCommand.setTarget(value);
siModelCommand.setValue(key);
loadConfig.getCommands().add(siModelCommand);
}
}
this.appendTest(loadConfig);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy