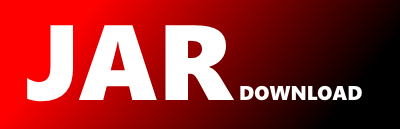
com.github.siwenyan.si.spy.SpyWebDriver Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of side Show documentation
Show all versions of side Show documentation
Java runner of Selenium IDE project
package com.github.siwenyan.si.spy;
import com.github.siwenyan.si.model.SiModelCommand;
import org.apache.commons.io.FileUtils;
import org.openqa.selenium.*;
import org.openqa.selenium.html5.*;
import org.openqa.selenium.interactions.*;
import org.openqa.selenium.internal.*;
import org.openqa.selenium.mobile.NetworkConnection;
import java.io.File;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import java.util.Set;
public class SpyWebDriver implements WebDriver, JavascriptExecutor, FindsById, FindsByClassName, FindsByLinkText,
FindsByName, FindsByCssSelector, FindsByTagName, FindsByXPath, HasInputDevices, HasCapabilities, Interactive,
TakesScreenshot, LocationContext, WebStorage, HasTouchScreen, NetworkConnection {
private SiSpy siSpy = new SiSpy();
private final WebDriver webDriver;
private String output;
public SpyWebDriver(WebDriver webDriver) {
this.webDriver = webDriver;
}
@Override
public void get(String url) {
webDriver.get(url);
SiModelCommand c = new SiModelCommand();
c.setCommand("open");
c.setTarget(url);
siSpy.appendCommand(c);
siSpy.getRecordedModel().setUrl(url);
}
@Override
public String getCurrentUrl() {
return webDriver.getCurrentUrl();
}
@Override
public String getTitle() {
return webDriver.getTitle();
}
@Override
public List findElements(By by) {
List els = webDriver.findElements(by);
List spyEls = new ArrayList<>(els.size());
for (WebElement el : els) {
spyEls.add(new SpyWebElement(this, el, by, null));
}
return spyEls;
}
@Override
public WebElement findElement(By by) {
WebElement el = webDriver.findElement(by);
return new SpyWebElement(this, el, by, null);
}
@Override
public String getPageSource() {
return webDriver.getPageSource();
}
@Override
public void close() {
webDriver.close();
}
@Override
public void quit() {
try {
String fn = "Spy" + System.currentTimeMillis() + ".side";
String s = this.getSiSpy().toString();
System.out.println(fn + "\r\n\t" + s);
if (null != this.getOutput()) {
FileUtils.writeStringToFile(new File(this.getOutput() + fn), s);
}
} catch (Exception e) {
e.printStackTrace();
} finally {
webDriver.quit();
}
}
@Override
public Set getWindowHandles() {
return webDriver.getWindowHandles();
}
@Override
public String getWindowHandle() {
return webDriver.getWindowHandle();
}
@Override
public TargetLocator switchTo() {
return webDriver.switchTo();
}
@Override
public Navigation navigate() {
return webDriver.navigate();
}
@Override
public Options manage() {
return webDriver.manage();
}
@Override
public Object executeScript(String script, Object... args) {
for (int i = 0; i < args.length; i++) {
if (args[i] instanceof SpyWebElement) {
args[i] = ((SpyWebElement) args[i]).el;
}
}
Object o = ((JavascriptExecutor) webDriver).executeScript(script, args);
return o;
}
@Override
public Object executeAsyncScript(String script, Object... args) {
for (int i = 0; i < args.length; i++) {
if (args[i] instanceof SpyWebElement) {
args[i] = ((SpyWebElement) args[i]).el;
}
}
Object o = ((JavascriptExecutor) webDriver).executeAsyncScript(script, args);
return o;
}
public SiSpy getSiSpy() {
return this.siSpy;
}
public String getOutput() {
return output;
}
public void setOutput(String output) {
this.output = output;
}
@Override
public WebElement findElementById(String using) {
return ((FindsById) webDriver).findElementById(using);
}
@Override
public List findElementsById(String using) {
return ((FindsById) webDriver).findElementsById(using);
}
@Override
public WebElement findElementByClassName(String using) {
return ((FindsByClassName) webDriver).findElementByClassName(using);
}
@Override
public List findElementsByClassName(String using) {
return ((FindsByClassName) webDriver).findElementsByClassName(using);
}
@Override
public WebElement findElementByLinkText(String using) {
return ((FindsByLinkText) webDriver).findElementByLinkText(using);
}
@Override
public List findElementsByLinkText(String using) {
return ((FindsByLinkText) webDriver).findElementsByLinkText(using);
}
@Override
public WebElement findElementByPartialLinkText(String using) {
return ((FindsByLinkText) webDriver).findElementByPartialLinkText(using);
}
@Override
public List findElementsByPartialLinkText(String using) {
return ((FindsByLinkText) webDriver).findElementsByPartialLinkText(using);
}
@Override
public WebElement findElementByName(String using) {
return ((FindsByName) webDriver).findElementByName(using);
}
@Override
public List findElementsByName(String using) {
return ((FindsByName) webDriver).findElementsByName(using);
}
@Override
public WebElement findElementByCssSelector(String using) {
return ((FindsByCssSelector) webDriver).findElementByCssSelector(using);
}
@Override
public List findElementsByCssSelector(String using) {
return ((FindsByCssSelector) webDriver).findElementsByCssSelector(using);
}
@Override
public WebElement findElementByTagName(String using) {
return ((FindsByTagName) webDriver).findElementByTagName(using);
}
@Override
public List findElementsByTagName(String using) {
return ((FindsByTagName) webDriver).findElementsByTagName(using);
}
@Override
public WebElement findElementByXPath(String using) {
return ((FindsByXPath) webDriver).findElementByXPath(using);
}
@Override
public List findElementsByXPath(String using) {
return ((FindsByXPath) webDriver).findElementsByXPath(using);
}
@Override
public Keyboard getKeyboard() {
return ((HasInputDevices) webDriver).getKeyboard();
}
@Override
public Mouse getMouse() {
return ((HasInputDevices) webDriver).getMouse();
}
@Override
public Capabilities getCapabilities() {
return ((HasCapabilities) webDriver).getCapabilities();
}
@Override
public void perform(Collection actions) {
((Interactive) webDriver).perform(actions);
}
@Override
public void resetInputState() {
((Interactive) webDriver).resetInputState();
}
@Override
public X getScreenshotAs(OutputType target) throws WebDriverException {
return ((TakesScreenshot) webDriver).getScreenshotAs(target);
}
@Override
public Location location() {
return ((LocationContext) webDriver).location();
}
@Override
public void setLocation(Location location) {
((LocationContext) webDriver).setLocation(location);
}
@Override
public LocalStorage getLocalStorage() {
return ((WebStorage) webDriver).getLocalStorage();
}
@Override
public SessionStorage getSessionStorage() {
return ((WebStorage) webDriver).getSessionStorage();
}
@Override
public TouchScreen getTouch() {
return ((HasTouchScreen) webDriver).getTouch();
}
@Override
public ConnectionType getNetworkConnection() {
return ((NetworkConnection) webDriver).getNetworkConnection();
}
@Override
public ConnectionType setNetworkConnection(ConnectionType type) {
return ((NetworkConnection) webDriver).setNetworkConnection(type);
}
public WebDriver adaptee() {
return webDriver;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy