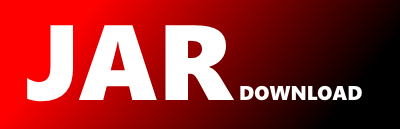
dswork.ee.MyTomcat Maven / Gradle / Ivy
The newest version!
package dswork.ee;
import java.io.File;
import java.util.Map;
import org.apache.catalina.LifecycleListener;
import org.apache.catalina.connector.Connector;
import org.apache.catalina.startup.Tomcat;
public class MyTomcat
{
private Tomcat tomcat = new Tomcat();
private int port = 8080;
private String hostname = "localhost";
private String baseDir = "/WorkServer/TomcatEmbed";
private java.io.File contextDir = null;
private java.util.Map map = new java.util.LinkedHashMap();
public MyTomcat()
{
}
private static String loaderPath = "";
private static java.io.File loaderFile = null;
static
{
java.net.URL url = Thread.currentThread().getContextClassLoader().getResource("");
String loadpath = url == null ? System.getProperty("user.dir") + "/" : url.getPath();
loadpath = String.valueOf(loadpath).replace('\\', '/').replace("//", "/");
if(loadpath.endsWith("/"))
{
loadpath = loadpath.substring(0, loadpath.length() - 1);
}
loaderPath = loadpath;
loaderFile = new java.io.File(loaderPath);
System.out.println("LoaderPath = " + loaderPath);
}
public static String getLoaderPath()
{
return loaderPath;
}
private static String projectPath = null;
private static String webPath = null;
public static void initPath()
{
if(projectPath == null || webPath == null)
{
String _project;
String _web = null;
if(loaderPath.endsWith("/src/main/webapp/WEB-INF/classes"))
{
_project = loaderFile.getParentFile().getParentFile().getParentFile().getParentFile().getParentFile().getPath().replace('\\', '/');
_web = loaderFile.getParentFile().getParentFile().getPath().replace('\\', '/');
}
else if(loaderPath.endsWith("/web/WEB-INF/classes"))
{
_project = loaderFile.getParentFile().getParentFile().getParentFile().getPath().replace('\\', '/');
_web = loaderFile.getParentFile().getParentFile().getPath().replace('\\', '/');
}
else if(loaderPath.endsWith("/target/classes"))
{
// 这里就有多种说法了,是使用/src/main/webapp呢?还是使用/target/classes/META-INF/resources
_project = loaderFile.getParentFile().getParentFile().getPath().replace('\\', '/');
}
else if(loaderPath.endsWith("/bin"))
{
_project = loaderFile.getParentFile().getPath().replace('\\', '/');
}
else
{
_project = loaderFile.getPath().replace('\\', '/');
}
if(_web == null)
{
_web = _project + "/src/main/webapp";
if(!(new File(_web)).isDirectory())
{
_web = _project + "/web";
if(!(new File(_web)).isDirectory())
{
_web = _project + "/target/classes/META-INF/resources";
if(!(new File(_web)).isDirectory())
{
_web = _project;// 实在找不到web目录了,使用项目目录吧
}
}
}
}
projectPath = _project;
webPath = _web;
}
}
public static String getProjectPath()
{
initPath();
return projectPath;
}
public static String getProjectName()
{
File f = new File(getProjectPath());
return f.getName();
}
public static String getWebPath()
{
initPath();
return webPath;
}
public MyTomcat addWebapp() throws Exception
{
map.put("/" + getProjectName(), getWebPath());
return this;
}
public MyTomcat addWebapp(String projectName) throws Exception
{
map.put(projectName, getWebPath());
return this;
}
public MyTomcat addWebapp(String projectName, String projectPath) throws Exception
{
map.put(projectName, (new java.io.File(String.valueOf(projectPath).replace('\\', '/').replace("//", "/"))).getPath().replace('\\', '/'));
return this;
}
public MyTomcat setPort(int port)
{
this.port = port;
return this;
}
public MyTomcat setHostname(String hostname)
{
this.hostname = hostname;
return this;
}
public MyTomcat setBaseDir(String baseDir)
{
this.baseDir = baseDir;
return this;
}
public MyTomcat setContextDir(String contextDir)
{
File f = new File(loaderPath + "/" + contextDir);
if(f.isFile())
{
this.contextDir = f;
}
else
{
this.contextDir = new File(contextDir);
}
return this;
}
public void start() throws Exception
{
start(true);
}
public void start(boolean await) throws Exception
{
tomcat.setPort(port);
tomcat.setBaseDir(baseDir);
tomcat.setHostname(hostname);
org.apache.juli.ClassLoaderLogManager.getLoggingMXBean().setLoggerLevel("", "ALL");
//tomcat.getServer().setParentClassLoader(Thread.currentThread().getContextClassLoader());
System.err.println("Webapp Loading");
for(Map.Entry x : map.entrySet())
{
System.out.println(x.getKey() + "=" + x.getValue());
org.apache.catalina.Context c = tomcat.addWebapp(tomcat.getHost(), x.getKey(), x.getValue(), (LifecycleListener) new EmbedContextConfig());
if(contextDir != null && contextDir.isFile())
{
c.setConfigFile(contextDir.toURI().toURL());
}
// c.setParentClassLoader(Thread.currentThread().getContextClassLoader());
c.setJarScanner(new EmbedJarScanner());
}
// tomcat9中移除了getConnector()
// tomcat8在getConnector中会给service添加一个Connector并监听端口,而在tomcat9中需要手动创建一个Connector并给service设置Connector
Connector conn = new Connector("org.apache.coyote.http11.Http11NioProtocol");
conn.setPort(port);
// conn.setThrowOnFailure(true);// tomcat9才有,后续反射判断一下再设置
tomcat.getService().addConnector(conn);
tomcat.start();
if(await)
{
tomcat.getServer().await();
}
}
public void await() throws Exception
{
tomcat.getServer().await();
}
// String jarPath = this.getClass().getProtectionDomain().getCodeSource().getLocation().getFile() + "!";
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy