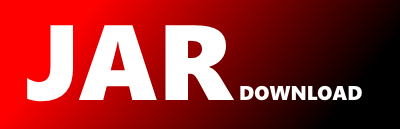
com.github.skjolber.log.domain.codegen.DomainFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of log-domain-codegen Show documentation
Show all versions of log-domain-codegen Show documentation
Source-code generator for log-domain yaml format.
package com.github.skjolber.log.domain.codegen;
import java.io.IOException;
import java.io.Reader;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import com.esotericsoftware.yamlbeans.YamlReader;
import com.github.skjolber.log.domain.model.Domain;
import com.github.skjolber.log.domain.model.Key;
import com.github.skjolber.log.domain.model.Tag;
public class DomainFactory {
@SuppressWarnings({ "rawtypes", "unchecked" })
public static Domain parse(Reader reader) throws IOException {
YamlReader yamlReader = new YamlReader(reader);
Object object = yamlReader.read();
Map map = (Map)object;
return parse(map);
}
@SuppressWarnings("unchecked")
public static Domain parse(Map map) throws IOException {
Domain ontology = new Domain();
ontology.setQualifier((String)map.get("qualifier"));
ontology.setVersion((String)map.get("version"));
ontology.setTargetPackage((String)map.get("package"));
ontology.setName((String)map.get("name"));
ontology.setDescription((String)map.get("description"));
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy