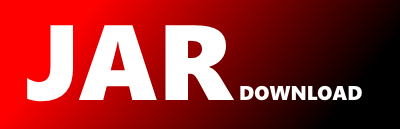
com.skjolberg.mockito.soap.SoapServerRule Maven / Gradle / Ivy
package com.skjolberg.mockito.soap;
import java.net.MalformedURLException;
import java.net.URL;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import org.apache.cxf.endpoint.Server;
import org.apache.cxf.jaxws.JaxWsServerFactoryBean;
/**
* Rule for mocking SOAP services using @{@linkplain JaxWsServerFactoryBean} to create {@linkplain Server}s.
* Each individual service requires a separate port.
*
* @author [email protected]
*
*/
public class SoapServerRule extends SoapServiceRule {
public static SoapServerRule newInstance() {
return new SoapServerRule();
}
private Map servers = new HashMap<>();
public void proxy(T target, Class port, String address, String wsdlLocation, List schemaLocations, Map properties) {
if(target == null) {
throw new IllegalArgumentException("Expected proxy target");
}
if(port == null) {
throw new IllegalArgumentException("Expect port class");
}
if(address == null) {
throw new IllegalArgumentException("Expected address");
}
assertValidAddress(address);
if(servers.containsKey(address)) {
throw new IllegalArgumentException("Server " + address + " already exists");
}
T serviceInterface = SoapServiceProxy.newInstance(target);
JaxWsServerFactoryBean svrFactory = new JaxWsServerFactoryBean();
svrFactory.setServiceClass(port);
svrFactory.setAddress(address);
svrFactory.setServiceBean(serviceInterface);
Map map = properties != null ? new HashMap(properties) : new HashMap();
if(wsdlLocation != null || schemaLocations != null) {
map.put("schema-validation-enabled", true);
if(wsdlLocation != null) {
svrFactory.setWsdlLocation(wsdlLocation);
}
if(schemaLocations != null) {
svrFactory.setSchemaLocations(schemaLocations);
}
}
svrFactory.setProperties(map);
Server server = svrFactory.create();
servers.put(address, server);
server.start();
}
protected void before() throws Throwable {
super.before();
}
protected void after() {
reset();
}
public void destroy() {
reset();
}
public void stop() {
for (Entry entry : servers.entrySet()) {
entry.getValue().stop();
}
}
public void start() {
for (Entry entry : servers.entrySet()) {
entry.getValue().start();
}
}
public void reset() {
for (Entry entry : servers.entrySet()) {
entry.getValue().getDestination().shutdown();
entry.getValue().destroy();
}
servers.clear();
}
private void assertValidAddress(String address) {
if (address != null && address.startsWith("local://")) {
return;
}
try {
new URL(address);
} catch (MalformedURLException e) {
throw new IllegalArgumentException("Expected valid address: " + address, e);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy