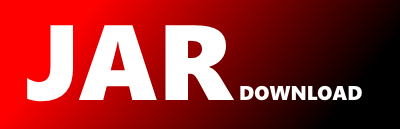
com.github.snowdream.android.app.dao.ISqlImpl Maven / Gradle / Ivy
/*
* Copyright (C) 2013 Snowdream Mobile
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.github.snowdream.android.app.dao;
import android.content.Context;
import com.github.snowdream.android.app.DownloadTask;
import com.j256.ormlite.android.apptools.OpenHelperManager;
import com.j256.ormlite.dao.Dao;
import java.sql.SQLException;
import java.util.List;
/**
* @author snowdream
* @version v1.0
* @date 2013-6-10
*/
public class ISqlImpl implements ISql {
private DatabaseHelper databaseHelper = null;
private Context mContext = null;
public ISqlImpl(Context context) {
mContext = context;
}
/*
* You'll need this in your class to release the helper when done.
*/
public void Release() {
if (databaseHelper != null) {
OpenHelperManager.releaseHelper();
databaseHelper = null;
}
}
/**
* You'll need this in your class to get the helper from the manager once
* per class.
*/
private DatabaseHelper getHelper() {
if (databaseHelper == null) {
databaseHelper = OpenHelperManager.getHelper(mContext, DatabaseHelper.class);
}
return databaseHelper;
}
public void addDownloadTask(DownloadTask task) throws SQLException {
if (task == null) {
return;
}
Dao taskDao = getHelper().getTaskDao();
taskDao.createOrUpdate(task);
}
public void updateDownloadTask(DownloadTask task) throws SQLException {
if (task == null) {
return;
}
Dao taskDao = getHelper().getTaskDao();
taskDao.update(task);
}
public DownloadTask queryDownloadTask(DownloadTask task) throws SQLException {
List tasks = null;
DownloadTask ttask = null;
if (task == null) {
return ttask;
}
Dao taskDao = getHelper().getTaskDao();
tasks = taskDao.queryForEq("url", task.getUrl());
if (tasks != null && tasks.size() > 0) {
ttask = tasks.get(0);
}
return ttask;
}
public void deleteDownloadTask(DownloadTask task) throws SQLException {
if (task == null) {
return;
}
Dao taskDao = getHelper().getTaskDao();
taskDao.delete(task);
}
/* @Override
public List queryDownloadTasksFromAlbum(Album album) throws SQLException {
List list = null;
Album talbum = null;
if (album == null) {
return list;
}
Dao albumDao = getHelper().getAlbumDao();
talbum = albumDao.queryForSameId(album);
if (talbum == null) {
return list;
}
list = new ArrayList();
for (DownloadTask task : talbum.getDownloadTasks()) {
list.add(task);
}
return list;
}
@Override
public List queryDownloadTasksFromAlbums(Albums albums) throws SQLException {
List list = null;
Albums talbums = null;
if (albums == null) {
return list;
}
Dao albumsDao = getHelper().getAlbumsDao();
talbums = albumsDao.queryForSameId(albums);
if (talbums == null) {
return list;
}
list = new ArrayList();
for (Album album : talbums.getAlbums()) {
List alist = queryDownloadTasksFromAlbum(album);
if (alist != null) {
list.addAll(alist);
}
}
return list;
}*/
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy