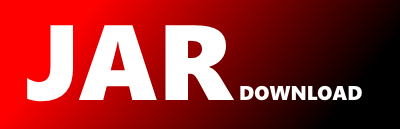
com.softwaresandbox.pubgclient.model.match.MatchResponse Maven / Gradle / Ivy
package com.softwaresandbox.pubgclient.model.match;
import com.google.gson.annotations.SerializedName;
import com.softwaresandbox.pubgclient.model.Links;
import com.softwaresandbox.pubgclient.model.match.asset.Asset;
import com.softwaresandbox.pubgclient.model.match.participant.Participant;
import com.softwaresandbox.pubgclient.model.match.roster.Roster;
import java.util.ArrayList;
import java.util.List;
import static java.util.stream.Collectors.toList;
public class MatchResponse {
@SerializedName("data")
private Match match;
@SerializedName("included")
@SuppressWarnings("MismatchedQueryAndUpdateOfCollection")
private List participantRosterAssetList = new ArrayList<>();
@SerializedName("links")
private Links links;
public Match getMatch() {
return match;
}
public void setMatch(Match match) {
this.match = match;
}
public Links getLinks() {
return links;
}
public void setLinks(Links links) {
this.links = links;
}
public List getParticipants() {
return this.participantRosterAssetList.stream()
.filter(participantRosterAsset -> participantRosterAsset instanceof Participant)
.map(participantRosterAsset -> (Participant) participantRosterAsset)
.collect(toList());
}
public List getRosters() {
return this.participantRosterAssetList.stream()
.filter(participantRosterAsset -> participantRosterAsset instanceof Roster)
.map(participantRosterAsset -> (Roster) participantRosterAsset)
.collect(toList());
}
public List getAssets() {
return this.participantRosterAssetList.stream()
.filter(participantRosterAsset -> participantRosterAsset instanceof Asset)
.map(participantRosterAsset -> (Asset) participantRosterAsset)
.collect(toList());
}
@Override
public String toString() {
return "MatchResponse{" +
"match=" + match +
", participants=" + getParticipants() +
", rosters=" + getRosters() +
", assets=" + getAssets() +
", links=" + links +
'}';
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy