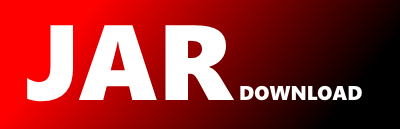
goja.mvc.Controller Maven / Gradle / Ivy
The newest version!
/*
* DO NOT ALTER OR REMOVE COPYRIGHT NOTICES OR THIS HEADER.
*
* Copyright (c) 2013-2014 sagyf Yang. The Four Group.
*/
package goja.mvc;
import goja.core.dto.PageDto;
import goja.core.kits.lang.DateKit;
import goja.mvc.render.NotModified;
import goja.rapid.mvc.datatables.DTCriterias;
import goja.rapid.mvc.datatables.DTDao;
import goja.rapid.mvc.datatables.DTResponse;
import goja.rapid.mvc.easyui.EuiDataGrid;
import goja.rapid.mvc.easyui.req.DataGridReq;
import goja.rapid.mvc.kits.Requests;
import goja.security.goja.SecurityKit;
import goja.security.shiro.AppUser;
import goja.security.shiro.Securitys;
import com.jfinal.plugin.activerecord.Model;
import com.jfinal.plugin.activerecord.Page;
import com.jfinal.plugin.activerecord.Record;
import com.google.common.base.Optional;
import com.google.common.base.Preconditions;
import com.google.common.base.Strings;
import com.google.common.collect.Maps;
import org.joda.time.DateTime;
import java.util.Collections;
import java.util.Enumeration;
import java.util.List;
import java.util.Map;
import javax.servlet.http.HttpServletRequest;
import static goja.core.StringPool.SLASH;
/**
* Controller.
*
* @author sagyf yang
* @version 1.0 2014-06-01 20:29
* @since JDK 1.6
*/
public class Controller extends com.jfinal.core.Controller {
/**
* Send a 304 Not Modified response
*/
protected static void notModified() {
new NotModified().render();
}
/**
* Rendering errors information, in Json format.
*
* @param error error message.
*/
protected void renderAjaxError(String error) {
renderJson(AjaxMessage.error(error));
}
/**
* Rendering errors information, in Json format.
*
* @param data error data.
*/
protected void renderAjaxError(T data) {
renderJson(AjaxMessage.error(data));
}
/**
* Rendering errors information, in Json format.
*
* @param error errors information.
* @param e exception.
*/
protected void renderAjaxError(String error, Exception e) {
renderJson(AjaxMessage.error(error, e));
}
/**
* In the form of JSON rendering failure information.
*
* @param failure failure information.
*/
protected void renderAjaxFailure(String failure) {
renderJson(AjaxMessage.failure(failure));
}
/**
* In the form of JSON rendering failure information.
*/
protected void renderAjaxFailure() {
renderJson(AjaxMessage.FAILURE);
}
/**
* In the form of JSON rendering forbidden information.
*/
protected void renderAjaxForbidden() {
renderJson(AjaxMessage.FORBIDDEN);
}
/**
* In the form of JSON rendering forbidden information.
*
* @param data the forbidden data.
* @param Generic parameter.
*/
protected void renderAjaxForbidden(T data) {
renderJson(AjaxMessage.forbidden(data));
}
/**
* In the form of JSON rendering forbidden information.
*
* @param message the forbidden message.
* @param data the forbidden data.
* @param Generic parameter.
*/
protected void renderAjaxForbidden(String message, T data) {
renderJson(AjaxMessage.forbidden(message, data));
}
/**
* In the form of JSON rendering success information.
*
* @param message success information.
*/
protected void renderAjaxSuccess(String message) {
renderJson(AjaxMessage.ok(message));
}
/**
* In the form of JSON rendering default success information.
*/
protected void renderAjaxSuccess() {
renderJson(AjaxMessage.OK);
}
/**
* With the success of data information.
*
* @param data the render data.
* @param Generic parameter.
*/
protected void renderAjaxSuccess(T data) {
renderJson(AjaxMessage.ok(data));
}
protected void renderAjaxSuccess(List list) {
if (list == null || list.isEmpty()) {
renderJson(AjaxMessage.nodata());
} else {
renderJson(AjaxMessage.ok(list));
}
}
/**
* News Ajax rendering not logged in.
*/
protected void renderAjaxNologin() {
renderJson(AjaxMessage.nologin());
}
/**
* News Ajax rendering not logged in.
*
* @param data the render data.
* @param Generic parameter.
*/
protected void renderAjaxNologin(T data) {
renderJson(AjaxMessage.nologin(data));
}
/**
* Render the empty data.
*/
protected void renderAjaxNodata() {
renderJson(AjaxMessage.NODATA);
}
/**
* Render the specified view as a string.
*
* @param view view template.
* @return the string.
*/
protected String renderTpl(String view) {
return template(view);
}
/**
* Render view as a string
*
* @param view view
* @return render string.
*/
protected String template(String view) {
final Enumeration attrs = getAttrNames();
final Map root = Maps.newHashMap();
while (attrs.hasMoreElements()) {
String attrName = attrs.nextElement();
root.put(attrName, getAttr(attrName));
}
return Freemarkers.processString(view, root);
}
/**
* Renderingtodo prompt
*/
protected void renderTODO() {
renderJson(AjaxMessage.developing());
}
/**
* Based on the current path structure is going to jump full Action of the path
*
* @param currentActionPath The current path, similar to/sau/index
* @param url The next path, similar to/au/login, the detail? The admin/detail.
* @return An Action under the full path.
*/
protected String parsePath(String currentActionPath, String url) {
if (url.startsWith(SLASH)) {
return url.split("\\?")[0];
} else if (!url.contains(SLASH)) {
return SLASH + currentActionPath.split(SLASH)[1] + SLASH + url.split("\\?")[0];
} else if (url.contains("http:") || url.contains("https:")) {
return null;
}
///abc/def","bcd/efg?abc
return currentActionPath + SLASH + url.split("\\?")[0];
}
/**
* The Request is ajax with return true
.
*
* @return true the request is ajax request.
*/
protected boolean isAjax() {
return Requests.ajax(getRequest());
}
/**
* Get parameters from the Request and encapsulation as an object for processing。
*
* @return jquery DataTables
*/
protected DTCriterias getCriterias() {
return DTCriterias.criteriasWithRequest(getRequest());
}
/**
* According to the request information of jquery.Datatables, the results of the query and
* returns the JSON data to the client. The specified query set the data.
*
* @param datas The data.
* @param criterias datatable criterias.
* @param Generic parameter.
*/
protected void renderDataTables(DTCriterias criterias, Page datas) {
Preconditions.checkNotNull(criterias, "datatable criterias is must be not null.");
DTResponse response =
DTResponse.build(criterias, datas.getList(), datas.getTotalRow(), datas.getTotalPage());
renderJson(response);
}
/**
* According to the request information of jquery.Datatables, the results of the query and
* returns the JSON data to the client. Automatically according to the request to create
* the query SQL and encapsulating the results back to the client.
*
* @param m_cls The Model class.
* @param criterias datatable criterias.
*/
protected void renderDataTables(DTCriterias criterias, Class extends Model> m_cls) {
Preconditions.checkNotNull(criterias, "datatable criterias is must be not null.");
DTResponse response = criterias.response(m_cls);
renderJson(response);
}
/**
* According to the request information of jquery.Datatables, the results of the query and
* returns the JSON data to the client. According to the SQL configuration file, in
* accordance with the Convention model_name.coloumns\model_name.where\model_name.order
* configured SQL to query and returns the results to the client.
*
* @param model_name The Model name.
* @param criterias datatable criterias.
*/
protected void renderDataTables(DTCriterias criterias, String model_name) {
Preconditions.checkNotNull(criterias, "datatable criterias is must be not null.");
final Page datas = DTDao.paginate(model_name, criterias);
DTResponse response =
DTResponse.build(criterias, datas.getList(), datas.getTotalRow(), datas.getTotalRow());
renderJson(response);
}
/**
* According to the request information of jquery.Datatables, the results of the query and
* returns the JSON data to the client. According to the SQL configuration file, in
* accordance with the Convention model_name.coloumns\model_name.where\model_name.order
* configured SQL to query and specify the parameters and return results to the client.
*
* @param criterias datatable criterias.
* @param sqlGroupName The Model name.
* @param params Query parameters
*/
protected void renderDataTables(DTCriterias criterias, String sqlGroupName, List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy