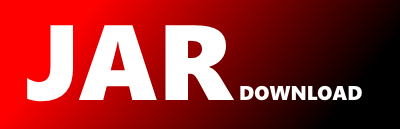
node_modules.apollo-codegen.src.utilities.CodeGenerator.ts Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of apollo-client-maven-plugin Show documentation
Show all versions of apollo-client-maven-plugin Show documentation
Maven plugin for generating graphql clients
export interface BasicGeneratedFile {
output: string
}
export class GeneratedFile implements BasicGeneratedFile {
scopeStack: Scope[] = [];
indentWidth = 2;
indentLevel = 0;
startOfIndentLevel = false;
public output = '';
pushScope(scope: Scope) {
this.scopeStack.push(scope);
}
popScope() {
return this.scopeStack.pop();
}
get scope(): Scope {
if (this.scopeStack.length < 1) throw new Error('No active scope');
return this.scopeStack[this.scopeStack.length - 1];
}
print(string?: string) {
if (string) {
this.output += string;
}
}
printNewline() {
if (this.output) {
this.print('\n');
this.startOfIndentLevel = false;
}
}
printNewlineIfNeeded() {
if (!this.startOfIndentLevel) {
this.printNewline();
}
}
printOnNewline(string?: string) {
if (string) {
this.printNewline();
this.printIndent();
this.print(string);
}
}
printIndent() {
const indentation = ' '.repeat(this.indentLevel * this.indentWidth);
this.output += indentation;
}
withIndent(closure: Function) {
if (!closure) return;
this.indentLevel++;
this.startOfIndentLevel = true;
closure();
this.indentLevel--;
}
withinBlock(closure: Function, open = ' {', close = '}') {
this.print(open);
this.withIndent(closure);
this.printOnNewline(close);
}
}
export default class CodeGenerator {
generatedFiles: { [fileName: string]: GeneratedFile } = {};
currentFile: GeneratedFile;
constructor(public context: Context) {
this.currentFile = new GeneratedFile();
}
withinFile(fileName: string, closure: Function) {
let file = this.generatedFiles[fileName];
if (!file) {
file = new GeneratedFile();
this.generatedFiles[fileName] = file;
}
const oldCurrentFile = this.currentFile;
this.currentFile = file;
closure();
this.currentFile = oldCurrentFile;
}
get output(): string {
return this.currentFile.output;
}
pushScope(scope: Scope) {
this.currentFile.pushScope(scope);
}
popScope() {
this.currentFile.popScope();
}
get scope(): Scope {
return this.currentFile.scope;
}
print(string?: string) {
this.currentFile.print(string);
}
printNewline() {
this.currentFile.printNewline();
}
printNewlineIfNeeded() {
this.currentFile.printNewlineIfNeeded();
}
printOnNewline(string?: string) {
this.currentFile.printOnNewline(string);
}
printIndent() {
this.currentFile.printIndent();
}
withIndent(closure: Function) {
this.currentFile.withIndent(closure);
}
withinBlock(closure: Function, open = ' {', close = '}') {
this.currentFile.withinBlock(closure, open, close);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy