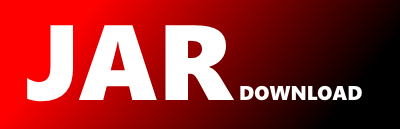
node_modules.graphql.jsutils.dedent.js.flow Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of apollo-client-maven-plugin Show documentation
Show all versions of apollo-client-maven-plugin Show documentation
Maven plugin for generating graphql clients
/**
* Copyright (c) 2017-present, Facebook, Inc.
*
* This source code is licensed under the MIT license found in the
* LICENSE file in the root directory of this source tree.
*
* @flow strict
*/
/**
* fixes indentation by removing leading spaces and tabs from each line
*/
function fixIndent(str: string): string {
const trimmedStr = str
.replace(/^\n*/m, '') // remove leading newline
.replace(/[ \t]*$/, ''); // remove trailing spaces and tabs
const indent = /^[ \t]*/.exec(trimmedStr)[0]; // figure out indent
return trimmedStr.replace(RegExp('^' + indent, 'mg'), ''); // remove indent
}
/**
* An ES6 string tag that fixes indentation. Also removes leading newlines
* and trailing spaces and tabs, but keeps trailing newlines.
*
* Example usage:
* const str = dedent`
* {
* test
* }
* `
* str === "{\n test\n}\n";
*/
export default function dedent(
strings: string | Array,
...values: Array
): string {
// when used as an ordinary function, allow passing a singleton string
const strArray = typeof strings === 'string' ? [strings] : strings;
const numValues = values.length;
const str = strArray.reduce((prev, cur, index) => {
let next = prev + cur;
if (index < numValues) {
next += values[index]; // interpolation
}
return next;
}, '');
return fixIndent(str);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy