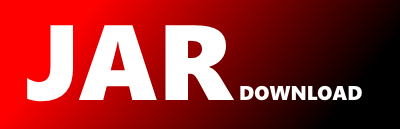
node_modules.graphql.utilities.introspectionQuery.js.flow Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of apollo-client-maven-plugin Show documentation
Show all versions of apollo-client-maven-plugin Show documentation
Maven plugin for generating graphql clients
/**
* Copyright (c) 2015-present, Facebook, Inc.
*
* This source code is licensed under the MIT license found in the
* LICENSE file in the root directory of this source tree.
*
* @flow strict
*/
import type { DirectiveLocationEnum } from '../language/directiveLocation';
export type IntrospectionOptions = {|
// Whether to include descriptions in the introspection result.
// Default: true
descriptions: boolean,
|};
export function getIntrospectionQuery(options?: IntrospectionOptions): string {
const descriptions = !(options && options.descriptions === false);
return `
query IntrospectionQuery {
__schema {
queryType { name }
mutationType { name }
subscriptionType { name }
types {
...FullType
}
directives {
name
${descriptions ? 'description' : ''}
locations
args {
...InputValue
}
}
}
}
fragment FullType on __Type {
kind
name
${descriptions ? 'description' : ''}
fields(includeDeprecated: true) {
name
${descriptions ? 'description' : ''}
args {
...InputValue
}
type {
...TypeRef
}
isDeprecated
deprecationReason
}
inputFields {
...InputValue
}
interfaces {
...TypeRef
}
enumValues(includeDeprecated: true) {
name
${descriptions ? 'description' : ''}
isDeprecated
deprecationReason
}
possibleTypes {
...TypeRef
}
}
fragment InputValue on __InputValue {
name
${descriptions ? 'description' : ''}
type { ...TypeRef }
defaultValue
}
fragment TypeRef on __Type {
kind
name
ofType {
kind
name
ofType {
kind
name
ofType {
kind
name
ofType {
kind
name
ofType {
kind
name
ofType {
kind
name
ofType {
kind
name
}
}
}
}
}
}
}
}
`;
}
export const introspectionQuery = getIntrospectionQuery();
export type IntrospectionQuery = {|
+__schema: IntrospectionSchema,
|};
export type IntrospectionSchema = {|
+queryType: IntrospectionNamedTypeRef,
+mutationType: ?IntrospectionNamedTypeRef,
+subscriptionType: ?IntrospectionNamedTypeRef,
+types: $ReadOnlyArray,
+directives: $ReadOnlyArray,
|};
export type IntrospectionType =
| IntrospectionScalarType
| IntrospectionObjectType
| IntrospectionInterfaceType
| IntrospectionUnionType
| IntrospectionEnumType
| IntrospectionInputObjectType;
export type IntrospectionOutputType =
| IntrospectionScalarType
| IntrospectionObjectType
| IntrospectionInterfaceType
| IntrospectionUnionType
| IntrospectionEnumType;
export type IntrospectionInputType =
| IntrospectionScalarType
| IntrospectionEnumType
| IntrospectionInputObjectType;
export type IntrospectionScalarType = {
+kind: 'SCALAR',
+name: string,
+description?: ?string,
};
export type IntrospectionObjectType = {
+kind: 'OBJECT',
+name: string,
+description?: ?string,
+fields: $ReadOnlyArray,
+interfaces: $ReadOnlyArray<
IntrospectionNamedTypeRef,
>,
};
export type IntrospectionInterfaceType = {
+kind: 'INTERFACE',
+name: string,
+description?: ?string,
+fields: $ReadOnlyArray,
+possibleTypes: $ReadOnlyArray<
IntrospectionNamedTypeRef,
>,
};
export type IntrospectionUnionType = {
+kind: 'UNION',
+name: string,
+description?: ?string,
+possibleTypes: $ReadOnlyArray<
IntrospectionNamedTypeRef,
>,
};
export type IntrospectionEnumType = {
+kind: 'ENUM',
+name: string,
+description?: ?string,
+enumValues: $ReadOnlyArray,
};
export type IntrospectionInputObjectType = {
+kind: 'INPUT_OBJECT',
+name: string,
+description?: ?string,
+inputFields: $ReadOnlyArray,
};
export type IntrospectionListTypeRef<
T: IntrospectionTypeRef = IntrospectionTypeRef,
> = {
+kind: 'LIST',
+ofType: T,
};
export type IntrospectionNonNullTypeRef<
T: IntrospectionTypeRef = IntrospectionTypeRef,
> = {
+kind: 'NON_NULL',
+ofType: T,
};
export type IntrospectionTypeRef =
| IntrospectionNamedTypeRef
| IntrospectionListTypeRef
| IntrospectionNonNullTypeRef<
| IntrospectionNamedTypeRef
| IntrospectionListTypeRef,
>;
export type IntrospectionOutputTypeRef =
| IntrospectionNamedTypeRef
| IntrospectionListTypeRef
| IntrospectionNonNullTypeRef<
| IntrospectionNamedTypeRef
| IntrospectionListTypeRef,
>;
export type IntrospectionInputTypeRef =
| IntrospectionNamedTypeRef
| IntrospectionListTypeRef
| IntrospectionNonNullTypeRef<
| IntrospectionNamedTypeRef
| IntrospectionListTypeRef,
>;
export type IntrospectionNamedTypeRef<
T: IntrospectionType = IntrospectionType,
> = {
+kind: $PropertyType,
+name: string,
};
export type IntrospectionField = {|
+name: string,
+description?: ?string,
+args: $ReadOnlyArray,
+type: IntrospectionOutputTypeRef,
+isDeprecated: boolean,
+deprecationReason: ?string,
|};
export type IntrospectionInputValue = {|
+name: string,
+description?: ?string,
+type: IntrospectionInputTypeRef,
+defaultValue: ?string,
|};
export type IntrospectionEnumValue = {|
+name: string,
+description?: ?string,
+isDeprecated: boolean,
+deprecationReason: ?string,
|};
export type IntrospectionDirective = {|
+name: string,
+description?: ?string,
+locations: $ReadOnlyArray,
+args: $ReadOnlyArray,
|};
© 2015 - 2025 Weber Informatics LLC | Privacy Policy