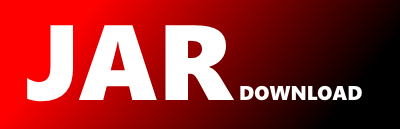
IPXACT2009ScalaCases.constraints.scala Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of spinalhdl-schema-gen_2.11 Show documentation
Show all versions of spinalhdl-schema-gen_2.11 Show documentation
SpinalHDL Schemetic Generator
The newest version!
package IPXACT2009ScalaCases
// Generated by IPXACT2009scalaxb.
sealed trait CellFunctionValueType
object CellFunctionValueType {
def fromString(value: String, scope: scala.xml.NamespaceBinding)(implicit fmt: IPXACT2009scalaxb.XMLFormat[CellFunctionValueType]): CellFunctionValueType = fmt.reads(scala.xml.Text(value), Nil) match {
case Right(x: CellFunctionValueType) => x
case x => throw new RuntimeException(s"fromString returned unexpected value $x for input $value")
}
lazy val values: Seq[CellFunctionValueType] = Seq(Nand2, Buf, Inv, Mux21, Dff, Latch, Xor2)
}
case object Nand2 extends CellFunctionValueType { override def toString = "nand2" }
case object Buf extends CellFunctionValueType { override def toString = "buf" }
case object Inv extends CellFunctionValueType { override def toString = "inv" }
case object Mux21 extends CellFunctionValueType { override def toString = "mux21" }
case object Dff extends CellFunctionValueType { override def toString = "dff" }
case object Latch extends CellFunctionValueType { override def toString = "latch" }
case object Xor2 extends CellFunctionValueType { override def toString = "xor2" }
sealed trait CellClassValueType
object CellClassValueType {
def fromString(value: String, scope: scala.xml.NamespaceBinding)(implicit fmt: IPXACT2009scalaxb.XMLFormat[CellClassValueType]): CellClassValueType = fmt.reads(scala.xml.Text(value), Nil) match {
case Right(x: CellClassValueType) => x
case x => throw new RuntimeException(s"fromString returned unexpected value $x for input $value")
}
lazy val values: Seq[CellClassValueType] = Seq(Combinational, Sequential)
}
case object Combinational extends CellClassValueType { override def toString = "combinational" }
case object Sequential extends CellClassValueType { override def toString = "sequential" }
sealed trait CellStrengthValueType
object CellStrengthValueType {
def fromString(value: String, scope: scala.xml.NamespaceBinding)(implicit fmt: IPXACT2009scalaxb.XMLFormat[CellStrengthValueType]): CellStrengthValueType = fmt.reads(scala.xml.Text(value), Nil) match {
case Right(x: CellStrengthValueType) => x
case x => throw new RuntimeException(s"fromString returned unexpected value $x for input $value")
}
lazy val values: Seq[CellStrengthValueType] = Seq(Low, Median, High)
}
case object Low extends CellStrengthValueType { override def toString = "low" }
case object Median extends CellStrengthValueType { override def toString = "median" }
case object High extends CellStrengthValueType { override def toString = "high" }
sealed trait EdgeValueType
object EdgeValueType {
def fromString(value: String, scope: scala.xml.NamespaceBinding)(implicit fmt: IPXACT2009scalaxb.XMLFormat[EdgeValueType]): EdgeValueType = fmt.reads(scala.xml.Text(value), Nil) match {
case Right(x: EdgeValueType) => x
case x => throw new RuntimeException(s"fromString returned unexpected value $x for input $value")
}
lazy val values: Seq[EdgeValueType] = Seq(Rise, Fall)
}
case object Rise extends EdgeValueType { override def toString = "rise" }
case object Fall extends EdgeValueType { override def toString = "fall" }
sealed trait DelayValueType
object DelayValueType {
def fromString(value: String, scope: scala.xml.NamespaceBinding)(implicit fmt: IPXACT2009scalaxb.XMLFormat[DelayValueType]): DelayValueType = fmt.reads(scala.xml.Text(value), Nil) match {
case Right(x: DelayValueType) => x
case x => throw new RuntimeException(s"fromString returned unexpected value $x for input $value")
}
lazy val values: Seq[DelayValueType] = Seq(Min, Max)
}
case object Min extends DelayValueType { override def toString = "min" }
case object Max extends DelayValueType { override def toString = "max" }
/** List of clocks associated with the component that are not associated with ports. Set the clockSource attribute on the clockDriver to indicate the source of a clock not associated with a particular component port.
*/
case class OtherClocks(otherClockDriver: Seq[OtherClockDriver] = Nil)
case class CellFunction(value: CellFunctionValueType,
attributes: Map[String, IPXACT2009scalaxb.DataRecord[Any]] = Map.empty) extends CellSpecificationOption {
lazy val spiritcellStrength = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}cellStrength") map { _.as[CellStrengthValueType]}
}
case class CellClass(value: CellClassValueType,
attributes: Map[String, IPXACT2009scalaxb.DataRecord[Any]] = Map.empty) extends CellSpecificationOption {
lazy val spiritcellStrength = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}cellStrength") map { _.as[CellStrengthValueType]}
}
case class CellSpecification(cellspecificationoption: IPXACT2009scalaxb.DataRecord[CellSpecificationOption])
trait CellSpecificationOption
case class TimingConstraint(value: Float,
attributes: Map[String, IPXACT2009scalaxb.DataRecord[Any]] = Map.empty) {
lazy val spiritclockEdge = attributes("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}clockEdge").as[EdgeValueType]
lazy val spiritdelayType = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}delayType") map { _.as[DelayValueType]}
lazy val clockName = attributes("@clockName").as[String]
}
case class DriveConstraint(cellSpecification: CellSpecification)
case class LoadConstraint(cellSpecification: CellSpecification,
count: Option[BigInt] = None)
case class LeftType3(value: BigInt)
case class RightType3(value: BigInt)
case class Vector3(left: LeftType3,
right: RightType3)
case class ConstraintSet(nameGroupOptionalSequence1: NameGroupOptionalSequence,
vector: Option[Vector3] = None,
driveConstraint: Option[DriveConstraint] = None,
loadConstraint: Option[LoadConstraint] = None,
timingConstraint: Seq[TimingConstraint] = Nil,
attributes: Map[String, IPXACT2009scalaxb.DataRecord[Any]] = Map.empty) {
lazy val spiritconstraintSetId = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}constraintSetId") map { _.as[String]}
}
case class ConstraintSets(constraintSet: Seq[ConstraintSet] = Nil)
/** Defines constraints that apply to a wire type port in an abstraction definition.
*/
case class AbstractionDefPortConstraintsType(abstractiondefportconstraintstypeoption: IPXACT2009scalaxb.DataRecord[AbstractionDefPortConstraintsTypeOption])
case class AbstractionDefPortConstraintsTypeSequence3(loadConstraint: LoadConstraint) extends AbstractionDefPortConstraintsTypeOption
trait AbstractionDefPortConstraintsTypeOption
case class AbstractionDefPortConstraintsTypeSequence2(driveConstraint: DriveConstraint,
loadConstraint: Option[LoadConstraint] = None) extends AbstractionDefPortConstraintsTypeOption
case class AbstractionDefPortConstraintsTypeSequence1(timingConstraint: Seq[TimingConstraint] = Nil,
driveConstraint: Option[DriveConstraint] = None,
loadConstraint: Option[LoadConstraint] = None) extends AbstractionDefPortConstraintsTypeOption
© 2015 - 2025 Weber Informatics LLC | Privacy Policy