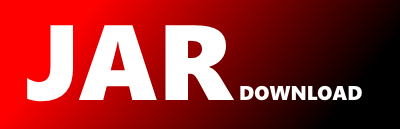
IPXACT2022ScalaCases.signalDrivers.scala Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of spinalhdl-schema-gen_2.11 Show documentation
Show all versions of spinalhdl-schema-gen_2.11 Show documentation
SpinalHDL Schemetic Generator
The newest version!
// Generated by IPXACT2022scalaxb.
package IPXACT2022ScalaCases
case class ClockDriver(clockPeriod: IPXACT2022ScalaCases.ClockPeriod,
clockPulseOffset: IPXACT2022ScalaCases.ClockPulseOffset,
clockPulseValue: IPXACT2022ScalaCases.UnsignedBitExpression,
clockPulseDuration: IPXACT2022ScalaCases.ClockPulseDuration,
attributes: Map[String, IPXACT2022scalaxb.DataRecord[Any]] = Map.empty) extends ClockDriverTypable with DriverTypeOption {
lazy val xmlid = attributes.get("@{http://www.w3.org/XML/1998/namespace}id") map { _.as[String]}
lazy val clockName = attributes.get("@clockName") map { _.as[String]}
}
case class SingleShotOffset(value: String,
attributes: Map[String, IPXACT2022scalaxb.DataRecord[Any]] = Map.empty) extends RealExpressionable {
lazy val minimum = attributes.get("@minimum") map { _.as[Double]}
lazy val maximum = attributes.get("@maximum") map { _.as[Double]}
lazy val units = attributes("@units").as[DelayValueUnitType]
}
case class SingleShotValue(value: String,
attributes: Map[String, IPXACT2022scalaxb.DataRecord[Any]] = Map.empty) extends UnsignedBitVectorExpressionable
case class SingleShotDuration(value: String,
attributes: Map[String, IPXACT2022scalaxb.DataRecord[Any]] = Map.empty) extends RealExpressionable {
lazy val minimum = attributes.get("@minimum") map { _.as[Double]}
lazy val maximum = attributes.get("@maximum") map { _.as[Double]}
lazy val units = attributes("@units").as[DelayValueUnitType]
}
case class SingleShotDriver(singleShotOffset: IPXACT2022ScalaCases.SingleShotOffset,
singleShotValue: IPXACT2022ScalaCases.SingleShotValue,
singleShotDuration: IPXACT2022ScalaCases.SingleShotDuration) extends DriverTypeOption
sealed trait DriverTypeType
object DriverTypeType {
def fromString(value: String, scope: scala.xml.NamespaceBinding)(implicit fmt: IPXACT2022scalaxb.XMLFormat[IPXACT2022ScalaCases.DriverTypeType]): DriverTypeType = fmt.reads(scala.xml.Text(value), Nil) match {
case Right(x: DriverTypeType) => x
case x => throw new RuntimeException(s"fromString returned unexpected value $x for input $value")
}
lazy val values: Seq[DriverTypeType] = Seq(Clock, SingleShot, AnyType)
}
case object Clock extends DriverTypeType { override def toString = "clock" }
case object SingleShot extends DriverTypeType { override def toString = "singleShot" }
case object AnyType extends DriverTypeType { override def toString = "any" }
case class RequiresDriver(value: Boolean,
attributes: Map[String, IPXACT2022scalaxb.DataRecord[Any]] = Map.empty) extends WireOption {
lazy val driverType = attributes("@driverType").as[DriverTypeType]
}
case class ViewRef(value: String,
attributes: Map[String, IPXACT2022scalaxb.DataRecord[Any]] = Map.empty) {
lazy val xmlid = attributes.get("@{http://www.w3.org/XML/1998/namespace}id") map { _.as[String]}
}
/** Wire port driver type.
*/
case class DriverType(drivertypesequence1: Option[IPXACT2022ScalaCases.DriverTypeSequence1] = None,
attributes: Map[String, IPXACT2022scalaxb.DataRecord[Any]] = Map.empty) {
lazy val xmlid = attributes.get("@{http://www.w3.org/XML/1998/namespace}id") map { _.as[String]}
}
case class DriverTypeSequence1(range: Option[IPXACT2022ScalaCases.RangeType] = None,
viewRef: Seq[IPXACT2022ScalaCases.ViewRef] = Nil,
drivertypeoption: IPXACT2022scalaxb.DataRecord[IPXACT2022ScalaCases.DriverTypeOption])
trait DriverTypeOption
case class Drivers(driver: Seq[IPXACT2022ScalaCases.DriverType] = Nil)
case class ClockPeriod(value: String,
attributes: Map[String, IPXACT2022scalaxb.DataRecord[Any]] = Map.empty) extends RealExpressionable {
lazy val minimum = attributes.get("@minimum") map { _.as[Double]}
lazy val maximum = attributes.get("@maximum") map { _.as[Double]}
lazy val units = attributes("@units").as[DelayValueUnitType]
}
case class ClockPulseOffset(value: String,
attributes: Map[String, IPXACT2022scalaxb.DataRecord[Any]] = Map.empty) extends RealExpressionable {
lazy val minimum = attributes.get("@minimum") map { _.as[Double]}
lazy val maximum = attributes.get("@maximum") map { _.as[Double]}
lazy val units = attributes("@units").as[DelayValueUnitType]
}
case class ClockPulseDuration(value: String,
attributes: Map[String, IPXACT2022scalaxb.DataRecord[Any]] = Map.empty) extends RealExpressionable {
lazy val minimum = attributes.get("@minimum") map { _.as[Double]}
lazy val maximum = attributes.get("@maximum") map { _.as[Double]}
lazy val units = attributes("@units").as[DelayValueUnitType]
}
trait ClockDriverTypable {
def clockPeriod: IPXACT2022ScalaCases.ClockPeriod
def clockPulseOffset: IPXACT2022ScalaCases.ClockPulseOffset
def clockPulseValue: IPXACT2022ScalaCases.UnsignedBitExpression
def clockPulseDuration: IPXACT2022ScalaCases.ClockPulseDuration
def xmlid: Option[String]
}
case class ClockDriverType(clockPeriod: IPXACT2022ScalaCases.ClockPeriod,
clockPulseOffset: IPXACT2022ScalaCases.ClockPulseOffset,
clockPulseValue: IPXACT2022ScalaCases.UnsignedBitExpression,
clockPulseDuration: IPXACT2022ScalaCases.ClockPulseDuration,
attributes: Map[String, IPXACT2022scalaxb.DataRecord[Any]] = Map.empty) extends ClockDriverTypable {
lazy val xmlid = attributes.get("@{http://www.w3.org/XML/1998/namespace}id") map { _.as[String]}
}
case class OtherClockDriver(clockPeriod: IPXACT2022ScalaCases.ClockPeriod,
clockPulseOffset: IPXACT2022ScalaCases.ClockPulseOffset,
clockPulseValue: IPXACT2022ScalaCases.UnsignedBitExpression,
clockPulseDuration: IPXACT2022ScalaCases.ClockPulseDuration,
attributes: Map[String, IPXACT2022scalaxb.DataRecord[Any]] = Map.empty) extends ClockDriverTypable {
lazy val xmlid = attributes.get("@{http://www.w3.org/XML/1998/namespace}id") map { _.as[String]}
lazy val clockName = attributes("@clockName").as[String]
lazy val clockSource = attributes.get("@clockSource") map { _.as[String]}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy