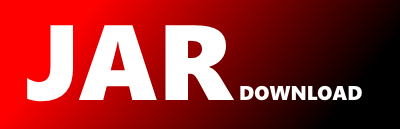
IPXACT2022ScalaCases.simpleTypes.scala Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of spinalhdl-schema-gen_2.11 Show documentation
Show all versions of spinalhdl-schema-gen_2.11 Show documentation
SpinalHDL Schemetic Generator
The newest version!
// Generated by IPXACT2022scalaxb.
package IPXACT2022ScalaCases
/** An unsigned longint which supports an expression value.
*/
trait UnsignedLongintExpressionable extends ComplexBaseExpressionable {
def value: String
def minimum: Option[Int]
def maximum: Option[Int]
def attributes: Map[String, IPXACT2022scalaxb.DataRecord[Any]]
}
/** An unsigned longint which supports an expression value.
*/
case class UnsignedLongintExpression(value: String,
attributes: Map[String, IPXACT2022scalaxb.DataRecord[Any]] = Map.empty) extends UnsignedLongintExpressionable {
lazy val minimum = attributes.get("@minimum") map { _.as[Int]}
lazy val maximum = attributes.get("@maximum") map { _.as[Int]}
}
/** A positive unsigned longint which supports an expression value.
*/
trait UnsignedPositiveLongintExpressionable extends ComplexBaseExpressionable {
def value: String
def minimum: Option[Int]
def maximum: Option[Int]
def attributes: Map[String, IPXACT2022scalaxb.DataRecord[Any]]
}
/** A positive unsigned longint which supports an expression value.
*/
case class UnsignedPositiveLongintExpression(value: String,
attributes: Map[String, IPXACT2022scalaxb.DataRecord[Any]] = Map.empty) extends UnsignedPositiveLongintExpressionable {
lazy val minimum = attributes.get("@minimum") map { _.as[Int]}
lazy val maximum = attributes.get("@maximum") map { _.as[Int]}
}
/** An unsigned longint which supports an expression value.
*/
case class SignedLongintExpression(value: String,
attributes: Map[String, IPXACT2022scalaxb.DataRecord[Any]] = Map.empty) extends ComplexBaseExpressionable {
lazy val minimum = attributes.get("@minimum") map { _.as[Int]}
lazy val maximum = attributes.get("@maximum") map { _.as[Int]}
}
/** An unsigned int which supports an expression value.
*/
trait UnsignedIntExpressionable extends ComplexBaseExpressionable {
def value: String
def minimum: Option[Int]
def maximum: Option[Int]
def attributes: Map[String, IPXACT2022scalaxb.DataRecord[Any]]
}
/** An unsigned int which supports an expression value.
*/
case class UnsignedIntExpression(value: String,
attributes: Map[String, IPXACT2022scalaxb.DataRecord[Any]] = Map.empty) extends UnsignedIntExpressionable {
lazy val minimum = attributes.get("@minimum") map { _.as[Int]}
lazy val maximum = attributes.get("@maximum") map { _.as[Int]}
}
/** An positive unsigned int which supports an expression value.
*/
trait UnsignedPositiveIntExpressionable extends ComplexBaseExpressionable {
def value: String
def minimum: Option[Int]
def maximum: Option[Int]
def attributes: Map[String, IPXACT2022scalaxb.DataRecord[Any]]
}
/** An positive unsigned int which supports an expression value.
*/
case class UnsignedPositiveIntExpression(value: String,
attributes: Map[String, IPXACT2022scalaxb.DataRecord[Any]] = Map.empty) extends UnsignedPositiveIntExpressionable {
lazy val minimum = attributes.get("@minimum") map { _.as[Int]}
lazy val maximum = attributes.get("@maximum") map { _.as[Int]}
}
/** An positive unsigned int which supports an expression value.
*/
case class UnresolvedUnsignedPositiveIntExpression(value: String,
attributes: Map[String, IPXACT2022scalaxb.DataRecord[Any]] = Map.empty) extends ComplexBaseExpressionable {
lazy val minimum = attributes.get("@minimum") map { _.as[Int]}
lazy val maximum = attributes.get("@maximum") map { _.as[Int]}
}
/** A real which supports an expression value.
*/
trait RealExpressionable extends ComplexBaseExpressionable {
def value: String
def minimum: Option[Double]
def maximum: Option[Double]
def attributes: Map[String, IPXACT2022scalaxb.DataRecord[Any]]
}
/** A real which supports an expression value.
*/
case class RealExpression(value: String,
attributes: Map[String, IPXACT2022scalaxb.DataRecord[Any]] = Map.empty) extends RealExpressionable {
lazy val minimum = attributes.get("@minimum") map { _.as[Double]}
lazy val maximum = attributes.get("@maximum") map { _.as[Double]}
}
/** Represents a string which cannot be fully resolved.
*/
trait UnresolvedStringExpressionable extends ComplexBaseExpressionable {
def value: String
def attributes: Map[String, IPXACT2022scalaxb.DataRecord[Any]]
}
/** Represents a string which cannot be fully resolved.
*/
case class UnresolvedStringExpression(value: String,
attributes: Map[String, IPXACT2022scalaxb.DataRecord[Any]] = Map.empty) extends UnresolvedStringExpressionable
/** Represents a string. It supports an expression value.
*/
trait StringExpressionable extends ComplexBaseExpressionable {
def value: String
def attributes: Map[String, IPXACT2022scalaxb.DataRecord[Any]]
}
/** Represents a string. It supports an expression value.
*/
case class StringExpression(value: String,
attributes: Map[String, IPXACT2022scalaxb.DataRecord[Any]] = Map.empty) extends StringExpressionable
/** Represents a single-bit/bool. It supports an expression value.
*/
case class UnsignedBitExpression(value: String,
attributes: Map[String, IPXACT2022scalaxb.DataRecord[Any]] = Map.empty) extends ComplexBaseExpressionable
/** Represents an expression qualified by an accompanying type. It supports an expression value.
*/
case class QualifiedExpression(value: String,
attributes: Map[String, IPXACT2022scalaxb.DataRecord[Any]] = Map.empty) extends ComplexBaseExpressionable with DriverTypeOption
/** Represents a bit-string. It supports an expression value.
*/
trait UnsignedBitVectorExpressionable extends ComplexBaseExpressionable with PortMapOption with WireOption {
def value: String
def attributes: Map[String, IPXACT2022scalaxb.DataRecord[Any]]
}
/** Represents a bit-string. It supports an expression value.
*/
case class UnsignedBitVectorExpression(value: String,
attributes: Map[String, IPXACT2022scalaxb.DataRecord[Any]] = Map.empty) extends UnsignedBitVectorExpressionable
/** Represents the base-type for an expressions.
*/
trait ComplexBaseExpressionable {
def value: String
def attributes: Map[String, IPXACT2022scalaxb.DataRecord[Any]]
}
/** Represents the base-type for an expressions.
*/
case class ComplexBaseExpression(value: String,
attributes: Map[String, IPXACT2022scalaxb.DataRecord[Any]] = Map.empty) extends ComplexBaseExpressionable
/** IP-XACT URI, like a standard xs:anyURI except that it can contain environment variables in the ${ } form, to be replaced by their value to provide the underlying URI
*/
trait IpxactURIable {
def value: String
}
/** IP-XACT URI, like a standard xs:anyURI except that it can contain environment variables in the ${ } form, to be replaced by their value to provide the underlying URI
*/
case class IpxactURI(value: String) extends IpxactURIable
/** Unsigned Bit Expression which cannot be fully resolved.
*/
case class UnresolvedUnsignedBitExpression(value: String,
attributes: Map[String, IPXACT2022scalaxb.DataRecord[Any]] = Map.empty) extends ComplexBaseExpressionable
© 2015 - 2025 Weber Informatics LLC | Privacy Policy