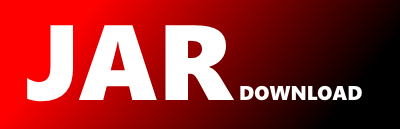
IPXACT2009ScalaCases.busInterface.scala Maven / Gradle / Ivy
package IPXACT2009ScalaCases
// Generated by IPXACT2009scalaxb.
sealed trait BitSteeringType
object BitSteeringType {
def fromString(value: String, scope: scala.xml.NamespaceBinding)(implicit fmt: IPXACT2009scalaxb.XMLFormat[BitSteeringType]): BitSteeringType = fmt.reads(scala.xml.Text(value), Nil) match {
case Right(x: BitSteeringType) => x
case x => throw new RuntimeException(s"fromString returned unexpected value $x for input $value")
}
lazy val values: Seq[BitSteeringType] = Seq(On, Off)
}
case object On extends BitSteeringType { override def toString = "on" }
case object Off extends BitSteeringType { override def toString = "off" }
sealed trait EndianessType
object EndianessType {
def fromString(value: String, scope: scala.xml.NamespaceBinding)(implicit fmt: IPXACT2009scalaxb.XMLFormat[EndianessType]): EndianessType = fmt.reads(scala.xml.Text(value), Nil) match {
case Right(x: EndianessType) => x
case x => throw new RuntimeException(s"fromString returned unexpected value $x for input $value")
}
lazy val values: Seq[EndianessType] = Seq(Big, Little)
}
case object Big extends EndianessType { override def toString = "big" }
case object Little extends EndianessType { override def toString = "little" }
case class BusInterfaces(busInterface: Seq[BusInterfaceType] = Nil)
case class LeftType(value: BigInt,
attributes: Map[String, IPXACT2009scalaxb.DataRecord[Any]] = Map.empty) {
lazy val spiritformat = attributes("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}format").as[FormatType]
lazy val spiritresolve = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}resolve") map { _.as[ResolveType]}
lazy val spiritid = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}id") map { _.as[String]}
lazy val spiritdependency = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}dependency") map { _.as[String]}
lazy val spiritchoiceRef = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}choiceRef") map { _.as[String]}
lazy val spiritorder = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}order") map { _.as[Float]}
lazy val spiritconfigGroups = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}configGroups") map { _.as[Seq[String]]}
lazy val spiritbitStringLength = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}bitStringLength") map { _.as[BigInt]}
lazy val spiritminimum = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}minimum") map { _.as[String]}
lazy val spiritmaximum = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}maximum") map { _.as[String]}
lazy val spiritrangeType = attributes("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}rangeType").as[RangeTypeType]
lazy val spiritprompt = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}prompt") map { _.as[String]}
}
case class RightType(value: BigInt,
attributes: Map[String, IPXACT2009scalaxb.DataRecord[Any]] = Map.empty) {
lazy val spiritformat = attributes("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}format").as[FormatType]
lazy val spiritresolve = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}resolve") map { _.as[ResolveType]}
lazy val spiritid = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}id") map { _.as[String]}
lazy val spiritdependency = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}dependency") map { _.as[String]}
lazy val spiritchoiceRef = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}choiceRef") map { _.as[String]}
lazy val spiritorder = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}order") map { _.as[Float]}
lazy val spiritconfigGroups = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}configGroups") map { _.as[Seq[String]]}
lazy val spiritbitStringLength = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}bitStringLength") map { _.as[BigInt]}
lazy val spiritminimum = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}minimum") map { _.as[String]}
lazy val spiritmaximum = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}maximum") map { _.as[String]}
lazy val spiritrangeType = attributes("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}rangeType").as[RangeTypeType]
lazy val spiritprompt = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}prompt") map { _.as[String]}
}
case class Vector(left: LeftType,
right: RightType)
case class LogicalPort(name: String,
vector: Option[Vector] = None)
case class PhysicalPort(name: String,
vector: Option[Vector4] = None)
case class PortMap(logicalPort: LogicalPort,
physicalPort: PhysicalPort)
case class PortMaps(portMap: Seq[PortMap] = Nil)
case class BitSteering(value: BitSteeringType,
attributes: Map[String, IPXACT2009scalaxb.DataRecord[Any]] = Map.empty) {
lazy val spiritformat = attributes("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}format").as[FormatType]
lazy val spiritresolve = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}resolve") map { _.as[ResolveType]}
lazy val spiritid = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}id") map { _.as[String]}
lazy val spiritdependency = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}dependency") map { _.as[String]}
lazy val spiritchoiceRef = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}choiceRef") map { _.as[String]}
lazy val spiritorder = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}order") map { _.as[Float]}
lazy val spiritconfigGroups = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}configGroups") map { _.as[Seq[String]]}
lazy val spiritbitStringLength = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}bitStringLength") map { _.as[BigInt]}
lazy val spiritminimum = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}minimum") map { _.as[String]}
lazy val spiritmaximum = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}maximum") map { _.as[String]}
lazy val spiritrangeType = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}rangeType") map { _.as[RangeTypeType]}
lazy val spiritprompt = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}prompt") map { _.as[String]}
}
/** Type definition for a busInterface in a component
*/
case class BusInterfaceType(nameGroupSequence1: NameGroupSequence,
busType: LibraryRefType,
abstractionType: Option[LibraryRefType] = None,
interfaceModeOption4: IPXACT2009scalaxb.DataRecord[InterfaceModeOption],
connectionRequired: Option[Boolean] = None,
portMaps: Option[PortMaps] = None,
bitsInLau: Option[BigInt] = None,
bitSteering: Option[BitSteering] = None,
endianness: Option[EndianessType] = None,
parameters: Option[Parameters] = None,
vendorExtensions: Option[VendorExtensions] = None,
attributes: Map[String, IPXACT2009scalaxb.DataRecord[Any]] = Map.empty)
case class Channel(nameGroupSequence1: NameGroupSequence,
busInterfaceRef: Seq[String] = Nil)
case class Channels(channel: Seq[Channel] = Nil)
case class RemapPort(value: String,
attributes: Map[String, IPXACT2009scalaxb.DataRecord[Any]] = Map.empty) {
lazy val portNameRef = attributes("@portNameRef").as[String]
lazy val portIndex = attributes.get("@portIndex") map { _.as[BigInt]}
}
case class RemapPorts(remapPort: Seq[RemapPort] = Nil)
case class RemapState(nameGroupSequence1: NameGroupSequence,
remapPorts: Option[RemapPorts] = None)
case class RemapStates(remapState: Seq[RemapState] = Nil)
case class BaseAddress(value: String,
attributes: Map[String, IPXACT2009scalaxb.DataRecord[Any]] = Map.empty) {
lazy val spiritformat = attributes("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}format").as[FormatType]
lazy val spiritresolve = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}resolve") map { _.as[ResolveType]}
lazy val spiritid = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}id") map { _.as[String]}
lazy val spiritdependency = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}dependency") map { _.as[String]}
lazy val spiritchoiceRef = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}choiceRef") map { _.as[String]}
lazy val spiritorder = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}order") map { _.as[Float]}
lazy val spiritconfigGroups = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}configGroups") map { _.as[Seq[String]]}
lazy val spiritbitStringLength = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}bitStringLength") map { _.as[BigInt]}
lazy val spiritminimum = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}minimum") map { _.as[String]}
lazy val spiritmaximum = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}maximum") map { _.as[String]}
lazy val spiritrangeType = attributes("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}rangeType").as[RangeTypeType]
lazy val spiritprompt = attributes("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}prompt").as[String]
}
case class AddressSpaceRef(baseAddress: Option[BaseAddress] = None,
attributes: Map[String, IPXACT2009scalaxb.DataRecord[Any]] = Map.empty) extends AddrSpaceRefTypable {
lazy val spiritaddressSpaceRef = attributes("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}addressSpaceRef").as[String]
}
case class Master(addressSpaceRef: Option[AddressSpaceRef] = None) extends InterfaceModeOption
case class Bridge(attributes: Map[String, IPXACT2009scalaxb.DataRecord[Any]] = Map.empty) {
lazy val masterRef = attributes("@masterRef").as[String]
lazy val opaque = attributes("@opaque").as[Boolean]
}
case class FileSetRefGroup2(group: Option[String] = None,
fileSetRef: Seq[FileSetRef] = Nil)
case class Slave(memoryMapRef: Option[MemoryMapRefType] = None,
bridge: Seq[Bridge] = Nil,
fileSetRefGroup: Seq[FileSetRefGroup2] = Nil) extends InterfaceModeOption
case class System(group: String) extends InterfaceModeOption
case class RemapAddress(value: String,
attributes: Map[String, IPXACT2009scalaxb.DataRecord[Any]] = Map.empty) {
lazy val spiritformat = attributes("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}format").as[FormatType]
lazy val spiritresolve = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}resolve") map { _.as[ResolveType]}
lazy val spiritid = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}id") map { _.as[String]}
lazy val spiritdependency = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}dependency") map { _.as[String]}
lazy val spiritchoiceRef = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}choiceRef") map { _.as[String]}
lazy val spiritorder = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}order") map { _.as[Float]}
lazy val spiritconfigGroups = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}configGroups") map { _.as[Seq[String]]}
lazy val spiritbitStringLength = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}bitStringLength") map { _.as[BigInt]}
lazy val spiritminimum = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}minimum") map { _.as[String]}
lazy val spiritmaximum = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}maximum") map { _.as[String]}
lazy val spiritrangeType = attributes("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}rangeType").as[RangeTypeType]
lazy val spiritprompt = attributes("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}prompt").as[String]
lazy val state = attributes.get("@state") map { _.as[String]}
}
case class RangeType(value: String,
attributes: Map[String, IPXACT2009scalaxb.DataRecord[Any]] = Map.empty) {
lazy val spiritformat = attributes("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}format").as[FormatType]
lazy val spiritresolve = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}resolve") map { _.as[ResolveType]}
lazy val spiritid = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}id") map { _.as[String]}
lazy val spiritdependency = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}dependency") map { _.as[String]}
lazy val spiritchoiceRef = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}choiceRef") map { _.as[String]}
lazy val spiritorder = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}order") map { _.as[Float]}
lazy val spiritconfigGroups = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}configGroups") map { _.as[Seq[String]]}
lazy val spiritbitStringLength = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}bitStringLength") map { _.as[BigInt]}
lazy val spiritminimum = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}minimum") map { _.as[String]}
lazy val spiritmaximum = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}maximum") map { _.as[String]}
lazy val spiritrangeType = attributes("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}rangeType").as[RangeTypeType]
lazy val spiritprompt = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}prompt") map { _.as[String]}
}
case class BaseAddresses(remapAddress: Seq[RemapAddress] = Nil,
range: RangeType)
case class MirroredSlave(mirroredslavesequence1: Option[MirroredSlaveSequence1] = None) extends InterfaceModeOption
case class MirroredSlaveSequence1(baseAddresses: Option[BaseAddresses] = None)
case class MirroredMaster() extends InterfaceModeOption
case class MirroredSystem(group: String) extends InterfaceModeOption
sealed trait InterfaceMode
object InterfaceMode {
def fromString(value: String, scope: scala.xml.NamespaceBinding)(implicit fmt: IPXACT2009scalaxb.XMLFormat[InterfaceMode]): InterfaceMode = fmt.reads(scala.xml.Text(value), Nil) match {
case Right(x: InterfaceMode) => x
case x => throw new RuntimeException(s"fromString returned unexpected value $x for input $value")
}
lazy val values: Seq[InterfaceMode] = Seq(MasterValue2, SlaveValue2, SystemValue2, MirroredMasterValue, MirroredSlaveValue, MirroredSystemValue)
}
case object MasterValue2 extends InterfaceMode { override def toString = "master" }
case object SlaveValue2 extends InterfaceMode { override def toString = "slave" }
case object SystemValue2 extends InterfaceMode { override def toString = "system" }
case object MirroredMasterValue extends InterfaceMode { override def toString = "mirroredMaster" }
case object MirroredSlaveValue extends InterfaceMode { override def toString = "mirroredSlave" }
case object MirroredSystemValue extends InterfaceMode { override def toString = "mirroredSystem" }
case class Monitor(group: Option[String] = None,
attributes: Map[String, IPXACT2009scalaxb.DataRecord[Any]] = Map.empty) extends InterfaceModeOption {
lazy val interfaceMode = attributes("@interfaceMode").as[InterfaceMode]
}
case class Master2() extends AbstractorInterfaceModeOption
case class Slave2() extends AbstractorInterfaceModeOption
case class System2(group: String) extends AbstractorInterfaceModeOption
case class MirroredSlave2() extends AbstractorInterfaceModeOption
case class MirroredMaster2() extends AbstractorInterfaceModeOption
case class MirroredSystem2(group: String) extends AbstractorInterfaceModeOption
case class LeftType2(value: BigInt,
attributes: Map[String, IPXACT2009scalaxb.DataRecord[Any]] = Map.empty) {
lazy val spiritformat = attributes("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}format").as[FormatType]
lazy val spiritresolve = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}resolve") map { _.as[ResolveType]}
lazy val spiritid = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}id") map { _.as[String]}
lazy val spiritdependency = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}dependency") map { _.as[String]}
lazy val spiritchoiceRef = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}choiceRef") map { _.as[String]}
lazy val spiritorder = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}order") map { _.as[Float]}
lazy val spiritconfigGroups = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}configGroups") map { _.as[Seq[String]]}
lazy val spiritbitStringLength = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}bitStringLength") map { _.as[BigInt]}
lazy val spiritminimum = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}minimum") map { _.as[String]}
lazy val spiritmaximum = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}maximum") map { _.as[String]}
lazy val spiritrangeType = attributes("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}rangeType").as[RangeTypeType]
lazy val spiritprompt = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}prompt") map { _.as[String]}
}
case class RightType2(value: BigInt,
attributes: Map[String, IPXACT2009scalaxb.DataRecord[Any]] = Map.empty) {
lazy val spiritformat = attributes("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}format").as[FormatType]
lazy val spiritresolve = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}resolve") map { _.as[ResolveType]}
lazy val spiritid = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}id") map { _.as[String]}
lazy val spiritdependency = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}dependency") map { _.as[String]}
lazy val spiritchoiceRef = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}choiceRef") map { _.as[String]}
lazy val spiritorder = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}order") map { _.as[Float]}
lazy val spiritconfigGroups = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}configGroups") map { _.as[Seq[String]]}
lazy val spiritbitStringLength = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}bitStringLength") map { _.as[BigInt]}
lazy val spiritminimum = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}minimum") map { _.as[String]}
lazy val spiritmaximum = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}maximum") map { _.as[String]}
lazy val spiritrangeType = attributes("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}rangeType").as[RangeTypeType]
lazy val spiritprompt = attributes.get("@{http://www.spiritconsortium.org/XMLSchema/SPIRIT/1685-2009}prompt") map { _.as[String]}
}
case class Vector2(left: LeftType2,
right: RightType2)
case class LogicalPort2(name: String,
vector: Option[Vector2] = None)
case class PhysicalPort2(name: String,
vector: Option[Vector4] = None)
case class PortMap2(logicalPort: LogicalPort2,
physicalPort: PhysicalPort2)
case class PortMaps2(portMap: Seq[PortMap2] = Nil)
/** Type definition for a busInterface in a component
*/
case class AbstractorBusInterfaceType(nameGroupSequence1: NameGroupSequence,
abstractionType: LibraryRefType,
portMaps: Option[PortMaps2] = None,
parameters: Option[Parameters] = None,
vendorExtensions: Option[VendorExtensions] = None,
attributes: Map[String, IPXACT2009scalaxb.DataRecord[Any]] = Map.empty)
trait AbstractorInterfaceModeOption
trait InterfaceModeOption
© 2015 - 2025 Weber Informatics LLC | Privacy Policy