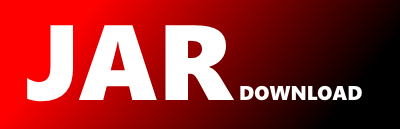
IPXACT2022ScalaCases.subInstances.scala Maven / Gradle / Ivy
// Generated by IPXACT2022scalaxb.
package IPXACT2022ScalaCases
/** An unsigned bit vector expression that resolves to the value set {0, 1, ...} or or the string values 'open' or 'default'. It is derived from simpleUnsignedBitVectorExpression and it supports an expression value.
*/
case class ComplexTiedValueExpression(value: String,
attributes: Map[String, IPXACT2022scalaxb.DataRecord[Any]] = Map.empty) {
lazy val minimum = attributes.get("@minimum") map { _.as[Int]}
lazy val maximum = attributes.get("@maximum") map { _.as[Int]}
}
case class ConfigurableElementValues(configurableelementvaluessequence1: Seq[IPXACT2022ScalaCases.ConfigurableElementValuesSequence1] = Nil)
case class ConfigurableElementValuesSequence1(configurableElementValue: IPXACT2022ScalaCases.ConfigurableElementValue)
case class ConfigurableElementValue(value: String,
attributes: Map[String, IPXACT2022scalaxb.DataRecord[Any]] = Map.empty) extends ComplexBaseExpressionable {
lazy val referenceId = attributes("@referenceId").as[String]
lazy val xmlid = attributes.get("@{http://www.w3.org/XML/1998/namespace}id") map { _.as[String]}
}
case class ComponentInstance(instanceName: String,
displayName: Option[String] = None,
shortDescription: Option[String] = None,
description: Option[String] = None,
componentRef: IPXACT2022ScalaCases.ConfigurableLibraryRefType,
powerDomainLinks: Option[IPXACT2022ScalaCases.PowerDomainLinks] = None,
vendorExtensions: Option[IPXACT2022ScalaCases.VendorExtensions] = None,
attributes: Map[String, IPXACT2022scalaxb.DataRecord[Any]] = Map.empty) {
lazy val xmlid = attributes.get("@{http://www.w3.org/XML/1998/namespace}id") map { _.as[String]}
}
case class ComponentInstances(componentInstance: Seq[IPXACT2022ScalaCases.ComponentInstance] = Nil)
case class InternalPortReference(subPortReference: Seq[IPXACT2022ScalaCases.SubPortReference] = Nil,
partSelect: Option[IPXACT2022ScalaCases.PartSelect] = None,
vendorExtensions: Option[IPXACT2022ScalaCases.VendorExtensions] = None,
attributes: Map[String, IPXACT2022scalaxb.DataRecord[Any]] = Map.empty) {
lazy val portRef = attributes("@portRef").as[String]
lazy val componentInstanceRef = attributes("@componentInstanceRef").as[String]
lazy val xmlid = attributes.get("@{http://www.w3.org/XML/1998/namespace}id") map { _.as[String]}
}
case class PortReferences(portreferencesoption: Seq[IPXACT2022scalaxb.DataRecord[IPXACT2022ScalaCases.PortReferencesOption]] = Nil)
trait PortReferencesOption
case class PortReferencesSequence1(internalPortReference: Seq[IPXACT2022ScalaCases.InternalPortReference] = Nil,
externalPortReference: Seq[IPXACT2022ScalaCases.ExternalPortReference] = Nil) extends PortReferencesOption
case class AdHocConnection(nameGroupPortSequence1: IPXACT2022ScalaCases.NameGroupPortSequence,
tiedValue: Option[IPXACT2022ScalaCases.ComplexTiedValueExpression] = None,
portReferences: IPXACT2022ScalaCases.PortReferences,
vendorExtensions: Option[IPXACT2022ScalaCases.VendorExtensions] = None,
attributes: Map[String, IPXACT2022scalaxb.DataRecord[Any]] = Map.empty) {
lazy val xmlid = attributes.get("@{http://www.w3.org/XML/1998/namespace}id") map { _.as[String]}
}
case class AdHocConnections(adHocConnection: Seq[IPXACT2022ScalaCases.AdHocConnection] = Nil)
case class Interconnection(nameGroupSequence1: IPXACT2022ScalaCases.NameGroupSequence,
activeInterface: IPXACT2022ScalaCases.ActiveInterface,
interconnectionoption: Seq[IPXACT2022scalaxb.DataRecord[IPXACT2022ScalaCases.InterconnectionOption]] = Nil,
vendorExtensions: Option[IPXACT2022ScalaCases.VendorExtensions] = None,
attributes: Map[String, IPXACT2022scalaxb.DataRecord[Any]] = Map.empty) extends InterconnectionsOption {
lazy val xmlid = attributes.get("@{http://www.w3.org/XML/1998/namespace}id") map { _.as[String]}
}
trait InterconnectionOption
case class InterconnectionSequence1(activeInterface: Seq[IPXACT2022ScalaCases.ActiveInterface] = Nil,
hierInterface: Seq[IPXACT2022ScalaCases.HierInterfaceType] = Nil) extends InterconnectionOption
case class MonitorInterconnection(nameGroupSequence1: IPXACT2022ScalaCases.NameGroupSequence,
monitoredActiveInterface: IPXACT2022ScalaCases.MonitorInterfaceType,
monitorInterface: Seq[IPXACT2022ScalaCases.MonitorInterfaceType] = Nil,
vendorExtensions: Option[IPXACT2022ScalaCases.VendorExtensions] = None,
attributes: Map[String, IPXACT2022scalaxb.DataRecord[Any]] = Map.empty) extends InterconnectionsOption {
lazy val xmlid = attributes.get("@{http://www.w3.org/XML/1998/namespace}id") map { _.as[String]}
}
case class Interconnections(interconnectionsoption: Seq[IPXACT2022scalaxb.DataRecord[IPXACT2022ScalaCases.InterconnectionsOption]] = Nil)
trait InterconnectionsOption
/** A representation of a component/bus interface relation; i.e. a bus interface belonging to a certain component.
*/
trait InterfaceTypable {
def componentInstanceRef: String
def busRef: String
def xmlid: Option[String]
}
/** A representation of a component/bus interface relation; i.e. a bus interface belonging to a certain component.
*/
case class InterfaceType(attributes: Map[String, IPXACT2022scalaxb.DataRecord[Any]] = Map.empty) extends InterfaceTypable {
lazy val componentInstanceRef = attributes("@componentInstanceRef").as[String]
lazy val busRef = attributes("@busRef").as[String]
lazy val xmlid = attributes.get("@{http://www.w3.org/XML/1998/namespace}id") map { _.as[String]}
}
/** A representation of an exported interface. The busRef indicates the name of the interface in the containing component.
*/
case class HierInterfaceType(description: Option[String] = None,
vendorExtensions: Option[IPXACT2022ScalaCases.VendorExtensions] = None,
attributes: Map[String, IPXACT2022scalaxb.DataRecord[Any]] = Map.empty) extends InterconnectionOption {
lazy val busRef = attributes("@busRef").as[String]
lazy val xmlid = attributes.get("@{http://www.w3.org/XML/1998/namespace}id") map { _.as[String]}
}
/** Hierarchical reference to an interface being monitored or monitoring another interface.
*/
case class MonitorInterfaceType(description: Option[String] = None,
vendorExtensions: Option[IPXACT2022ScalaCases.VendorExtensions] = None,
attributes: Map[String, IPXACT2022scalaxb.DataRecord[Any]] = Map.empty) extends InterfaceTypable {
lazy val componentInstanceRef = attributes("@componentInstanceRef").as[String]
lazy val busRef = attributes("@busRef").as[String]
lazy val xmlid = attributes.get("@{http://www.w3.org/XML/1998/namespace}id") map { _.as[String]}
lazy val path = attributes.get("@path") map { _.as[String]}
}
case class ExternalPortReference(subPortReference: Seq[IPXACT2022ScalaCases.SubPortReference] = Nil,
partSelect: Option[IPXACT2022ScalaCases.PartSelect] = None,
vendorExtensions: Option[IPXACT2022ScalaCases.VendorExtensions] = None,
attributes: Map[String, IPXACT2022scalaxb.DataRecord[Any]] = Map.empty) extends PortReferencesOption {
lazy val portRef = attributes("@portRef").as[String]
lazy val xmlid = attributes.get("@{http://www.w3.org/XML/1998/namespace}id") map { _.as[String]}
}
case class ExcludePort(value: String,
attributes: Map[String, IPXACT2022scalaxb.DataRecord[Any]] = Map.empty) {
lazy val xmlid = attributes.get("@{http://www.w3.org/XML/1998/namespace}id") map { _.as[String]}
}
case class ExcludePorts(excludePort: Seq[IPXACT2022ScalaCases.ExcludePort] = Nil)
case class ActiveInterface(description: Option[String] = None,
excludePorts: Option[IPXACT2022ScalaCases.ExcludePorts] = None,
vendorExtensions: Option[IPXACT2022ScalaCases.VendorExtensions] = None,
attributes: Map[String, IPXACT2022scalaxb.DataRecord[Any]] = Map.empty) extends InterfaceTypable {
lazy val componentInstanceRef = attributes("@componentInstanceRef").as[String]
lazy val busRef = attributes("@busRef").as[String]
lazy val xmlid = attributes.get("@{http://www.w3.org/XML/1998/namespace}id") map { _.as[String]}
}
case class InternalPowerDomainReference(value: String,
attributes: Map[String, IPXACT2022scalaxb.DataRecord[Any]] = Map.empty) {
lazy val xmlid = attributes.get("@{http://www.w3.org/XML/1998/namespace}id") map { _.as[String]}
}
case class PowerDomainLink(externalPowerDomainReference: IPXACT2022ScalaCases.StringExpressionable,
internalPowerDomainReference: Seq[IPXACT2022ScalaCases.InternalPowerDomainReference] = Nil,
attributes: Map[String, IPXACT2022scalaxb.DataRecord[Any]] = Map.empty) {
lazy val xmlid = attributes.get("@{http://www.w3.org/XML/1998/namespace}id") map { _.as[String]}
}
case class PowerDomainLinks(powerDomainLink: Seq[IPXACT2022ScalaCases.PowerDomainLink] = Nil)
© 2015 - 2025 Weber Informatics LLC | Privacy Policy