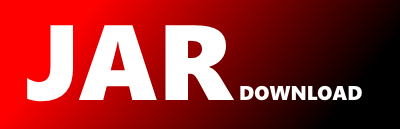
com.github.spjoe.getter.GetterResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of annotation-cache Show documentation
Show all versions of annotation-cache Show documentation
A library which provides caching for annotation on getters of java bean properties.
It also creates byte code (via reflectAsm) to call getter and setter of the annotated java bean property
/*
Copyright 2016 Camillo Dell'mour
Licensed under the Apache License,Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
*/
package com.github.spjoe.getter;
import java.util.function.Supplier;
public final class GetterResult {
private static final GetterResult NO_GETTER_RESULT = new GetterResult(null, false, false);
private static final GetterResult GETTER_RESULT_FAILED = new GetterResult(null, true, false);
private final T result;
private final boolean getterExist;
private boolean success;
private GetterResult(final T result, final boolean getterExist, final boolean success) {
this.result = result;
this.getterExist = getterExist;
this.success = success;
}
public boolean doesGetterExist() {
return getterExist;
}
public boolean isSuccess() {
return success;
}
public T orElse(final T defaultValue) {
return orElseGet(() -> defaultValue);
}
public T orElseGet(final Supplier defaultSupplier) {
return getterExist && success ? result : defaultSupplier.get();
}
public T orElseThrow(final Supplier throwableSupplier) throws X {
if (getterExist && success) {
return result;
} else {
throw throwableSupplier.get();
}
}
public static GetterResult noGetter() {
return NO_GETTER_RESULT;
}
public static GetterResult failed() {
return GETTER_RESULT_FAILED;
}
public static GetterResult success(final T result) {
return new GetterResult<>(result, true, true);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy