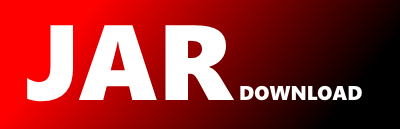
com.github.spoptchev.kotlin.preconditions.Precondition.kt Maven / Gradle / Ivy
package com.github.spoptchev.kotlin.preconditions
data class Result(val valid: Boolean, val lazyMessage: () -> String)
data class Condition(
val value: T,
private val label: String,
private val negated: Boolean = false
) {
val expectedTo: String by lazy {
when (negated) {
true -> "expected $label $value not to"
else -> "expected $label $value to"
}
}
fun test(run: Condition.() -> Result): Result = run(this)
fun withResult(valid: Boolean, lazyMessage: () -> String) = Result(valid == !negated, lazyMessage)
}
data class Assertion(
private val value: T,
private val label: String,
private val evaluate: EvaluationMethod
) {
fun run(precondition: Precondition): T = evalPrecondition(precondition)
.let { evaluate(it.valid, it.lazyMessage) }
.let { value }
private fun evalPrecondition(precondition: Precondition, negated: Boolean = false): Result = when(precondition) {
is Matcher ->
precondition.test(Condition(value, label, negated))
is AndPrecondition -> {
val left = evalPrecondition(precondition.left)
if (left.valid) evalPrecondition(precondition.right) else left
}
is OrPrecondition -> {
val left = evalPrecondition(precondition.left)
if (left.valid) left else evalPrecondition(precondition.right)
}
is NotPrecondition ->
evalPrecondition(precondition.precondition, true)
}
}
sealed class Precondition
abstract class Matcher : Precondition() {
abstract fun test(condition: Condition): Result
}
class AndPrecondition(val left: Precondition, val right: Precondition) : Precondition()
class OrPrecondition(val left: Precondition, val right: Precondition) : Precondition()
class NotPrecondition(val precondition: Precondition) : Precondition()
© 2015 - 2024 Weber Informatics LLC | Privacy Policy