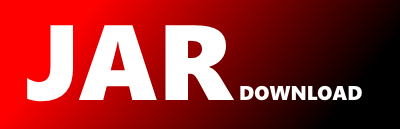
edu.umd.cs.findbugs.detect.ThrowingExceptions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of spotbugs Show documentation
Show all versions of spotbugs Show documentation
SpotBugs: Because it's easy!
package edu.umd.cs.findbugs.detect;
import edu.umd.cs.findbugs.BugInstance;
import edu.umd.cs.findbugs.BugReporter;
import edu.umd.cs.findbugs.OpcodeStack;
import edu.umd.cs.findbugs.annotations.NonNull;
import edu.umd.cs.findbugs.ba.AnalysisContext;
import edu.umd.cs.findbugs.ba.SignatureParser;
import edu.umd.cs.findbugs.ba.XMethod;
import edu.umd.cs.findbugs.bcel.OpcodeStackDetector;
import edu.umd.cs.findbugs.internalAnnotations.DottedClassName;
import java.util.Arrays;
import java.util.Optional;
import java.util.stream.Stream;
import edu.umd.cs.findbugs.util.ClassName;
import edu.umd.cs.findbugs.util.Values;
import org.apache.bcel.Const;
import org.apache.bcel.classfile.Code;
import org.apache.bcel.classfile.ExceptionTable;
import org.apache.bcel.classfile.JavaClass;
import org.apache.bcel.classfile.Method;
import org.apache.commons.lang3.StringUtils;
public class ThrowingExceptions extends OpcodeStackDetector {
private final BugReporter bugReporter;
public ThrowingExceptions(BugReporter bugReporter) {
this.bugReporter = bugReporter;
}
private String exceptionThrown = null;
@Override
public void visit(Method obj) {
exceptionThrown = null;
if (obj.isSynthetic()) {
return;
}
// If the method is generic or is a method of a generic class, then first check the generic signature to avoid detection
// of generic descendants of Exception or Throwable as Exception or Throwable itself.
Stream exceptionStream = null;
String signature = obj.getGenericSignature();
if (signature != null) {
String[] exceptions = StringUtils.substringsBetween(signature, "^", ";");
if (exceptions != null) {
exceptionStream = Arrays.stream(exceptions)
.filter(s -> s.charAt(0) == 'L')
.map(s -> ClassName.toDottedClassName(s.substring(1)));
}
}
// If the method is not generic, or it does not throw a generic exception then it has no exception specification in its generic signature.
if (signature == null || exceptionStream == null) {
ExceptionTable exceptionTable = obj.getExceptionTable();
if (exceptionTable != null) {
exceptionStream = Arrays.stream(exceptionTable.getExceptionNames());
}
}
// If the method throws Throwable or Exception because its ancestor throws the same exception then ignore it.
if (exceptionStream != null) {
Optional exception = exceptionStream
.filter(s -> Values.DOTTED_JAVA_LANG_EXCEPTION.equals(s) || Values.DOTTED_JAVA_LANG_THROWABLE.equals(s))
.findAny();
if (exception.isPresent() && !parentThrows(getThisClass(), obj, exception.get())) {
exceptionThrown = exception.get();
}
}
// If the method's body is empty then we report the bug immediately.
if (obj.getCode() == null && exceptionThrown != null) {
reportBug(Values.DOTTED_JAVA_LANG_EXCEPTION.equals(exceptionThrown) ? "THROWS_METHOD_THROWS_CLAUSE_BASIC_EXCEPTION"
: "THROWS_METHOD_THROWS_CLAUSE_THROWABLE", getXMethod());
}
}
@Override
public void visitAfter(Code obj) {
// For methods with bodies we report the exception after checking their body.
if (exceptionThrown != null) {
reportBug(Values.DOTTED_JAVA_LANG_EXCEPTION.equals(exceptionThrown) ? "THROWS_METHOD_THROWS_CLAUSE_BASIC_EXCEPTION"
: "THROWS_METHOD_THROWS_CLAUSE_THROWABLE", getXMethod());
}
}
@Override
public void sawOpcode(int seen) {
if (seen == Const.ATHROW) {
OpcodeStack.Item item = stack.getStackItem(0);
if (item != null && "Ljava/lang/RuntimeException;".equals(item.getSignature())) {
bugReporter.reportBug(new BugInstance(this, "THROWS_METHOD_THROWS_RUNTIMEEXCEPTION", LOW_PRIORITY)
.addClass(this)
.addMethod(getXMethod())
.addSourceLine(this));
}
} else if (exceptionThrown != null
&& (seen == Const.INVOKEVIRTUAL || seen == Const.INVOKEINTERFACE || seen == Const.INVOKESTATIC)) {
// If the method throws Throwable or Exception because it invokes another method throwing such
// exceptions then ignore this bug by resetting exceptionThrown to null.
XMethod calledMethod = getXMethodOperand();
if (calledMethod == null) {
return;
}
String[] thrownExceptions = calledMethod.getThrownExceptions();
if (thrownExceptions != null && Arrays.stream(thrownExceptions)
.map(ClassName::toDottedClassName)
.anyMatch(exceptionThrown::equals)) {
exceptionThrown = null;
}
}
}
private void reportBug(String bugName, XMethod method) {
bugReporter.reportBug(new BugInstance(this, bugName, LOW_PRIORITY)
.addClass(this)
.addMethod(method));
}
private boolean parentThrows(@NonNull JavaClass clazz, @NonNull Method method, @DottedClassName String exception) {
boolean throwsEx = false;
try {
JavaClass ancestor = clazz.getSuperClass();
if (ancestor != null) {
Optional superMethod = Arrays.stream(ancestor.getMethods())
.filter(m -> method.getName().equals(m.getName()) && signatureMatches(method, m))
.findAny();
if (superMethod.isPresent()) {
throwsEx = Arrays.asList(superMethod.get().getExceptionTable().getExceptionNames()).contains(exception);
} else {
throwsEx = parentThrows(ancestor, method, exception);
}
}
for (JavaClass intf : clazz.getInterfaces()) {
Optional superMethod = Arrays.stream(intf.getMethods())
.filter(m -> method.getName().equals(m.getName()) && signatureMatches(method, m))
.findAny();
if (superMethod.isPresent()) {
throwsEx |= Arrays.asList(superMethod.get().getExceptionTable().getExceptionNames()).contains(exception);
} else {
throwsEx |= parentThrows(intf, method, exception);
}
}
} catch (ClassNotFoundException e) {
AnalysisContext.reportMissingClass(e);
}
return throwsEx;
}
private boolean signatureMatches(Method child, Method parent) {
String genSig = parent.getGenericSignature();
if (genSig == null) {
return child.getSignature().equals(parent.getSignature());
}
String sig = child.getSignature();
SignatureParser genSP = new SignatureParser(genSig);
SignatureParser sp = new SignatureParser(sig);
if (genSP.getNumParameters() != sp.getNumParameters()) {
return false;
}
String[] gArgs = genSP.getArguments();
String[] args = sp.getArguments();
for (int i = 0; i < sp.getNumParameters(); ++i) {
if (gArgs[i].charAt(0) == 'T') {
if (args[i].charAt(0) != 'L') {
return false;
}
} else {
if (!gArgs[i].equals(args[i])) {
return false;
}
}
}
String gRet = genSP.getReturnTypeSignature();
String ret = sp.getReturnTypeSignature();
return (gRet.charAt(0) == 'T' && ret.charAt(0) == 'L') || gRet.equals(ret);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy