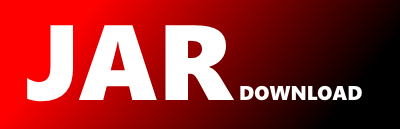
org.myframework.codeutil.Column Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of coder-maven-plugin Show documentation
Show all versions of coder-maven-plugin Show documentation
Assist in generating CRUD code, without limitation on the specific language, as long as you can write velocity templates.
package org.myframework.codeutil;
import org.myframework.util.StringUtil;
public class Column {
private String javaName;
private String columnName;
private String dataType ;
private String jdbcType ;
private String nullable ="false";
private String length="10";
private String precision="10";
private String scale = "0";
private String comments="";
//是否主键字段
private String columnKey="";
private String unique;
public String getComments() {
return comments;
}
public void setComments(String comments) {
this.comments = comments;
}
public boolean isColumnKey() {
return "TRUE".equalsIgnoreCase(columnKey);
}
public void setColumnKey(String columnKey) {
this.columnKey = columnKey;
}
public String getColumnName() {
return columnName;
}
public String getJdbcType() {
return jdbcType;
}
public void setJdbcType(String jdbcType) {
this.jdbcType = jdbcType;
}
public String getSetterMethod() {
return "set"+StringUtil.firstCharUpperCase(javaName) ;
}
public String getGetterMethod() {
return "get"+StringUtil.firstCharUpperCase(javaName) ;
}
public void setColumnName(String columnName) {
this.columnName = columnName;
this.javaName = StringUtil.toBeanPatternStr(columnName);
}
public String getUnique() {
return unique;
}
public void setUnique(String unique) {
this.unique = unique;
}
public String getNullable() {
return nullable;
}
public void setNullable(String nullable) {
this.nullable = nullable;
}
public String getLength() {
return length;
}
public void setLength(String length) {
this.length = length;
}
public String getPrecision() {
return precision;
}
public void setPrecision(String precision) {
this.precision = precision;
}
public String getScale() {
return scale;
}
public void setScale(String scale) {
this.scale = scale;
}
public String getJavaName() {
return javaName;
}
public void setJavaName(String javaName) {
this.javaName = javaName;
}
public String getDataType() {
return dataType;
}
public void setDataType(String dataType) {
this.dataType = dataType;
}
@Override
public String toString() {
return "Column [javaName=" + javaName + ", columnName=" + columnName
+ ", dataType=" + dataType+ ", jdbcType=" + jdbcType+ ", unique=" + unique
+ ", nullable=" + nullable + ", length=" + length
+ ", precision=" + precision + ", scale=" + scale
+ ", comments=" + comments + ", columnKey=" + columnKey + "]";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy