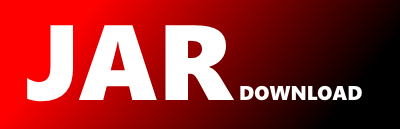
com.github.springbootPlus.excel.ExcelContext Maven / Gradle / Ivy
package com.github.springbootPlus.excel;
import com.github.springbootPlus.excel.config.ExcelDefinition;
import com.github.springbootPlus.excel.config.FieldValue;
import com.github.springbootPlus.excel.exception.ExcelException;
import com.github.springbootPlus.excel.parsing.ExcelContextHolder;
import com.github.springbootPlus.excel.parsing.ExcelExport;
import com.github.springbootPlus.excel.parsing.ExcelHeader;
import com.github.springbootPlus.excel.parsing.ExcelImport;
import com.github.springbootPlus.excel.result.ExcelExportResult;
import com.github.springbootPlus.excel.result.ExcelImportResult;
import com.github.springbootPlus.excel.util.ReflectUtils;
import com.github.springbootPlus.excel.util.StringUtils;
import com.github.springbootPlus.excel.xml.XMLExcelDefinitionReader;
import org.apache.poi.ss.usermodel.Workbook;
import java.io.File;
import java.io.FileOutputStream;
import java.io.InputStream;
import java.io.OutputStream;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
/**
* Excel上下文支持,只需指定location配置文件路径,即可使用
* ExcelContext context = new ExcelContext("excel/material-config.xml");
* context.createExcel( id,beans);
*
* @author lisuo
*/
public class ExcelContext {
private ExcelDefinitionReader definitionReader;
/**
* 用于缓存Excel配置
*/
private Map> fieldValueMap = new HashMap>();
/**
* 导出
*/
private ExcelExport excelExport;
/**
* 导入
*/
private ExcelImport excelImport;
/**
* @param location 配置文件类路径
*/
public ExcelContext(String location) {
try {
//这里默认使用XML ExcelContent,如果有自己的需求需要自行修改
definitionReader = new XMLExcelDefinitionReader(location);
excelExport = new ExcelExport(definitionReader);
excelImport = new ExcelImport(definitionReader);
} catch (Exception e) {
throw new RuntimeException(e);
}
}
/**
* 创建Excel
*
* @param id 配置ID
* @param beans 配置class对应的List
* @return Workbook
* @throws Exception
*/
public Workbook createExcel(String id, List> beans) throws Exception {
return createExcel(id, beans, null, null);
}
/**
* 创建Excel
*
* @param id 配置ID
* @param beans 配置class对应的List
* @return File
* @throws Exception
*/
public File createExcelFile(String id, List> beans) throws Exception {
File tmpFile = File.createTempFile(StringUtils.getUUId(), ".xlsx");
OutputStream ops = new FileOutputStream(tmpFile);
Workbook workbook = createExcel(id, beans);
workbook.write(ops);
ops.close();
workbook.close();
return tmpFile;
}
/**
* 创建Excel部分信息
*
* @param id 配置ID
* @param beans 配置class对应的List
* @return Workbook
* @throws Exception
*/
public ExcelExportResult createExcelForPart(String id, List> beans) throws Exception {
return createExcelForPart(id, beans, null, null);
}
/**
* 创建Excel
*
* @param id 配置ID
* @param beans 配置class对应的List
* @param header 导出之前,在标题前面做出一些额外的操作,比如增加文档描述等,可以为null
* @return Workbook
* @throws Exception
*/
public Workbook createExcel(String id, List> beans, ExcelHeader header) throws Exception {
return createExcel(id, beans, header, null);
}
/**
* 创建Excel部分信息
*
* @param id 配置ID
* @param beans 配置class对应的List
* @param header 导出之前,在标题前面做出一些额外的操作,比如增加文档描述等,可以为null
* @return Workbook
* @throws Exception
*/
public ExcelExportResult createExcelForPart(String id, List> beans, ExcelHeader header) throws Exception {
return createExcelForPart(id, beans, header, null);
}
/**
* 创建Excel
*
* @param id 配置ID
* @param beans 配置class对应的List
* @param header 导出之前,在标题前面做出一些额外的操作,比如增加文档描述等,可以为null
* @param fields 指定Excel导出的字段(bean对应的字段名称),可以为null
* @return Workbook
* @throws Exception
*/
public Workbook createExcel(String id, List> beans, ExcelHeader header, List fields) throws Exception {
return excelExport.createExcel(id, beans, header, fields).build();
}
/**
* 创建Excel部分信息
*
* @param id 配置ID
* @param beans 配置class对应的List
* @param header 导出之前,在标题前面做出一些额外的操作,比如增加文档描述等,可以为null
* @param fields 指定Excel导出的字段(bean对应的字段名称),可以为null
* @return Workbook
* @throws Exception
*/
public ExcelExportResult createExcelForPart(String id, List> beans, ExcelHeader header, List fields) throws Exception {
return excelExport.createExcel(id, beans, header, fields);
}
/**
* 创建Excel,模板信息
*
* @param id ExcelXML配置Bean的ID
* @param header Excel头信息(在标题之前)
* @param fields 指定导出的字段
* @return
* @throws Exception
*/
public Workbook createExcelTemplate(String id, ExcelHeader header, List fields) throws Exception {
return excelExport.createExcelTemplate(id, header, fields);
}
/***
* 读取Excel信息
* @param id 配置ID
* @param excelStream Excel文件流
* @return ExcelImportResult
* @throws Exception
*/
public ExcelImportResult readExcel(String id, InputStream excelStream) throws Exception {
return excelImport.readExcel(id, excelStream, null, false);
}
/***
* 读取Excel信息
* @param id 配置ID
* @param excelStream Excel文件流
* @return ExcelImportResult
* @throws Exception
*/
public ExcelImportResult readExcel(String id, InputStream excelStream, Map context) throws Exception {
ExcelContextHolder.setContext(context);
return excelImport.readExcel(id, excelStream, null, true);
}
/***
* 读取Excel信息
* @param id 配置ID
* @param excelStream Excel文件流
* @param multivalidate 是否逐条校验,默认单行出错立即抛出ExcelException,为true时为批量校验,可通过ExcelImportResult.hasErrors,和getErrors获取具体错误信息
* @return ExcelImportResult
* @throws Exception
*/
public ExcelImportResult readExcel(String id, InputStream excelStream, boolean multivalidate) throws Exception {
return excelImport.readExcel(id, excelStream, null, multivalidate);
}
/***
* 读取Excel信息
* @param id 配置ID
* @param excelStream Excel文件流
* @param sheetIndex Sheet索引位
* @return ExcelImportResult
* @throws Exception
*/
public ExcelImportResult readExcel(String id, InputStream excelStream, int sheetIndex) throws Exception {
return excelImport.readExcel(id, excelStream, sheetIndex, false);
}
/***
* 读取Excel信息
* @param id 配置ID
* @param excelStream Excel文件流
* @param sheetIndex Sheet索引位
* @param multivalidate 是否逐条校验,默认单行出错立即抛出ExcelException,为true时为批量校验,可通过ExcelImportResult.hasErrors,和getErrors获取具体错误信息
* @return ExcelImportResult
* @throws Exception
*/
public ExcelImportResult readExcel(String id, InputStream excelStream, int sheetIndex, boolean multivalidate) throws Exception {
return excelImport.readExcel(id, excelStream, sheetIndex, multivalidate);
}
/**
* 获取Excel 配置文件中的字段
*
* @param key
* @return
*/
public List getFieldValues(String key) {
List list = fieldValueMap.get(key);
if (list == null) {
ExcelDefinition def = definitionReader.getRegistry().get(key);
if (def == null) {
throw new ExcelException("没有找到[" + key + "]的配置信息");
}
//使用copy方式,避免使用者修改原生的配置信息
List fieldValues = def.getFieldValues();
list = new ArrayList(fieldValues.size());
for (FieldValue fieldValue : fieldValues) {
FieldValue val = new FieldValue();
ReflectUtils.copyProps(fieldValue, val);
list.add(val);
}
fieldValueMap.put(key, list);
}
return list;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy